mirror of
https://github.com/hay-kot/homebox.git
synced 2024-11-16 21:58:40 +00:00
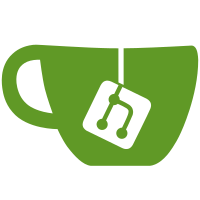
* add user profiles and theme selectors * lowercase buttons by default * basic layout * (wip) init token APIs * refactor server to support variable options * fix types * api refactor / registration tests * implement UI for url and join * remove console.logs * rename repository factory * fix upload size
60 lines
1.2 KiB
TypeScript
60 lines
1.2 KiB
TypeScript
import { Ref } from "vue";
|
|
|
|
export type DaisyTheme =
|
|
| "light"
|
|
| "dark"
|
|
| "cupcake"
|
|
| "bumblebee"
|
|
| "emerald"
|
|
| "corporate"
|
|
| "synthwave"
|
|
| "retro"
|
|
| "cyberpunk"
|
|
| "valentine"
|
|
| "halloween"
|
|
| "garden"
|
|
| "forest"
|
|
| "aqua"
|
|
| "lofi"
|
|
| "pastel"
|
|
| "fantasy"
|
|
| "wireframe"
|
|
| "black"
|
|
| "luxury"
|
|
| "dracula"
|
|
| "cmyk"
|
|
| "autumn"
|
|
| "business"
|
|
| "acid"
|
|
| "lemonade"
|
|
| "night"
|
|
| "coffee"
|
|
| "winter";
|
|
|
|
export type LocationViewPreferences = {
|
|
showDetails: boolean;
|
|
showEmpty: boolean;
|
|
editorSimpleView: boolean;
|
|
theme: DaisyTheme;
|
|
};
|
|
|
|
/**
|
|
* useViewPreferences loads the view preferences from local storage and hydrates
|
|
* them. These are reactive and will update the local storage when changed.
|
|
*/
|
|
export function useViewPreferences(): Ref<LocationViewPreferences> {
|
|
const results = useLocalStorage(
|
|
"homebox/preferences/location",
|
|
{
|
|
showDetails: true,
|
|
showEmpty: true,
|
|
editorSimpleView: true,
|
|
theme: "garden",
|
|
},
|
|
{ mergeDefaults: true }
|
|
);
|
|
|
|
// casting is required because the type returned is removable, however since we
|
|
// use `mergeDefaults` the result _should_ always be present.
|
|
return results as unknown as Ref<LocationViewPreferences>;
|
|
}
|