mirror of
https://github.com/hay-kot/homebox.git
synced 2024-11-22 16:45:43 +00:00
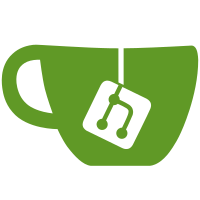
* basic currency service for loading at runtime
* api endpoint for currencies
* sort slice before return
* remove currency validation
* validate using currency service
* implement selecting dynamic currency options
* bump go version
* fix type definition
* specify explicit type
* change go versions
* proper types for assetId
* log/return currency error
* make case insensative
* use ToUpper instead
* feat: adding new currencies (#715)
* fix: task swag (#710)
Co-authored-by: Quoing <pavel.cadersky@mavenir.com>
* [feat] Adding new currencies
---------
Co-authored-by: quoing <quoing@users.noreply.github.com>
Co-authored-by: Quoing <pavel.cadersky@mavenir.com>
Co-authored-by: Bradley <41597815+userbradley@users.noreply.github.com>
* remove ts file and consoldate new values into json
* move flag to options namespace
* add env config for currencies
* basic documentaion
* remove in sync test
---------
Co-authored-by: quoing <quoing@users.noreply.github.com>
Co-authored-by: Quoing <pavel.cadersky@mavenir.com>
Co-authored-by: Bradley <41597815+userbradley@users.noreply.github.com>
Former-commit-id: c4b923847a
67 lines
1.5 KiB
Go
67 lines
1.5 KiB
Go
// Package services provides the core business logic for the application.
|
|
package services
|
|
|
|
import (
|
|
"github.com/hay-kot/homebox/backend/internal/core/currencies"
|
|
"github.com/hay-kot/homebox/backend/internal/data/repo"
|
|
)
|
|
|
|
type AllServices struct {
|
|
User *UserService
|
|
Group *GroupService
|
|
Items *ItemService
|
|
BackgroundService *BackgroundService
|
|
Currencies *currencies.CurrencyRegistry
|
|
}
|
|
|
|
type OptionsFunc func(*options)
|
|
|
|
type options struct {
|
|
autoIncrementAssetID bool
|
|
currencies []currencies.Currency
|
|
}
|
|
|
|
func WithAutoIncrementAssetID(v bool) func(*options) {
|
|
return func(o *options) {
|
|
o.autoIncrementAssetID = v
|
|
}
|
|
}
|
|
|
|
func WithCurrencies(v []currencies.Currency) func(*options) {
|
|
return func(o *options) {
|
|
o.currencies = v
|
|
}
|
|
}
|
|
|
|
func New(repos *repo.AllRepos, opts ...OptionsFunc) *AllServices {
|
|
if repos == nil {
|
|
panic("repos cannot be nil")
|
|
}
|
|
|
|
defaultCurrencies, err := currencies.CollectionCurrencies(
|
|
currencies.CollectDefaults(),
|
|
)
|
|
if err != nil {
|
|
panic("failed to collect default currencies")
|
|
}
|
|
|
|
options := &options{
|
|
autoIncrementAssetID: true,
|
|
currencies: defaultCurrencies,
|
|
}
|
|
|
|
for _, opt := range opts {
|
|
opt(options)
|
|
}
|
|
|
|
return &AllServices{
|
|
User: &UserService{repos},
|
|
Group: &GroupService{repos},
|
|
Items: &ItemService{
|
|
repo: repos,
|
|
autoIncrementAssetID: options.autoIncrementAssetID,
|
|
},
|
|
BackgroundService: &BackgroundService{repos},
|
|
Currencies: currencies.NewCurrencyService(options.currencies),
|
|
}
|
|
}
|