mirror of
https://github.com/hay-kot/homebox.git
synced 2024-11-16 21:58:40 +00:00
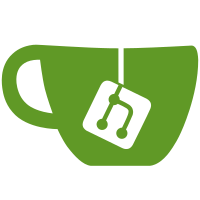
Add archive option feature. Archived items can only be seen on the items page when including archived is selected. Archived items are excluded from the count and from other views
2634 lines
72 KiB
Go
2634 lines
72 KiB
Go
// Code generated by ent, DO NOT EDIT.
|
|
|
|
package ent
|
|
|
|
import (
|
|
"context"
|
|
"errors"
|
|
"fmt"
|
|
"time"
|
|
|
|
"entgo.io/ent/dialect/sql"
|
|
"entgo.io/ent/dialect/sql/sqlgraph"
|
|
"entgo.io/ent/schema/field"
|
|
"github.com/google/uuid"
|
|
"github.com/hay-kot/homebox/backend/internal/data/ent/attachment"
|
|
"github.com/hay-kot/homebox/backend/internal/data/ent/group"
|
|
"github.com/hay-kot/homebox/backend/internal/data/ent/item"
|
|
"github.com/hay-kot/homebox/backend/internal/data/ent/itemfield"
|
|
"github.com/hay-kot/homebox/backend/internal/data/ent/label"
|
|
"github.com/hay-kot/homebox/backend/internal/data/ent/location"
|
|
"github.com/hay-kot/homebox/backend/internal/data/ent/predicate"
|
|
)
|
|
|
|
// ItemUpdate is the builder for updating Item entities.
|
|
type ItemUpdate struct {
|
|
config
|
|
hooks []Hook
|
|
mutation *ItemMutation
|
|
}
|
|
|
|
// Where appends a list predicates to the ItemUpdate builder.
|
|
func (iu *ItemUpdate) Where(ps ...predicate.Item) *ItemUpdate {
|
|
iu.mutation.Where(ps...)
|
|
return iu
|
|
}
|
|
|
|
// SetUpdatedAt sets the "updated_at" field.
|
|
func (iu *ItemUpdate) SetUpdatedAt(t time.Time) *ItemUpdate {
|
|
iu.mutation.SetUpdatedAt(t)
|
|
return iu
|
|
}
|
|
|
|
// SetName sets the "name" field.
|
|
func (iu *ItemUpdate) SetName(s string) *ItemUpdate {
|
|
iu.mutation.SetName(s)
|
|
return iu
|
|
}
|
|
|
|
// SetDescription sets the "description" field.
|
|
func (iu *ItemUpdate) SetDescription(s string) *ItemUpdate {
|
|
iu.mutation.SetDescription(s)
|
|
return iu
|
|
}
|
|
|
|
// SetNillableDescription sets the "description" field if the given value is not nil.
|
|
func (iu *ItemUpdate) SetNillableDescription(s *string) *ItemUpdate {
|
|
if s != nil {
|
|
iu.SetDescription(*s)
|
|
}
|
|
return iu
|
|
}
|
|
|
|
// ClearDescription clears the value of the "description" field.
|
|
func (iu *ItemUpdate) ClearDescription() *ItemUpdate {
|
|
iu.mutation.ClearDescription()
|
|
return iu
|
|
}
|
|
|
|
// SetNotes sets the "notes" field.
|
|
func (iu *ItemUpdate) SetNotes(s string) *ItemUpdate {
|
|
iu.mutation.SetNotes(s)
|
|
return iu
|
|
}
|
|
|
|
// SetNillableNotes sets the "notes" field if the given value is not nil.
|
|
func (iu *ItemUpdate) SetNillableNotes(s *string) *ItemUpdate {
|
|
if s != nil {
|
|
iu.SetNotes(*s)
|
|
}
|
|
return iu
|
|
}
|
|
|
|
// ClearNotes clears the value of the "notes" field.
|
|
func (iu *ItemUpdate) ClearNotes() *ItemUpdate {
|
|
iu.mutation.ClearNotes()
|
|
return iu
|
|
}
|
|
|
|
// SetQuantity sets the "quantity" field.
|
|
func (iu *ItemUpdate) SetQuantity(i int) *ItemUpdate {
|
|
iu.mutation.ResetQuantity()
|
|
iu.mutation.SetQuantity(i)
|
|
return iu
|
|
}
|
|
|
|
// SetNillableQuantity sets the "quantity" field if the given value is not nil.
|
|
func (iu *ItemUpdate) SetNillableQuantity(i *int) *ItemUpdate {
|
|
if i != nil {
|
|
iu.SetQuantity(*i)
|
|
}
|
|
return iu
|
|
}
|
|
|
|
// AddQuantity adds i to the "quantity" field.
|
|
func (iu *ItemUpdate) AddQuantity(i int) *ItemUpdate {
|
|
iu.mutation.AddQuantity(i)
|
|
return iu
|
|
}
|
|
|
|
// SetInsured sets the "insured" field.
|
|
func (iu *ItemUpdate) SetInsured(b bool) *ItemUpdate {
|
|
iu.mutation.SetInsured(b)
|
|
return iu
|
|
}
|
|
|
|
// SetNillableInsured sets the "insured" field if the given value is not nil.
|
|
func (iu *ItemUpdate) SetNillableInsured(b *bool) *ItemUpdate {
|
|
if b != nil {
|
|
iu.SetInsured(*b)
|
|
}
|
|
return iu
|
|
}
|
|
|
|
// SetArchived sets the "archived" field.
|
|
func (iu *ItemUpdate) SetArchived(b bool) *ItemUpdate {
|
|
iu.mutation.SetArchived(b)
|
|
return iu
|
|
}
|
|
|
|
// SetNillableArchived sets the "archived" field if the given value is not nil.
|
|
func (iu *ItemUpdate) SetNillableArchived(b *bool) *ItemUpdate {
|
|
if b != nil {
|
|
iu.SetArchived(*b)
|
|
}
|
|
return iu
|
|
}
|
|
|
|
// SetSerialNumber sets the "serial_number" field.
|
|
func (iu *ItemUpdate) SetSerialNumber(s string) *ItemUpdate {
|
|
iu.mutation.SetSerialNumber(s)
|
|
return iu
|
|
}
|
|
|
|
// SetNillableSerialNumber sets the "serial_number" field if the given value is not nil.
|
|
func (iu *ItemUpdate) SetNillableSerialNumber(s *string) *ItemUpdate {
|
|
if s != nil {
|
|
iu.SetSerialNumber(*s)
|
|
}
|
|
return iu
|
|
}
|
|
|
|
// ClearSerialNumber clears the value of the "serial_number" field.
|
|
func (iu *ItemUpdate) ClearSerialNumber() *ItemUpdate {
|
|
iu.mutation.ClearSerialNumber()
|
|
return iu
|
|
}
|
|
|
|
// SetModelNumber sets the "model_number" field.
|
|
func (iu *ItemUpdate) SetModelNumber(s string) *ItemUpdate {
|
|
iu.mutation.SetModelNumber(s)
|
|
return iu
|
|
}
|
|
|
|
// SetNillableModelNumber sets the "model_number" field if the given value is not nil.
|
|
func (iu *ItemUpdate) SetNillableModelNumber(s *string) *ItemUpdate {
|
|
if s != nil {
|
|
iu.SetModelNumber(*s)
|
|
}
|
|
return iu
|
|
}
|
|
|
|
// ClearModelNumber clears the value of the "model_number" field.
|
|
func (iu *ItemUpdate) ClearModelNumber() *ItemUpdate {
|
|
iu.mutation.ClearModelNumber()
|
|
return iu
|
|
}
|
|
|
|
// SetManufacturer sets the "manufacturer" field.
|
|
func (iu *ItemUpdate) SetManufacturer(s string) *ItemUpdate {
|
|
iu.mutation.SetManufacturer(s)
|
|
return iu
|
|
}
|
|
|
|
// SetNillableManufacturer sets the "manufacturer" field if the given value is not nil.
|
|
func (iu *ItemUpdate) SetNillableManufacturer(s *string) *ItemUpdate {
|
|
if s != nil {
|
|
iu.SetManufacturer(*s)
|
|
}
|
|
return iu
|
|
}
|
|
|
|
// ClearManufacturer clears the value of the "manufacturer" field.
|
|
func (iu *ItemUpdate) ClearManufacturer() *ItemUpdate {
|
|
iu.mutation.ClearManufacturer()
|
|
return iu
|
|
}
|
|
|
|
// SetLifetimeWarranty sets the "lifetime_warranty" field.
|
|
func (iu *ItemUpdate) SetLifetimeWarranty(b bool) *ItemUpdate {
|
|
iu.mutation.SetLifetimeWarranty(b)
|
|
return iu
|
|
}
|
|
|
|
// SetNillableLifetimeWarranty sets the "lifetime_warranty" field if the given value is not nil.
|
|
func (iu *ItemUpdate) SetNillableLifetimeWarranty(b *bool) *ItemUpdate {
|
|
if b != nil {
|
|
iu.SetLifetimeWarranty(*b)
|
|
}
|
|
return iu
|
|
}
|
|
|
|
// SetWarrantyExpires sets the "warranty_expires" field.
|
|
func (iu *ItemUpdate) SetWarrantyExpires(t time.Time) *ItemUpdate {
|
|
iu.mutation.SetWarrantyExpires(t)
|
|
return iu
|
|
}
|
|
|
|
// SetNillableWarrantyExpires sets the "warranty_expires" field if the given value is not nil.
|
|
func (iu *ItemUpdate) SetNillableWarrantyExpires(t *time.Time) *ItemUpdate {
|
|
if t != nil {
|
|
iu.SetWarrantyExpires(*t)
|
|
}
|
|
return iu
|
|
}
|
|
|
|
// ClearWarrantyExpires clears the value of the "warranty_expires" field.
|
|
func (iu *ItemUpdate) ClearWarrantyExpires() *ItemUpdate {
|
|
iu.mutation.ClearWarrantyExpires()
|
|
return iu
|
|
}
|
|
|
|
// SetWarrantyDetails sets the "warranty_details" field.
|
|
func (iu *ItemUpdate) SetWarrantyDetails(s string) *ItemUpdate {
|
|
iu.mutation.SetWarrantyDetails(s)
|
|
return iu
|
|
}
|
|
|
|
// SetNillableWarrantyDetails sets the "warranty_details" field if the given value is not nil.
|
|
func (iu *ItemUpdate) SetNillableWarrantyDetails(s *string) *ItemUpdate {
|
|
if s != nil {
|
|
iu.SetWarrantyDetails(*s)
|
|
}
|
|
return iu
|
|
}
|
|
|
|
// ClearWarrantyDetails clears the value of the "warranty_details" field.
|
|
func (iu *ItemUpdate) ClearWarrantyDetails() *ItemUpdate {
|
|
iu.mutation.ClearWarrantyDetails()
|
|
return iu
|
|
}
|
|
|
|
// SetPurchaseTime sets the "purchase_time" field.
|
|
func (iu *ItemUpdate) SetPurchaseTime(t time.Time) *ItemUpdate {
|
|
iu.mutation.SetPurchaseTime(t)
|
|
return iu
|
|
}
|
|
|
|
// SetNillablePurchaseTime sets the "purchase_time" field if the given value is not nil.
|
|
func (iu *ItemUpdate) SetNillablePurchaseTime(t *time.Time) *ItemUpdate {
|
|
if t != nil {
|
|
iu.SetPurchaseTime(*t)
|
|
}
|
|
return iu
|
|
}
|
|
|
|
// ClearPurchaseTime clears the value of the "purchase_time" field.
|
|
func (iu *ItemUpdate) ClearPurchaseTime() *ItemUpdate {
|
|
iu.mutation.ClearPurchaseTime()
|
|
return iu
|
|
}
|
|
|
|
// SetPurchaseFrom sets the "purchase_from" field.
|
|
func (iu *ItemUpdate) SetPurchaseFrom(s string) *ItemUpdate {
|
|
iu.mutation.SetPurchaseFrom(s)
|
|
return iu
|
|
}
|
|
|
|
// SetNillablePurchaseFrom sets the "purchase_from" field if the given value is not nil.
|
|
func (iu *ItemUpdate) SetNillablePurchaseFrom(s *string) *ItemUpdate {
|
|
if s != nil {
|
|
iu.SetPurchaseFrom(*s)
|
|
}
|
|
return iu
|
|
}
|
|
|
|
// ClearPurchaseFrom clears the value of the "purchase_from" field.
|
|
func (iu *ItemUpdate) ClearPurchaseFrom() *ItemUpdate {
|
|
iu.mutation.ClearPurchaseFrom()
|
|
return iu
|
|
}
|
|
|
|
// SetPurchasePrice sets the "purchase_price" field.
|
|
func (iu *ItemUpdate) SetPurchasePrice(f float64) *ItemUpdate {
|
|
iu.mutation.ResetPurchasePrice()
|
|
iu.mutation.SetPurchasePrice(f)
|
|
return iu
|
|
}
|
|
|
|
// SetNillablePurchasePrice sets the "purchase_price" field if the given value is not nil.
|
|
func (iu *ItemUpdate) SetNillablePurchasePrice(f *float64) *ItemUpdate {
|
|
if f != nil {
|
|
iu.SetPurchasePrice(*f)
|
|
}
|
|
return iu
|
|
}
|
|
|
|
// AddPurchasePrice adds f to the "purchase_price" field.
|
|
func (iu *ItemUpdate) AddPurchasePrice(f float64) *ItemUpdate {
|
|
iu.mutation.AddPurchasePrice(f)
|
|
return iu
|
|
}
|
|
|
|
// SetSoldTime sets the "sold_time" field.
|
|
func (iu *ItemUpdate) SetSoldTime(t time.Time) *ItemUpdate {
|
|
iu.mutation.SetSoldTime(t)
|
|
return iu
|
|
}
|
|
|
|
// SetNillableSoldTime sets the "sold_time" field if the given value is not nil.
|
|
func (iu *ItemUpdate) SetNillableSoldTime(t *time.Time) *ItemUpdate {
|
|
if t != nil {
|
|
iu.SetSoldTime(*t)
|
|
}
|
|
return iu
|
|
}
|
|
|
|
// ClearSoldTime clears the value of the "sold_time" field.
|
|
func (iu *ItemUpdate) ClearSoldTime() *ItemUpdate {
|
|
iu.mutation.ClearSoldTime()
|
|
return iu
|
|
}
|
|
|
|
// SetSoldTo sets the "sold_to" field.
|
|
func (iu *ItemUpdate) SetSoldTo(s string) *ItemUpdate {
|
|
iu.mutation.SetSoldTo(s)
|
|
return iu
|
|
}
|
|
|
|
// SetNillableSoldTo sets the "sold_to" field if the given value is not nil.
|
|
func (iu *ItemUpdate) SetNillableSoldTo(s *string) *ItemUpdate {
|
|
if s != nil {
|
|
iu.SetSoldTo(*s)
|
|
}
|
|
return iu
|
|
}
|
|
|
|
// ClearSoldTo clears the value of the "sold_to" field.
|
|
func (iu *ItemUpdate) ClearSoldTo() *ItemUpdate {
|
|
iu.mutation.ClearSoldTo()
|
|
return iu
|
|
}
|
|
|
|
// SetSoldPrice sets the "sold_price" field.
|
|
func (iu *ItemUpdate) SetSoldPrice(f float64) *ItemUpdate {
|
|
iu.mutation.ResetSoldPrice()
|
|
iu.mutation.SetSoldPrice(f)
|
|
return iu
|
|
}
|
|
|
|
// SetNillableSoldPrice sets the "sold_price" field if the given value is not nil.
|
|
func (iu *ItemUpdate) SetNillableSoldPrice(f *float64) *ItemUpdate {
|
|
if f != nil {
|
|
iu.SetSoldPrice(*f)
|
|
}
|
|
return iu
|
|
}
|
|
|
|
// AddSoldPrice adds f to the "sold_price" field.
|
|
func (iu *ItemUpdate) AddSoldPrice(f float64) *ItemUpdate {
|
|
iu.mutation.AddSoldPrice(f)
|
|
return iu
|
|
}
|
|
|
|
// SetSoldNotes sets the "sold_notes" field.
|
|
func (iu *ItemUpdate) SetSoldNotes(s string) *ItemUpdate {
|
|
iu.mutation.SetSoldNotes(s)
|
|
return iu
|
|
}
|
|
|
|
// SetNillableSoldNotes sets the "sold_notes" field if the given value is not nil.
|
|
func (iu *ItemUpdate) SetNillableSoldNotes(s *string) *ItemUpdate {
|
|
if s != nil {
|
|
iu.SetSoldNotes(*s)
|
|
}
|
|
return iu
|
|
}
|
|
|
|
// ClearSoldNotes clears the value of the "sold_notes" field.
|
|
func (iu *ItemUpdate) ClearSoldNotes() *ItemUpdate {
|
|
iu.mutation.ClearSoldNotes()
|
|
return iu
|
|
}
|
|
|
|
// SetParentID sets the "parent" edge to the Item entity by ID.
|
|
func (iu *ItemUpdate) SetParentID(id uuid.UUID) *ItemUpdate {
|
|
iu.mutation.SetParentID(id)
|
|
return iu
|
|
}
|
|
|
|
// SetNillableParentID sets the "parent" edge to the Item entity by ID if the given value is not nil.
|
|
func (iu *ItemUpdate) SetNillableParentID(id *uuid.UUID) *ItemUpdate {
|
|
if id != nil {
|
|
iu = iu.SetParentID(*id)
|
|
}
|
|
return iu
|
|
}
|
|
|
|
// SetParent sets the "parent" edge to the Item entity.
|
|
func (iu *ItemUpdate) SetParent(i *Item) *ItemUpdate {
|
|
return iu.SetParentID(i.ID)
|
|
}
|
|
|
|
// AddChildIDs adds the "children" edge to the Item entity by IDs.
|
|
func (iu *ItemUpdate) AddChildIDs(ids ...uuid.UUID) *ItemUpdate {
|
|
iu.mutation.AddChildIDs(ids...)
|
|
return iu
|
|
}
|
|
|
|
// AddChildren adds the "children" edges to the Item entity.
|
|
func (iu *ItemUpdate) AddChildren(i ...*Item) *ItemUpdate {
|
|
ids := make([]uuid.UUID, len(i))
|
|
for j := range i {
|
|
ids[j] = i[j].ID
|
|
}
|
|
return iu.AddChildIDs(ids...)
|
|
}
|
|
|
|
// SetGroupID sets the "group" edge to the Group entity by ID.
|
|
func (iu *ItemUpdate) SetGroupID(id uuid.UUID) *ItemUpdate {
|
|
iu.mutation.SetGroupID(id)
|
|
return iu
|
|
}
|
|
|
|
// SetGroup sets the "group" edge to the Group entity.
|
|
func (iu *ItemUpdate) SetGroup(g *Group) *ItemUpdate {
|
|
return iu.SetGroupID(g.ID)
|
|
}
|
|
|
|
// AddLabelIDs adds the "label" edge to the Label entity by IDs.
|
|
func (iu *ItemUpdate) AddLabelIDs(ids ...uuid.UUID) *ItemUpdate {
|
|
iu.mutation.AddLabelIDs(ids...)
|
|
return iu
|
|
}
|
|
|
|
// AddLabel adds the "label" edges to the Label entity.
|
|
func (iu *ItemUpdate) AddLabel(l ...*Label) *ItemUpdate {
|
|
ids := make([]uuid.UUID, len(l))
|
|
for i := range l {
|
|
ids[i] = l[i].ID
|
|
}
|
|
return iu.AddLabelIDs(ids...)
|
|
}
|
|
|
|
// SetLocationID sets the "location" edge to the Location entity by ID.
|
|
func (iu *ItemUpdate) SetLocationID(id uuid.UUID) *ItemUpdate {
|
|
iu.mutation.SetLocationID(id)
|
|
return iu
|
|
}
|
|
|
|
// SetNillableLocationID sets the "location" edge to the Location entity by ID if the given value is not nil.
|
|
func (iu *ItemUpdate) SetNillableLocationID(id *uuid.UUID) *ItemUpdate {
|
|
if id != nil {
|
|
iu = iu.SetLocationID(*id)
|
|
}
|
|
return iu
|
|
}
|
|
|
|
// SetLocation sets the "location" edge to the Location entity.
|
|
func (iu *ItemUpdate) SetLocation(l *Location) *ItemUpdate {
|
|
return iu.SetLocationID(l.ID)
|
|
}
|
|
|
|
// AddFieldIDs adds the "fields" edge to the ItemField entity by IDs.
|
|
func (iu *ItemUpdate) AddFieldIDs(ids ...uuid.UUID) *ItemUpdate {
|
|
iu.mutation.AddFieldIDs(ids...)
|
|
return iu
|
|
}
|
|
|
|
// AddFields adds the "fields" edges to the ItemField entity.
|
|
func (iu *ItemUpdate) AddFields(i ...*ItemField) *ItemUpdate {
|
|
ids := make([]uuid.UUID, len(i))
|
|
for j := range i {
|
|
ids[j] = i[j].ID
|
|
}
|
|
return iu.AddFieldIDs(ids...)
|
|
}
|
|
|
|
// AddAttachmentIDs adds the "attachments" edge to the Attachment entity by IDs.
|
|
func (iu *ItemUpdate) AddAttachmentIDs(ids ...uuid.UUID) *ItemUpdate {
|
|
iu.mutation.AddAttachmentIDs(ids...)
|
|
return iu
|
|
}
|
|
|
|
// AddAttachments adds the "attachments" edges to the Attachment entity.
|
|
func (iu *ItemUpdate) AddAttachments(a ...*Attachment) *ItemUpdate {
|
|
ids := make([]uuid.UUID, len(a))
|
|
for i := range a {
|
|
ids[i] = a[i].ID
|
|
}
|
|
return iu.AddAttachmentIDs(ids...)
|
|
}
|
|
|
|
// Mutation returns the ItemMutation object of the builder.
|
|
func (iu *ItemUpdate) Mutation() *ItemMutation {
|
|
return iu.mutation
|
|
}
|
|
|
|
// ClearParent clears the "parent" edge to the Item entity.
|
|
func (iu *ItemUpdate) ClearParent() *ItemUpdate {
|
|
iu.mutation.ClearParent()
|
|
return iu
|
|
}
|
|
|
|
// ClearChildren clears all "children" edges to the Item entity.
|
|
func (iu *ItemUpdate) ClearChildren() *ItemUpdate {
|
|
iu.mutation.ClearChildren()
|
|
return iu
|
|
}
|
|
|
|
// RemoveChildIDs removes the "children" edge to Item entities by IDs.
|
|
func (iu *ItemUpdate) RemoveChildIDs(ids ...uuid.UUID) *ItemUpdate {
|
|
iu.mutation.RemoveChildIDs(ids...)
|
|
return iu
|
|
}
|
|
|
|
// RemoveChildren removes "children" edges to Item entities.
|
|
func (iu *ItemUpdate) RemoveChildren(i ...*Item) *ItemUpdate {
|
|
ids := make([]uuid.UUID, len(i))
|
|
for j := range i {
|
|
ids[j] = i[j].ID
|
|
}
|
|
return iu.RemoveChildIDs(ids...)
|
|
}
|
|
|
|
// ClearGroup clears the "group" edge to the Group entity.
|
|
func (iu *ItemUpdate) ClearGroup() *ItemUpdate {
|
|
iu.mutation.ClearGroup()
|
|
return iu
|
|
}
|
|
|
|
// ClearLabel clears all "label" edges to the Label entity.
|
|
func (iu *ItemUpdate) ClearLabel() *ItemUpdate {
|
|
iu.mutation.ClearLabel()
|
|
return iu
|
|
}
|
|
|
|
// RemoveLabelIDs removes the "label" edge to Label entities by IDs.
|
|
func (iu *ItemUpdate) RemoveLabelIDs(ids ...uuid.UUID) *ItemUpdate {
|
|
iu.mutation.RemoveLabelIDs(ids...)
|
|
return iu
|
|
}
|
|
|
|
// RemoveLabel removes "label" edges to Label entities.
|
|
func (iu *ItemUpdate) RemoveLabel(l ...*Label) *ItemUpdate {
|
|
ids := make([]uuid.UUID, len(l))
|
|
for i := range l {
|
|
ids[i] = l[i].ID
|
|
}
|
|
return iu.RemoveLabelIDs(ids...)
|
|
}
|
|
|
|
// ClearLocation clears the "location" edge to the Location entity.
|
|
func (iu *ItemUpdate) ClearLocation() *ItemUpdate {
|
|
iu.mutation.ClearLocation()
|
|
return iu
|
|
}
|
|
|
|
// ClearFields clears all "fields" edges to the ItemField entity.
|
|
func (iu *ItemUpdate) ClearFields() *ItemUpdate {
|
|
iu.mutation.ClearFields()
|
|
return iu
|
|
}
|
|
|
|
// RemoveFieldIDs removes the "fields" edge to ItemField entities by IDs.
|
|
func (iu *ItemUpdate) RemoveFieldIDs(ids ...uuid.UUID) *ItemUpdate {
|
|
iu.mutation.RemoveFieldIDs(ids...)
|
|
return iu
|
|
}
|
|
|
|
// RemoveFields removes "fields" edges to ItemField entities.
|
|
func (iu *ItemUpdate) RemoveFields(i ...*ItemField) *ItemUpdate {
|
|
ids := make([]uuid.UUID, len(i))
|
|
for j := range i {
|
|
ids[j] = i[j].ID
|
|
}
|
|
return iu.RemoveFieldIDs(ids...)
|
|
}
|
|
|
|
// ClearAttachments clears all "attachments" edges to the Attachment entity.
|
|
func (iu *ItemUpdate) ClearAttachments() *ItemUpdate {
|
|
iu.mutation.ClearAttachments()
|
|
return iu
|
|
}
|
|
|
|
// RemoveAttachmentIDs removes the "attachments" edge to Attachment entities by IDs.
|
|
func (iu *ItemUpdate) RemoveAttachmentIDs(ids ...uuid.UUID) *ItemUpdate {
|
|
iu.mutation.RemoveAttachmentIDs(ids...)
|
|
return iu
|
|
}
|
|
|
|
// RemoveAttachments removes "attachments" edges to Attachment entities.
|
|
func (iu *ItemUpdate) RemoveAttachments(a ...*Attachment) *ItemUpdate {
|
|
ids := make([]uuid.UUID, len(a))
|
|
for i := range a {
|
|
ids[i] = a[i].ID
|
|
}
|
|
return iu.RemoveAttachmentIDs(ids...)
|
|
}
|
|
|
|
// Save executes the query and returns the number of nodes affected by the update operation.
|
|
func (iu *ItemUpdate) Save(ctx context.Context) (int, error) {
|
|
var (
|
|
err error
|
|
affected int
|
|
)
|
|
iu.defaults()
|
|
if len(iu.hooks) == 0 {
|
|
if err = iu.check(); err != nil {
|
|
return 0, err
|
|
}
|
|
affected, err = iu.sqlSave(ctx)
|
|
} else {
|
|
var mut Mutator = MutateFunc(func(ctx context.Context, m Mutation) (Value, error) {
|
|
mutation, ok := m.(*ItemMutation)
|
|
if !ok {
|
|
return nil, fmt.Errorf("unexpected mutation type %T", m)
|
|
}
|
|
if err = iu.check(); err != nil {
|
|
return 0, err
|
|
}
|
|
iu.mutation = mutation
|
|
affected, err = iu.sqlSave(ctx)
|
|
mutation.done = true
|
|
return affected, err
|
|
})
|
|
for i := len(iu.hooks) - 1; i >= 0; i-- {
|
|
if iu.hooks[i] == nil {
|
|
return 0, fmt.Errorf("ent: uninitialized hook (forgotten import ent/runtime?)")
|
|
}
|
|
mut = iu.hooks[i](mut)
|
|
}
|
|
if _, err := mut.Mutate(ctx, iu.mutation); err != nil {
|
|
return 0, err
|
|
}
|
|
}
|
|
return affected, err
|
|
}
|
|
|
|
// SaveX is like Save, but panics if an error occurs.
|
|
func (iu *ItemUpdate) SaveX(ctx context.Context) int {
|
|
affected, err := iu.Save(ctx)
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
return affected
|
|
}
|
|
|
|
// Exec executes the query.
|
|
func (iu *ItemUpdate) Exec(ctx context.Context) error {
|
|
_, err := iu.Save(ctx)
|
|
return err
|
|
}
|
|
|
|
// ExecX is like Exec, but panics if an error occurs.
|
|
func (iu *ItemUpdate) ExecX(ctx context.Context) {
|
|
if err := iu.Exec(ctx); err != nil {
|
|
panic(err)
|
|
}
|
|
}
|
|
|
|
// defaults sets the default values of the builder before save.
|
|
func (iu *ItemUpdate) defaults() {
|
|
if _, ok := iu.mutation.UpdatedAt(); !ok {
|
|
v := item.UpdateDefaultUpdatedAt()
|
|
iu.mutation.SetUpdatedAt(v)
|
|
}
|
|
}
|
|
|
|
// check runs all checks and user-defined validators on the builder.
|
|
func (iu *ItemUpdate) check() error {
|
|
if v, ok := iu.mutation.Name(); ok {
|
|
if err := item.NameValidator(v); err != nil {
|
|
return &ValidationError{Name: "name", err: fmt.Errorf(`ent: validator failed for field "Item.name": %w`, err)}
|
|
}
|
|
}
|
|
if v, ok := iu.mutation.Description(); ok {
|
|
if err := item.DescriptionValidator(v); err != nil {
|
|
return &ValidationError{Name: "description", err: fmt.Errorf(`ent: validator failed for field "Item.description": %w`, err)}
|
|
}
|
|
}
|
|
if v, ok := iu.mutation.Notes(); ok {
|
|
if err := item.NotesValidator(v); err != nil {
|
|
return &ValidationError{Name: "notes", err: fmt.Errorf(`ent: validator failed for field "Item.notes": %w`, err)}
|
|
}
|
|
}
|
|
if v, ok := iu.mutation.SerialNumber(); ok {
|
|
if err := item.SerialNumberValidator(v); err != nil {
|
|
return &ValidationError{Name: "serial_number", err: fmt.Errorf(`ent: validator failed for field "Item.serial_number": %w`, err)}
|
|
}
|
|
}
|
|
if v, ok := iu.mutation.ModelNumber(); ok {
|
|
if err := item.ModelNumberValidator(v); err != nil {
|
|
return &ValidationError{Name: "model_number", err: fmt.Errorf(`ent: validator failed for field "Item.model_number": %w`, err)}
|
|
}
|
|
}
|
|
if v, ok := iu.mutation.Manufacturer(); ok {
|
|
if err := item.ManufacturerValidator(v); err != nil {
|
|
return &ValidationError{Name: "manufacturer", err: fmt.Errorf(`ent: validator failed for field "Item.manufacturer": %w`, err)}
|
|
}
|
|
}
|
|
if v, ok := iu.mutation.WarrantyDetails(); ok {
|
|
if err := item.WarrantyDetailsValidator(v); err != nil {
|
|
return &ValidationError{Name: "warranty_details", err: fmt.Errorf(`ent: validator failed for field "Item.warranty_details": %w`, err)}
|
|
}
|
|
}
|
|
if v, ok := iu.mutation.SoldNotes(); ok {
|
|
if err := item.SoldNotesValidator(v); err != nil {
|
|
return &ValidationError{Name: "sold_notes", err: fmt.Errorf(`ent: validator failed for field "Item.sold_notes": %w`, err)}
|
|
}
|
|
}
|
|
if _, ok := iu.mutation.GroupID(); iu.mutation.GroupCleared() && !ok {
|
|
return errors.New(`ent: clearing a required unique edge "Item.group"`)
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (iu *ItemUpdate) sqlSave(ctx context.Context) (n int, err error) {
|
|
_spec := &sqlgraph.UpdateSpec{
|
|
Node: &sqlgraph.NodeSpec{
|
|
Table: item.Table,
|
|
Columns: item.Columns,
|
|
ID: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: item.FieldID,
|
|
},
|
|
},
|
|
}
|
|
if ps := iu.mutation.predicates; len(ps) > 0 {
|
|
_spec.Predicate = func(selector *sql.Selector) {
|
|
for i := range ps {
|
|
ps[i](selector)
|
|
}
|
|
}
|
|
}
|
|
if value, ok := iu.mutation.UpdatedAt(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeTime,
|
|
Value: value,
|
|
Column: item.FieldUpdatedAt,
|
|
})
|
|
}
|
|
if value, ok := iu.mutation.Name(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Value: value,
|
|
Column: item.FieldName,
|
|
})
|
|
}
|
|
if value, ok := iu.mutation.Description(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Value: value,
|
|
Column: item.FieldDescription,
|
|
})
|
|
}
|
|
if iu.mutation.DescriptionCleared() {
|
|
_spec.Fields.Clear = append(_spec.Fields.Clear, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Column: item.FieldDescription,
|
|
})
|
|
}
|
|
if iu.mutation.ImportRefCleared() {
|
|
_spec.Fields.Clear = append(_spec.Fields.Clear, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Column: item.FieldImportRef,
|
|
})
|
|
}
|
|
if value, ok := iu.mutation.Notes(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Value: value,
|
|
Column: item.FieldNotes,
|
|
})
|
|
}
|
|
if iu.mutation.NotesCleared() {
|
|
_spec.Fields.Clear = append(_spec.Fields.Clear, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Column: item.FieldNotes,
|
|
})
|
|
}
|
|
if value, ok := iu.mutation.Quantity(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeInt,
|
|
Value: value,
|
|
Column: item.FieldQuantity,
|
|
})
|
|
}
|
|
if value, ok := iu.mutation.AddedQuantity(); ok {
|
|
_spec.Fields.Add = append(_spec.Fields.Add, &sqlgraph.FieldSpec{
|
|
Type: field.TypeInt,
|
|
Value: value,
|
|
Column: item.FieldQuantity,
|
|
})
|
|
}
|
|
if value, ok := iu.mutation.Insured(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeBool,
|
|
Value: value,
|
|
Column: item.FieldInsured,
|
|
})
|
|
}
|
|
if value, ok := iu.mutation.Archived(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeBool,
|
|
Value: value,
|
|
Column: item.FieldArchived,
|
|
})
|
|
}
|
|
if value, ok := iu.mutation.SerialNumber(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Value: value,
|
|
Column: item.FieldSerialNumber,
|
|
})
|
|
}
|
|
if iu.mutation.SerialNumberCleared() {
|
|
_spec.Fields.Clear = append(_spec.Fields.Clear, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Column: item.FieldSerialNumber,
|
|
})
|
|
}
|
|
if value, ok := iu.mutation.ModelNumber(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Value: value,
|
|
Column: item.FieldModelNumber,
|
|
})
|
|
}
|
|
if iu.mutation.ModelNumberCleared() {
|
|
_spec.Fields.Clear = append(_spec.Fields.Clear, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Column: item.FieldModelNumber,
|
|
})
|
|
}
|
|
if value, ok := iu.mutation.Manufacturer(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Value: value,
|
|
Column: item.FieldManufacturer,
|
|
})
|
|
}
|
|
if iu.mutation.ManufacturerCleared() {
|
|
_spec.Fields.Clear = append(_spec.Fields.Clear, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Column: item.FieldManufacturer,
|
|
})
|
|
}
|
|
if value, ok := iu.mutation.LifetimeWarranty(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeBool,
|
|
Value: value,
|
|
Column: item.FieldLifetimeWarranty,
|
|
})
|
|
}
|
|
if value, ok := iu.mutation.WarrantyExpires(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeTime,
|
|
Value: value,
|
|
Column: item.FieldWarrantyExpires,
|
|
})
|
|
}
|
|
if iu.mutation.WarrantyExpiresCleared() {
|
|
_spec.Fields.Clear = append(_spec.Fields.Clear, &sqlgraph.FieldSpec{
|
|
Type: field.TypeTime,
|
|
Column: item.FieldWarrantyExpires,
|
|
})
|
|
}
|
|
if value, ok := iu.mutation.WarrantyDetails(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Value: value,
|
|
Column: item.FieldWarrantyDetails,
|
|
})
|
|
}
|
|
if iu.mutation.WarrantyDetailsCleared() {
|
|
_spec.Fields.Clear = append(_spec.Fields.Clear, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Column: item.FieldWarrantyDetails,
|
|
})
|
|
}
|
|
if value, ok := iu.mutation.PurchaseTime(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeTime,
|
|
Value: value,
|
|
Column: item.FieldPurchaseTime,
|
|
})
|
|
}
|
|
if iu.mutation.PurchaseTimeCleared() {
|
|
_spec.Fields.Clear = append(_spec.Fields.Clear, &sqlgraph.FieldSpec{
|
|
Type: field.TypeTime,
|
|
Column: item.FieldPurchaseTime,
|
|
})
|
|
}
|
|
if value, ok := iu.mutation.PurchaseFrom(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Value: value,
|
|
Column: item.FieldPurchaseFrom,
|
|
})
|
|
}
|
|
if iu.mutation.PurchaseFromCleared() {
|
|
_spec.Fields.Clear = append(_spec.Fields.Clear, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Column: item.FieldPurchaseFrom,
|
|
})
|
|
}
|
|
if value, ok := iu.mutation.PurchasePrice(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeFloat64,
|
|
Value: value,
|
|
Column: item.FieldPurchasePrice,
|
|
})
|
|
}
|
|
if value, ok := iu.mutation.AddedPurchasePrice(); ok {
|
|
_spec.Fields.Add = append(_spec.Fields.Add, &sqlgraph.FieldSpec{
|
|
Type: field.TypeFloat64,
|
|
Value: value,
|
|
Column: item.FieldPurchasePrice,
|
|
})
|
|
}
|
|
if value, ok := iu.mutation.SoldTime(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeTime,
|
|
Value: value,
|
|
Column: item.FieldSoldTime,
|
|
})
|
|
}
|
|
if iu.mutation.SoldTimeCleared() {
|
|
_spec.Fields.Clear = append(_spec.Fields.Clear, &sqlgraph.FieldSpec{
|
|
Type: field.TypeTime,
|
|
Column: item.FieldSoldTime,
|
|
})
|
|
}
|
|
if value, ok := iu.mutation.SoldTo(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Value: value,
|
|
Column: item.FieldSoldTo,
|
|
})
|
|
}
|
|
if iu.mutation.SoldToCleared() {
|
|
_spec.Fields.Clear = append(_spec.Fields.Clear, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Column: item.FieldSoldTo,
|
|
})
|
|
}
|
|
if value, ok := iu.mutation.SoldPrice(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeFloat64,
|
|
Value: value,
|
|
Column: item.FieldSoldPrice,
|
|
})
|
|
}
|
|
if value, ok := iu.mutation.AddedSoldPrice(); ok {
|
|
_spec.Fields.Add = append(_spec.Fields.Add, &sqlgraph.FieldSpec{
|
|
Type: field.TypeFloat64,
|
|
Value: value,
|
|
Column: item.FieldSoldPrice,
|
|
})
|
|
}
|
|
if value, ok := iu.mutation.SoldNotes(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Value: value,
|
|
Column: item.FieldSoldNotes,
|
|
})
|
|
}
|
|
if iu.mutation.SoldNotesCleared() {
|
|
_spec.Fields.Clear = append(_spec.Fields.Clear, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Column: item.FieldSoldNotes,
|
|
})
|
|
}
|
|
if iu.mutation.ParentCleared() {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.M2O,
|
|
Inverse: true,
|
|
Table: item.ParentTable,
|
|
Columns: []string{item.ParentColumn},
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: item.FieldID,
|
|
},
|
|
},
|
|
}
|
|
_spec.Edges.Clear = append(_spec.Edges.Clear, edge)
|
|
}
|
|
if nodes := iu.mutation.ParentIDs(); len(nodes) > 0 {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.M2O,
|
|
Inverse: true,
|
|
Table: item.ParentTable,
|
|
Columns: []string{item.ParentColumn},
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: item.FieldID,
|
|
},
|
|
},
|
|
}
|
|
for _, k := range nodes {
|
|
edge.Target.Nodes = append(edge.Target.Nodes, k)
|
|
}
|
|
_spec.Edges.Add = append(_spec.Edges.Add, edge)
|
|
}
|
|
if iu.mutation.ChildrenCleared() {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.O2M,
|
|
Inverse: false,
|
|
Table: item.ChildrenTable,
|
|
Columns: []string{item.ChildrenColumn},
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: item.FieldID,
|
|
},
|
|
},
|
|
}
|
|
_spec.Edges.Clear = append(_spec.Edges.Clear, edge)
|
|
}
|
|
if nodes := iu.mutation.RemovedChildrenIDs(); len(nodes) > 0 && !iu.mutation.ChildrenCleared() {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.O2M,
|
|
Inverse: false,
|
|
Table: item.ChildrenTable,
|
|
Columns: []string{item.ChildrenColumn},
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: item.FieldID,
|
|
},
|
|
},
|
|
}
|
|
for _, k := range nodes {
|
|
edge.Target.Nodes = append(edge.Target.Nodes, k)
|
|
}
|
|
_spec.Edges.Clear = append(_spec.Edges.Clear, edge)
|
|
}
|
|
if nodes := iu.mutation.ChildrenIDs(); len(nodes) > 0 {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.O2M,
|
|
Inverse: false,
|
|
Table: item.ChildrenTable,
|
|
Columns: []string{item.ChildrenColumn},
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: item.FieldID,
|
|
},
|
|
},
|
|
}
|
|
for _, k := range nodes {
|
|
edge.Target.Nodes = append(edge.Target.Nodes, k)
|
|
}
|
|
_spec.Edges.Add = append(_spec.Edges.Add, edge)
|
|
}
|
|
if iu.mutation.GroupCleared() {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.M2O,
|
|
Inverse: true,
|
|
Table: item.GroupTable,
|
|
Columns: []string{item.GroupColumn},
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: group.FieldID,
|
|
},
|
|
},
|
|
}
|
|
_spec.Edges.Clear = append(_spec.Edges.Clear, edge)
|
|
}
|
|
if nodes := iu.mutation.GroupIDs(); len(nodes) > 0 {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.M2O,
|
|
Inverse: true,
|
|
Table: item.GroupTable,
|
|
Columns: []string{item.GroupColumn},
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: group.FieldID,
|
|
},
|
|
},
|
|
}
|
|
for _, k := range nodes {
|
|
edge.Target.Nodes = append(edge.Target.Nodes, k)
|
|
}
|
|
_spec.Edges.Add = append(_spec.Edges.Add, edge)
|
|
}
|
|
if iu.mutation.LabelCleared() {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.M2M,
|
|
Inverse: true,
|
|
Table: item.LabelTable,
|
|
Columns: item.LabelPrimaryKey,
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: label.FieldID,
|
|
},
|
|
},
|
|
}
|
|
_spec.Edges.Clear = append(_spec.Edges.Clear, edge)
|
|
}
|
|
if nodes := iu.mutation.RemovedLabelIDs(); len(nodes) > 0 && !iu.mutation.LabelCleared() {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.M2M,
|
|
Inverse: true,
|
|
Table: item.LabelTable,
|
|
Columns: item.LabelPrimaryKey,
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: label.FieldID,
|
|
},
|
|
},
|
|
}
|
|
for _, k := range nodes {
|
|
edge.Target.Nodes = append(edge.Target.Nodes, k)
|
|
}
|
|
_spec.Edges.Clear = append(_spec.Edges.Clear, edge)
|
|
}
|
|
if nodes := iu.mutation.LabelIDs(); len(nodes) > 0 {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.M2M,
|
|
Inverse: true,
|
|
Table: item.LabelTable,
|
|
Columns: item.LabelPrimaryKey,
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: label.FieldID,
|
|
},
|
|
},
|
|
}
|
|
for _, k := range nodes {
|
|
edge.Target.Nodes = append(edge.Target.Nodes, k)
|
|
}
|
|
_spec.Edges.Add = append(_spec.Edges.Add, edge)
|
|
}
|
|
if iu.mutation.LocationCleared() {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.M2O,
|
|
Inverse: true,
|
|
Table: item.LocationTable,
|
|
Columns: []string{item.LocationColumn},
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: location.FieldID,
|
|
},
|
|
},
|
|
}
|
|
_spec.Edges.Clear = append(_spec.Edges.Clear, edge)
|
|
}
|
|
if nodes := iu.mutation.LocationIDs(); len(nodes) > 0 {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.M2O,
|
|
Inverse: true,
|
|
Table: item.LocationTable,
|
|
Columns: []string{item.LocationColumn},
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: location.FieldID,
|
|
},
|
|
},
|
|
}
|
|
for _, k := range nodes {
|
|
edge.Target.Nodes = append(edge.Target.Nodes, k)
|
|
}
|
|
_spec.Edges.Add = append(_spec.Edges.Add, edge)
|
|
}
|
|
if iu.mutation.FieldsCleared() {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.O2M,
|
|
Inverse: false,
|
|
Table: item.FieldsTable,
|
|
Columns: []string{item.FieldsColumn},
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: itemfield.FieldID,
|
|
},
|
|
},
|
|
}
|
|
_spec.Edges.Clear = append(_spec.Edges.Clear, edge)
|
|
}
|
|
if nodes := iu.mutation.RemovedFieldsIDs(); len(nodes) > 0 && !iu.mutation.FieldsCleared() {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.O2M,
|
|
Inverse: false,
|
|
Table: item.FieldsTable,
|
|
Columns: []string{item.FieldsColumn},
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: itemfield.FieldID,
|
|
},
|
|
},
|
|
}
|
|
for _, k := range nodes {
|
|
edge.Target.Nodes = append(edge.Target.Nodes, k)
|
|
}
|
|
_spec.Edges.Clear = append(_spec.Edges.Clear, edge)
|
|
}
|
|
if nodes := iu.mutation.FieldsIDs(); len(nodes) > 0 {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.O2M,
|
|
Inverse: false,
|
|
Table: item.FieldsTable,
|
|
Columns: []string{item.FieldsColumn},
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: itemfield.FieldID,
|
|
},
|
|
},
|
|
}
|
|
for _, k := range nodes {
|
|
edge.Target.Nodes = append(edge.Target.Nodes, k)
|
|
}
|
|
_spec.Edges.Add = append(_spec.Edges.Add, edge)
|
|
}
|
|
if iu.mutation.AttachmentsCleared() {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.O2M,
|
|
Inverse: false,
|
|
Table: item.AttachmentsTable,
|
|
Columns: []string{item.AttachmentsColumn},
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: attachment.FieldID,
|
|
},
|
|
},
|
|
}
|
|
_spec.Edges.Clear = append(_spec.Edges.Clear, edge)
|
|
}
|
|
if nodes := iu.mutation.RemovedAttachmentsIDs(); len(nodes) > 0 && !iu.mutation.AttachmentsCleared() {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.O2M,
|
|
Inverse: false,
|
|
Table: item.AttachmentsTable,
|
|
Columns: []string{item.AttachmentsColumn},
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: attachment.FieldID,
|
|
},
|
|
},
|
|
}
|
|
for _, k := range nodes {
|
|
edge.Target.Nodes = append(edge.Target.Nodes, k)
|
|
}
|
|
_spec.Edges.Clear = append(_spec.Edges.Clear, edge)
|
|
}
|
|
if nodes := iu.mutation.AttachmentsIDs(); len(nodes) > 0 {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.O2M,
|
|
Inverse: false,
|
|
Table: item.AttachmentsTable,
|
|
Columns: []string{item.AttachmentsColumn},
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: attachment.FieldID,
|
|
},
|
|
},
|
|
}
|
|
for _, k := range nodes {
|
|
edge.Target.Nodes = append(edge.Target.Nodes, k)
|
|
}
|
|
_spec.Edges.Add = append(_spec.Edges.Add, edge)
|
|
}
|
|
if n, err = sqlgraph.UpdateNodes(ctx, iu.driver, _spec); err != nil {
|
|
if _, ok := err.(*sqlgraph.NotFoundError); ok {
|
|
err = &NotFoundError{item.Label}
|
|
} else if sqlgraph.IsConstraintError(err) {
|
|
err = &ConstraintError{msg: err.Error(), wrap: err}
|
|
}
|
|
return 0, err
|
|
}
|
|
return n, nil
|
|
}
|
|
|
|
// ItemUpdateOne is the builder for updating a single Item entity.
|
|
type ItemUpdateOne struct {
|
|
config
|
|
fields []string
|
|
hooks []Hook
|
|
mutation *ItemMutation
|
|
}
|
|
|
|
// SetUpdatedAt sets the "updated_at" field.
|
|
func (iuo *ItemUpdateOne) SetUpdatedAt(t time.Time) *ItemUpdateOne {
|
|
iuo.mutation.SetUpdatedAt(t)
|
|
return iuo
|
|
}
|
|
|
|
// SetName sets the "name" field.
|
|
func (iuo *ItemUpdateOne) SetName(s string) *ItemUpdateOne {
|
|
iuo.mutation.SetName(s)
|
|
return iuo
|
|
}
|
|
|
|
// SetDescription sets the "description" field.
|
|
func (iuo *ItemUpdateOne) SetDescription(s string) *ItemUpdateOne {
|
|
iuo.mutation.SetDescription(s)
|
|
return iuo
|
|
}
|
|
|
|
// SetNillableDescription sets the "description" field if the given value is not nil.
|
|
func (iuo *ItemUpdateOne) SetNillableDescription(s *string) *ItemUpdateOne {
|
|
if s != nil {
|
|
iuo.SetDescription(*s)
|
|
}
|
|
return iuo
|
|
}
|
|
|
|
// ClearDescription clears the value of the "description" field.
|
|
func (iuo *ItemUpdateOne) ClearDescription() *ItemUpdateOne {
|
|
iuo.mutation.ClearDescription()
|
|
return iuo
|
|
}
|
|
|
|
// SetNotes sets the "notes" field.
|
|
func (iuo *ItemUpdateOne) SetNotes(s string) *ItemUpdateOne {
|
|
iuo.mutation.SetNotes(s)
|
|
return iuo
|
|
}
|
|
|
|
// SetNillableNotes sets the "notes" field if the given value is not nil.
|
|
func (iuo *ItemUpdateOne) SetNillableNotes(s *string) *ItemUpdateOne {
|
|
if s != nil {
|
|
iuo.SetNotes(*s)
|
|
}
|
|
return iuo
|
|
}
|
|
|
|
// ClearNotes clears the value of the "notes" field.
|
|
func (iuo *ItemUpdateOne) ClearNotes() *ItemUpdateOne {
|
|
iuo.mutation.ClearNotes()
|
|
return iuo
|
|
}
|
|
|
|
// SetQuantity sets the "quantity" field.
|
|
func (iuo *ItemUpdateOne) SetQuantity(i int) *ItemUpdateOne {
|
|
iuo.mutation.ResetQuantity()
|
|
iuo.mutation.SetQuantity(i)
|
|
return iuo
|
|
}
|
|
|
|
// SetNillableQuantity sets the "quantity" field if the given value is not nil.
|
|
func (iuo *ItemUpdateOne) SetNillableQuantity(i *int) *ItemUpdateOne {
|
|
if i != nil {
|
|
iuo.SetQuantity(*i)
|
|
}
|
|
return iuo
|
|
}
|
|
|
|
// AddQuantity adds i to the "quantity" field.
|
|
func (iuo *ItemUpdateOne) AddQuantity(i int) *ItemUpdateOne {
|
|
iuo.mutation.AddQuantity(i)
|
|
return iuo
|
|
}
|
|
|
|
// SetInsured sets the "insured" field.
|
|
func (iuo *ItemUpdateOne) SetInsured(b bool) *ItemUpdateOne {
|
|
iuo.mutation.SetInsured(b)
|
|
return iuo
|
|
}
|
|
|
|
// SetNillableInsured sets the "insured" field if the given value is not nil.
|
|
func (iuo *ItemUpdateOne) SetNillableInsured(b *bool) *ItemUpdateOne {
|
|
if b != nil {
|
|
iuo.SetInsured(*b)
|
|
}
|
|
return iuo
|
|
}
|
|
|
|
// SetArchived sets the "archived" field.
|
|
func (iuo *ItemUpdateOne) SetArchived(b bool) *ItemUpdateOne {
|
|
iuo.mutation.SetArchived(b)
|
|
return iuo
|
|
}
|
|
|
|
// SetNillableArchived sets the "archived" field if the given value is not nil.
|
|
func (iuo *ItemUpdateOne) SetNillableArchived(b *bool) *ItemUpdateOne {
|
|
if b != nil {
|
|
iuo.SetArchived(*b)
|
|
}
|
|
return iuo
|
|
}
|
|
|
|
// SetSerialNumber sets the "serial_number" field.
|
|
func (iuo *ItemUpdateOne) SetSerialNumber(s string) *ItemUpdateOne {
|
|
iuo.mutation.SetSerialNumber(s)
|
|
return iuo
|
|
}
|
|
|
|
// SetNillableSerialNumber sets the "serial_number" field if the given value is not nil.
|
|
func (iuo *ItemUpdateOne) SetNillableSerialNumber(s *string) *ItemUpdateOne {
|
|
if s != nil {
|
|
iuo.SetSerialNumber(*s)
|
|
}
|
|
return iuo
|
|
}
|
|
|
|
// ClearSerialNumber clears the value of the "serial_number" field.
|
|
func (iuo *ItemUpdateOne) ClearSerialNumber() *ItemUpdateOne {
|
|
iuo.mutation.ClearSerialNumber()
|
|
return iuo
|
|
}
|
|
|
|
// SetModelNumber sets the "model_number" field.
|
|
func (iuo *ItemUpdateOne) SetModelNumber(s string) *ItemUpdateOne {
|
|
iuo.mutation.SetModelNumber(s)
|
|
return iuo
|
|
}
|
|
|
|
// SetNillableModelNumber sets the "model_number" field if the given value is not nil.
|
|
func (iuo *ItemUpdateOne) SetNillableModelNumber(s *string) *ItemUpdateOne {
|
|
if s != nil {
|
|
iuo.SetModelNumber(*s)
|
|
}
|
|
return iuo
|
|
}
|
|
|
|
// ClearModelNumber clears the value of the "model_number" field.
|
|
func (iuo *ItemUpdateOne) ClearModelNumber() *ItemUpdateOne {
|
|
iuo.mutation.ClearModelNumber()
|
|
return iuo
|
|
}
|
|
|
|
// SetManufacturer sets the "manufacturer" field.
|
|
func (iuo *ItemUpdateOne) SetManufacturer(s string) *ItemUpdateOne {
|
|
iuo.mutation.SetManufacturer(s)
|
|
return iuo
|
|
}
|
|
|
|
// SetNillableManufacturer sets the "manufacturer" field if the given value is not nil.
|
|
func (iuo *ItemUpdateOne) SetNillableManufacturer(s *string) *ItemUpdateOne {
|
|
if s != nil {
|
|
iuo.SetManufacturer(*s)
|
|
}
|
|
return iuo
|
|
}
|
|
|
|
// ClearManufacturer clears the value of the "manufacturer" field.
|
|
func (iuo *ItemUpdateOne) ClearManufacturer() *ItemUpdateOne {
|
|
iuo.mutation.ClearManufacturer()
|
|
return iuo
|
|
}
|
|
|
|
// SetLifetimeWarranty sets the "lifetime_warranty" field.
|
|
func (iuo *ItemUpdateOne) SetLifetimeWarranty(b bool) *ItemUpdateOne {
|
|
iuo.mutation.SetLifetimeWarranty(b)
|
|
return iuo
|
|
}
|
|
|
|
// SetNillableLifetimeWarranty sets the "lifetime_warranty" field if the given value is not nil.
|
|
func (iuo *ItemUpdateOne) SetNillableLifetimeWarranty(b *bool) *ItemUpdateOne {
|
|
if b != nil {
|
|
iuo.SetLifetimeWarranty(*b)
|
|
}
|
|
return iuo
|
|
}
|
|
|
|
// SetWarrantyExpires sets the "warranty_expires" field.
|
|
func (iuo *ItemUpdateOne) SetWarrantyExpires(t time.Time) *ItemUpdateOne {
|
|
iuo.mutation.SetWarrantyExpires(t)
|
|
return iuo
|
|
}
|
|
|
|
// SetNillableWarrantyExpires sets the "warranty_expires" field if the given value is not nil.
|
|
func (iuo *ItemUpdateOne) SetNillableWarrantyExpires(t *time.Time) *ItemUpdateOne {
|
|
if t != nil {
|
|
iuo.SetWarrantyExpires(*t)
|
|
}
|
|
return iuo
|
|
}
|
|
|
|
// ClearWarrantyExpires clears the value of the "warranty_expires" field.
|
|
func (iuo *ItemUpdateOne) ClearWarrantyExpires() *ItemUpdateOne {
|
|
iuo.mutation.ClearWarrantyExpires()
|
|
return iuo
|
|
}
|
|
|
|
// SetWarrantyDetails sets the "warranty_details" field.
|
|
func (iuo *ItemUpdateOne) SetWarrantyDetails(s string) *ItemUpdateOne {
|
|
iuo.mutation.SetWarrantyDetails(s)
|
|
return iuo
|
|
}
|
|
|
|
// SetNillableWarrantyDetails sets the "warranty_details" field if the given value is not nil.
|
|
func (iuo *ItemUpdateOne) SetNillableWarrantyDetails(s *string) *ItemUpdateOne {
|
|
if s != nil {
|
|
iuo.SetWarrantyDetails(*s)
|
|
}
|
|
return iuo
|
|
}
|
|
|
|
// ClearWarrantyDetails clears the value of the "warranty_details" field.
|
|
func (iuo *ItemUpdateOne) ClearWarrantyDetails() *ItemUpdateOne {
|
|
iuo.mutation.ClearWarrantyDetails()
|
|
return iuo
|
|
}
|
|
|
|
// SetPurchaseTime sets the "purchase_time" field.
|
|
func (iuo *ItemUpdateOne) SetPurchaseTime(t time.Time) *ItemUpdateOne {
|
|
iuo.mutation.SetPurchaseTime(t)
|
|
return iuo
|
|
}
|
|
|
|
// SetNillablePurchaseTime sets the "purchase_time" field if the given value is not nil.
|
|
func (iuo *ItemUpdateOne) SetNillablePurchaseTime(t *time.Time) *ItemUpdateOne {
|
|
if t != nil {
|
|
iuo.SetPurchaseTime(*t)
|
|
}
|
|
return iuo
|
|
}
|
|
|
|
// ClearPurchaseTime clears the value of the "purchase_time" field.
|
|
func (iuo *ItemUpdateOne) ClearPurchaseTime() *ItemUpdateOne {
|
|
iuo.mutation.ClearPurchaseTime()
|
|
return iuo
|
|
}
|
|
|
|
// SetPurchaseFrom sets the "purchase_from" field.
|
|
func (iuo *ItemUpdateOne) SetPurchaseFrom(s string) *ItemUpdateOne {
|
|
iuo.mutation.SetPurchaseFrom(s)
|
|
return iuo
|
|
}
|
|
|
|
// SetNillablePurchaseFrom sets the "purchase_from" field if the given value is not nil.
|
|
func (iuo *ItemUpdateOne) SetNillablePurchaseFrom(s *string) *ItemUpdateOne {
|
|
if s != nil {
|
|
iuo.SetPurchaseFrom(*s)
|
|
}
|
|
return iuo
|
|
}
|
|
|
|
// ClearPurchaseFrom clears the value of the "purchase_from" field.
|
|
func (iuo *ItemUpdateOne) ClearPurchaseFrom() *ItemUpdateOne {
|
|
iuo.mutation.ClearPurchaseFrom()
|
|
return iuo
|
|
}
|
|
|
|
// SetPurchasePrice sets the "purchase_price" field.
|
|
func (iuo *ItemUpdateOne) SetPurchasePrice(f float64) *ItemUpdateOne {
|
|
iuo.mutation.ResetPurchasePrice()
|
|
iuo.mutation.SetPurchasePrice(f)
|
|
return iuo
|
|
}
|
|
|
|
// SetNillablePurchasePrice sets the "purchase_price" field if the given value is not nil.
|
|
func (iuo *ItemUpdateOne) SetNillablePurchasePrice(f *float64) *ItemUpdateOne {
|
|
if f != nil {
|
|
iuo.SetPurchasePrice(*f)
|
|
}
|
|
return iuo
|
|
}
|
|
|
|
// AddPurchasePrice adds f to the "purchase_price" field.
|
|
func (iuo *ItemUpdateOne) AddPurchasePrice(f float64) *ItemUpdateOne {
|
|
iuo.mutation.AddPurchasePrice(f)
|
|
return iuo
|
|
}
|
|
|
|
// SetSoldTime sets the "sold_time" field.
|
|
func (iuo *ItemUpdateOne) SetSoldTime(t time.Time) *ItemUpdateOne {
|
|
iuo.mutation.SetSoldTime(t)
|
|
return iuo
|
|
}
|
|
|
|
// SetNillableSoldTime sets the "sold_time" field if the given value is not nil.
|
|
func (iuo *ItemUpdateOne) SetNillableSoldTime(t *time.Time) *ItemUpdateOne {
|
|
if t != nil {
|
|
iuo.SetSoldTime(*t)
|
|
}
|
|
return iuo
|
|
}
|
|
|
|
// ClearSoldTime clears the value of the "sold_time" field.
|
|
func (iuo *ItemUpdateOne) ClearSoldTime() *ItemUpdateOne {
|
|
iuo.mutation.ClearSoldTime()
|
|
return iuo
|
|
}
|
|
|
|
// SetSoldTo sets the "sold_to" field.
|
|
func (iuo *ItemUpdateOne) SetSoldTo(s string) *ItemUpdateOne {
|
|
iuo.mutation.SetSoldTo(s)
|
|
return iuo
|
|
}
|
|
|
|
// SetNillableSoldTo sets the "sold_to" field if the given value is not nil.
|
|
func (iuo *ItemUpdateOne) SetNillableSoldTo(s *string) *ItemUpdateOne {
|
|
if s != nil {
|
|
iuo.SetSoldTo(*s)
|
|
}
|
|
return iuo
|
|
}
|
|
|
|
// ClearSoldTo clears the value of the "sold_to" field.
|
|
func (iuo *ItemUpdateOne) ClearSoldTo() *ItemUpdateOne {
|
|
iuo.mutation.ClearSoldTo()
|
|
return iuo
|
|
}
|
|
|
|
// SetSoldPrice sets the "sold_price" field.
|
|
func (iuo *ItemUpdateOne) SetSoldPrice(f float64) *ItemUpdateOne {
|
|
iuo.mutation.ResetSoldPrice()
|
|
iuo.mutation.SetSoldPrice(f)
|
|
return iuo
|
|
}
|
|
|
|
// SetNillableSoldPrice sets the "sold_price" field if the given value is not nil.
|
|
func (iuo *ItemUpdateOne) SetNillableSoldPrice(f *float64) *ItemUpdateOne {
|
|
if f != nil {
|
|
iuo.SetSoldPrice(*f)
|
|
}
|
|
return iuo
|
|
}
|
|
|
|
// AddSoldPrice adds f to the "sold_price" field.
|
|
func (iuo *ItemUpdateOne) AddSoldPrice(f float64) *ItemUpdateOne {
|
|
iuo.mutation.AddSoldPrice(f)
|
|
return iuo
|
|
}
|
|
|
|
// SetSoldNotes sets the "sold_notes" field.
|
|
func (iuo *ItemUpdateOne) SetSoldNotes(s string) *ItemUpdateOne {
|
|
iuo.mutation.SetSoldNotes(s)
|
|
return iuo
|
|
}
|
|
|
|
// SetNillableSoldNotes sets the "sold_notes" field if the given value is not nil.
|
|
func (iuo *ItemUpdateOne) SetNillableSoldNotes(s *string) *ItemUpdateOne {
|
|
if s != nil {
|
|
iuo.SetSoldNotes(*s)
|
|
}
|
|
return iuo
|
|
}
|
|
|
|
// ClearSoldNotes clears the value of the "sold_notes" field.
|
|
func (iuo *ItemUpdateOne) ClearSoldNotes() *ItemUpdateOne {
|
|
iuo.mutation.ClearSoldNotes()
|
|
return iuo
|
|
}
|
|
|
|
// SetParentID sets the "parent" edge to the Item entity by ID.
|
|
func (iuo *ItemUpdateOne) SetParentID(id uuid.UUID) *ItemUpdateOne {
|
|
iuo.mutation.SetParentID(id)
|
|
return iuo
|
|
}
|
|
|
|
// SetNillableParentID sets the "parent" edge to the Item entity by ID if the given value is not nil.
|
|
func (iuo *ItemUpdateOne) SetNillableParentID(id *uuid.UUID) *ItemUpdateOne {
|
|
if id != nil {
|
|
iuo = iuo.SetParentID(*id)
|
|
}
|
|
return iuo
|
|
}
|
|
|
|
// SetParent sets the "parent" edge to the Item entity.
|
|
func (iuo *ItemUpdateOne) SetParent(i *Item) *ItemUpdateOne {
|
|
return iuo.SetParentID(i.ID)
|
|
}
|
|
|
|
// AddChildIDs adds the "children" edge to the Item entity by IDs.
|
|
func (iuo *ItemUpdateOne) AddChildIDs(ids ...uuid.UUID) *ItemUpdateOne {
|
|
iuo.mutation.AddChildIDs(ids...)
|
|
return iuo
|
|
}
|
|
|
|
// AddChildren adds the "children" edges to the Item entity.
|
|
func (iuo *ItemUpdateOne) AddChildren(i ...*Item) *ItemUpdateOne {
|
|
ids := make([]uuid.UUID, len(i))
|
|
for j := range i {
|
|
ids[j] = i[j].ID
|
|
}
|
|
return iuo.AddChildIDs(ids...)
|
|
}
|
|
|
|
// SetGroupID sets the "group" edge to the Group entity by ID.
|
|
func (iuo *ItemUpdateOne) SetGroupID(id uuid.UUID) *ItemUpdateOne {
|
|
iuo.mutation.SetGroupID(id)
|
|
return iuo
|
|
}
|
|
|
|
// SetGroup sets the "group" edge to the Group entity.
|
|
func (iuo *ItemUpdateOne) SetGroup(g *Group) *ItemUpdateOne {
|
|
return iuo.SetGroupID(g.ID)
|
|
}
|
|
|
|
// AddLabelIDs adds the "label" edge to the Label entity by IDs.
|
|
func (iuo *ItemUpdateOne) AddLabelIDs(ids ...uuid.UUID) *ItemUpdateOne {
|
|
iuo.mutation.AddLabelIDs(ids...)
|
|
return iuo
|
|
}
|
|
|
|
// AddLabel adds the "label" edges to the Label entity.
|
|
func (iuo *ItemUpdateOne) AddLabel(l ...*Label) *ItemUpdateOne {
|
|
ids := make([]uuid.UUID, len(l))
|
|
for i := range l {
|
|
ids[i] = l[i].ID
|
|
}
|
|
return iuo.AddLabelIDs(ids...)
|
|
}
|
|
|
|
// SetLocationID sets the "location" edge to the Location entity by ID.
|
|
func (iuo *ItemUpdateOne) SetLocationID(id uuid.UUID) *ItemUpdateOne {
|
|
iuo.mutation.SetLocationID(id)
|
|
return iuo
|
|
}
|
|
|
|
// SetNillableLocationID sets the "location" edge to the Location entity by ID if the given value is not nil.
|
|
func (iuo *ItemUpdateOne) SetNillableLocationID(id *uuid.UUID) *ItemUpdateOne {
|
|
if id != nil {
|
|
iuo = iuo.SetLocationID(*id)
|
|
}
|
|
return iuo
|
|
}
|
|
|
|
// SetLocation sets the "location" edge to the Location entity.
|
|
func (iuo *ItemUpdateOne) SetLocation(l *Location) *ItemUpdateOne {
|
|
return iuo.SetLocationID(l.ID)
|
|
}
|
|
|
|
// AddFieldIDs adds the "fields" edge to the ItemField entity by IDs.
|
|
func (iuo *ItemUpdateOne) AddFieldIDs(ids ...uuid.UUID) *ItemUpdateOne {
|
|
iuo.mutation.AddFieldIDs(ids...)
|
|
return iuo
|
|
}
|
|
|
|
// AddFields adds the "fields" edges to the ItemField entity.
|
|
func (iuo *ItemUpdateOne) AddFields(i ...*ItemField) *ItemUpdateOne {
|
|
ids := make([]uuid.UUID, len(i))
|
|
for j := range i {
|
|
ids[j] = i[j].ID
|
|
}
|
|
return iuo.AddFieldIDs(ids...)
|
|
}
|
|
|
|
// AddAttachmentIDs adds the "attachments" edge to the Attachment entity by IDs.
|
|
func (iuo *ItemUpdateOne) AddAttachmentIDs(ids ...uuid.UUID) *ItemUpdateOne {
|
|
iuo.mutation.AddAttachmentIDs(ids...)
|
|
return iuo
|
|
}
|
|
|
|
// AddAttachments adds the "attachments" edges to the Attachment entity.
|
|
func (iuo *ItemUpdateOne) AddAttachments(a ...*Attachment) *ItemUpdateOne {
|
|
ids := make([]uuid.UUID, len(a))
|
|
for i := range a {
|
|
ids[i] = a[i].ID
|
|
}
|
|
return iuo.AddAttachmentIDs(ids...)
|
|
}
|
|
|
|
// Mutation returns the ItemMutation object of the builder.
|
|
func (iuo *ItemUpdateOne) Mutation() *ItemMutation {
|
|
return iuo.mutation
|
|
}
|
|
|
|
// ClearParent clears the "parent" edge to the Item entity.
|
|
func (iuo *ItemUpdateOne) ClearParent() *ItemUpdateOne {
|
|
iuo.mutation.ClearParent()
|
|
return iuo
|
|
}
|
|
|
|
// ClearChildren clears all "children" edges to the Item entity.
|
|
func (iuo *ItemUpdateOne) ClearChildren() *ItemUpdateOne {
|
|
iuo.mutation.ClearChildren()
|
|
return iuo
|
|
}
|
|
|
|
// RemoveChildIDs removes the "children" edge to Item entities by IDs.
|
|
func (iuo *ItemUpdateOne) RemoveChildIDs(ids ...uuid.UUID) *ItemUpdateOne {
|
|
iuo.mutation.RemoveChildIDs(ids...)
|
|
return iuo
|
|
}
|
|
|
|
// RemoveChildren removes "children" edges to Item entities.
|
|
func (iuo *ItemUpdateOne) RemoveChildren(i ...*Item) *ItemUpdateOne {
|
|
ids := make([]uuid.UUID, len(i))
|
|
for j := range i {
|
|
ids[j] = i[j].ID
|
|
}
|
|
return iuo.RemoveChildIDs(ids...)
|
|
}
|
|
|
|
// ClearGroup clears the "group" edge to the Group entity.
|
|
func (iuo *ItemUpdateOne) ClearGroup() *ItemUpdateOne {
|
|
iuo.mutation.ClearGroup()
|
|
return iuo
|
|
}
|
|
|
|
// ClearLabel clears all "label" edges to the Label entity.
|
|
func (iuo *ItemUpdateOne) ClearLabel() *ItemUpdateOne {
|
|
iuo.mutation.ClearLabel()
|
|
return iuo
|
|
}
|
|
|
|
// RemoveLabelIDs removes the "label" edge to Label entities by IDs.
|
|
func (iuo *ItemUpdateOne) RemoveLabelIDs(ids ...uuid.UUID) *ItemUpdateOne {
|
|
iuo.mutation.RemoveLabelIDs(ids...)
|
|
return iuo
|
|
}
|
|
|
|
// RemoveLabel removes "label" edges to Label entities.
|
|
func (iuo *ItemUpdateOne) RemoveLabel(l ...*Label) *ItemUpdateOne {
|
|
ids := make([]uuid.UUID, len(l))
|
|
for i := range l {
|
|
ids[i] = l[i].ID
|
|
}
|
|
return iuo.RemoveLabelIDs(ids...)
|
|
}
|
|
|
|
// ClearLocation clears the "location" edge to the Location entity.
|
|
func (iuo *ItemUpdateOne) ClearLocation() *ItemUpdateOne {
|
|
iuo.mutation.ClearLocation()
|
|
return iuo
|
|
}
|
|
|
|
// ClearFields clears all "fields" edges to the ItemField entity.
|
|
func (iuo *ItemUpdateOne) ClearFields() *ItemUpdateOne {
|
|
iuo.mutation.ClearFields()
|
|
return iuo
|
|
}
|
|
|
|
// RemoveFieldIDs removes the "fields" edge to ItemField entities by IDs.
|
|
func (iuo *ItemUpdateOne) RemoveFieldIDs(ids ...uuid.UUID) *ItemUpdateOne {
|
|
iuo.mutation.RemoveFieldIDs(ids...)
|
|
return iuo
|
|
}
|
|
|
|
// RemoveFields removes "fields" edges to ItemField entities.
|
|
func (iuo *ItemUpdateOne) RemoveFields(i ...*ItemField) *ItemUpdateOne {
|
|
ids := make([]uuid.UUID, len(i))
|
|
for j := range i {
|
|
ids[j] = i[j].ID
|
|
}
|
|
return iuo.RemoveFieldIDs(ids...)
|
|
}
|
|
|
|
// ClearAttachments clears all "attachments" edges to the Attachment entity.
|
|
func (iuo *ItemUpdateOne) ClearAttachments() *ItemUpdateOne {
|
|
iuo.mutation.ClearAttachments()
|
|
return iuo
|
|
}
|
|
|
|
// RemoveAttachmentIDs removes the "attachments" edge to Attachment entities by IDs.
|
|
func (iuo *ItemUpdateOne) RemoveAttachmentIDs(ids ...uuid.UUID) *ItemUpdateOne {
|
|
iuo.mutation.RemoveAttachmentIDs(ids...)
|
|
return iuo
|
|
}
|
|
|
|
// RemoveAttachments removes "attachments" edges to Attachment entities.
|
|
func (iuo *ItemUpdateOne) RemoveAttachments(a ...*Attachment) *ItemUpdateOne {
|
|
ids := make([]uuid.UUID, len(a))
|
|
for i := range a {
|
|
ids[i] = a[i].ID
|
|
}
|
|
return iuo.RemoveAttachmentIDs(ids...)
|
|
}
|
|
|
|
// Select allows selecting one or more fields (columns) of the returned entity.
|
|
// The default is selecting all fields defined in the entity schema.
|
|
func (iuo *ItemUpdateOne) Select(field string, fields ...string) *ItemUpdateOne {
|
|
iuo.fields = append([]string{field}, fields...)
|
|
return iuo
|
|
}
|
|
|
|
// Save executes the query and returns the updated Item entity.
|
|
func (iuo *ItemUpdateOne) Save(ctx context.Context) (*Item, error) {
|
|
var (
|
|
err error
|
|
node *Item
|
|
)
|
|
iuo.defaults()
|
|
if len(iuo.hooks) == 0 {
|
|
if err = iuo.check(); err != nil {
|
|
return nil, err
|
|
}
|
|
node, err = iuo.sqlSave(ctx)
|
|
} else {
|
|
var mut Mutator = MutateFunc(func(ctx context.Context, m Mutation) (Value, error) {
|
|
mutation, ok := m.(*ItemMutation)
|
|
if !ok {
|
|
return nil, fmt.Errorf("unexpected mutation type %T", m)
|
|
}
|
|
if err = iuo.check(); err != nil {
|
|
return nil, err
|
|
}
|
|
iuo.mutation = mutation
|
|
node, err = iuo.sqlSave(ctx)
|
|
mutation.done = true
|
|
return node, err
|
|
})
|
|
for i := len(iuo.hooks) - 1; i >= 0; i-- {
|
|
if iuo.hooks[i] == nil {
|
|
return nil, fmt.Errorf("ent: uninitialized hook (forgotten import ent/runtime?)")
|
|
}
|
|
mut = iuo.hooks[i](mut)
|
|
}
|
|
v, err := mut.Mutate(ctx, iuo.mutation)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
nv, ok := v.(*Item)
|
|
if !ok {
|
|
return nil, fmt.Errorf("unexpected node type %T returned from ItemMutation", v)
|
|
}
|
|
node = nv
|
|
}
|
|
return node, err
|
|
}
|
|
|
|
// SaveX is like Save, but panics if an error occurs.
|
|
func (iuo *ItemUpdateOne) SaveX(ctx context.Context) *Item {
|
|
node, err := iuo.Save(ctx)
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
return node
|
|
}
|
|
|
|
// Exec executes the query on the entity.
|
|
func (iuo *ItemUpdateOne) Exec(ctx context.Context) error {
|
|
_, err := iuo.Save(ctx)
|
|
return err
|
|
}
|
|
|
|
// ExecX is like Exec, but panics if an error occurs.
|
|
func (iuo *ItemUpdateOne) ExecX(ctx context.Context) {
|
|
if err := iuo.Exec(ctx); err != nil {
|
|
panic(err)
|
|
}
|
|
}
|
|
|
|
// defaults sets the default values of the builder before save.
|
|
func (iuo *ItemUpdateOne) defaults() {
|
|
if _, ok := iuo.mutation.UpdatedAt(); !ok {
|
|
v := item.UpdateDefaultUpdatedAt()
|
|
iuo.mutation.SetUpdatedAt(v)
|
|
}
|
|
}
|
|
|
|
// check runs all checks and user-defined validators on the builder.
|
|
func (iuo *ItemUpdateOne) check() error {
|
|
if v, ok := iuo.mutation.Name(); ok {
|
|
if err := item.NameValidator(v); err != nil {
|
|
return &ValidationError{Name: "name", err: fmt.Errorf(`ent: validator failed for field "Item.name": %w`, err)}
|
|
}
|
|
}
|
|
if v, ok := iuo.mutation.Description(); ok {
|
|
if err := item.DescriptionValidator(v); err != nil {
|
|
return &ValidationError{Name: "description", err: fmt.Errorf(`ent: validator failed for field "Item.description": %w`, err)}
|
|
}
|
|
}
|
|
if v, ok := iuo.mutation.Notes(); ok {
|
|
if err := item.NotesValidator(v); err != nil {
|
|
return &ValidationError{Name: "notes", err: fmt.Errorf(`ent: validator failed for field "Item.notes": %w`, err)}
|
|
}
|
|
}
|
|
if v, ok := iuo.mutation.SerialNumber(); ok {
|
|
if err := item.SerialNumberValidator(v); err != nil {
|
|
return &ValidationError{Name: "serial_number", err: fmt.Errorf(`ent: validator failed for field "Item.serial_number": %w`, err)}
|
|
}
|
|
}
|
|
if v, ok := iuo.mutation.ModelNumber(); ok {
|
|
if err := item.ModelNumberValidator(v); err != nil {
|
|
return &ValidationError{Name: "model_number", err: fmt.Errorf(`ent: validator failed for field "Item.model_number": %w`, err)}
|
|
}
|
|
}
|
|
if v, ok := iuo.mutation.Manufacturer(); ok {
|
|
if err := item.ManufacturerValidator(v); err != nil {
|
|
return &ValidationError{Name: "manufacturer", err: fmt.Errorf(`ent: validator failed for field "Item.manufacturer": %w`, err)}
|
|
}
|
|
}
|
|
if v, ok := iuo.mutation.WarrantyDetails(); ok {
|
|
if err := item.WarrantyDetailsValidator(v); err != nil {
|
|
return &ValidationError{Name: "warranty_details", err: fmt.Errorf(`ent: validator failed for field "Item.warranty_details": %w`, err)}
|
|
}
|
|
}
|
|
if v, ok := iuo.mutation.SoldNotes(); ok {
|
|
if err := item.SoldNotesValidator(v); err != nil {
|
|
return &ValidationError{Name: "sold_notes", err: fmt.Errorf(`ent: validator failed for field "Item.sold_notes": %w`, err)}
|
|
}
|
|
}
|
|
if _, ok := iuo.mutation.GroupID(); iuo.mutation.GroupCleared() && !ok {
|
|
return errors.New(`ent: clearing a required unique edge "Item.group"`)
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (iuo *ItemUpdateOne) sqlSave(ctx context.Context) (_node *Item, err error) {
|
|
_spec := &sqlgraph.UpdateSpec{
|
|
Node: &sqlgraph.NodeSpec{
|
|
Table: item.Table,
|
|
Columns: item.Columns,
|
|
ID: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: item.FieldID,
|
|
},
|
|
},
|
|
}
|
|
id, ok := iuo.mutation.ID()
|
|
if !ok {
|
|
return nil, &ValidationError{Name: "id", err: errors.New(`ent: missing "Item.id" for update`)}
|
|
}
|
|
_spec.Node.ID.Value = id
|
|
if fields := iuo.fields; len(fields) > 0 {
|
|
_spec.Node.Columns = make([]string, 0, len(fields))
|
|
_spec.Node.Columns = append(_spec.Node.Columns, item.FieldID)
|
|
for _, f := range fields {
|
|
if !item.ValidColumn(f) {
|
|
return nil, &ValidationError{Name: f, err: fmt.Errorf("ent: invalid field %q for query", f)}
|
|
}
|
|
if f != item.FieldID {
|
|
_spec.Node.Columns = append(_spec.Node.Columns, f)
|
|
}
|
|
}
|
|
}
|
|
if ps := iuo.mutation.predicates; len(ps) > 0 {
|
|
_spec.Predicate = func(selector *sql.Selector) {
|
|
for i := range ps {
|
|
ps[i](selector)
|
|
}
|
|
}
|
|
}
|
|
if value, ok := iuo.mutation.UpdatedAt(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeTime,
|
|
Value: value,
|
|
Column: item.FieldUpdatedAt,
|
|
})
|
|
}
|
|
if value, ok := iuo.mutation.Name(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Value: value,
|
|
Column: item.FieldName,
|
|
})
|
|
}
|
|
if value, ok := iuo.mutation.Description(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Value: value,
|
|
Column: item.FieldDescription,
|
|
})
|
|
}
|
|
if iuo.mutation.DescriptionCleared() {
|
|
_spec.Fields.Clear = append(_spec.Fields.Clear, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Column: item.FieldDescription,
|
|
})
|
|
}
|
|
if iuo.mutation.ImportRefCleared() {
|
|
_spec.Fields.Clear = append(_spec.Fields.Clear, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Column: item.FieldImportRef,
|
|
})
|
|
}
|
|
if value, ok := iuo.mutation.Notes(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Value: value,
|
|
Column: item.FieldNotes,
|
|
})
|
|
}
|
|
if iuo.mutation.NotesCleared() {
|
|
_spec.Fields.Clear = append(_spec.Fields.Clear, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Column: item.FieldNotes,
|
|
})
|
|
}
|
|
if value, ok := iuo.mutation.Quantity(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeInt,
|
|
Value: value,
|
|
Column: item.FieldQuantity,
|
|
})
|
|
}
|
|
if value, ok := iuo.mutation.AddedQuantity(); ok {
|
|
_spec.Fields.Add = append(_spec.Fields.Add, &sqlgraph.FieldSpec{
|
|
Type: field.TypeInt,
|
|
Value: value,
|
|
Column: item.FieldQuantity,
|
|
})
|
|
}
|
|
if value, ok := iuo.mutation.Insured(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeBool,
|
|
Value: value,
|
|
Column: item.FieldInsured,
|
|
})
|
|
}
|
|
if value, ok := iuo.mutation.Archived(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeBool,
|
|
Value: value,
|
|
Column: item.FieldArchived,
|
|
})
|
|
}
|
|
if value, ok := iuo.mutation.SerialNumber(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Value: value,
|
|
Column: item.FieldSerialNumber,
|
|
})
|
|
}
|
|
if iuo.mutation.SerialNumberCleared() {
|
|
_spec.Fields.Clear = append(_spec.Fields.Clear, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Column: item.FieldSerialNumber,
|
|
})
|
|
}
|
|
if value, ok := iuo.mutation.ModelNumber(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Value: value,
|
|
Column: item.FieldModelNumber,
|
|
})
|
|
}
|
|
if iuo.mutation.ModelNumberCleared() {
|
|
_spec.Fields.Clear = append(_spec.Fields.Clear, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Column: item.FieldModelNumber,
|
|
})
|
|
}
|
|
if value, ok := iuo.mutation.Manufacturer(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Value: value,
|
|
Column: item.FieldManufacturer,
|
|
})
|
|
}
|
|
if iuo.mutation.ManufacturerCleared() {
|
|
_spec.Fields.Clear = append(_spec.Fields.Clear, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Column: item.FieldManufacturer,
|
|
})
|
|
}
|
|
if value, ok := iuo.mutation.LifetimeWarranty(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeBool,
|
|
Value: value,
|
|
Column: item.FieldLifetimeWarranty,
|
|
})
|
|
}
|
|
if value, ok := iuo.mutation.WarrantyExpires(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeTime,
|
|
Value: value,
|
|
Column: item.FieldWarrantyExpires,
|
|
})
|
|
}
|
|
if iuo.mutation.WarrantyExpiresCleared() {
|
|
_spec.Fields.Clear = append(_spec.Fields.Clear, &sqlgraph.FieldSpec{
|
|
Type: field.TypeTime,
|
|
Column: item.FieldWarrantyExpires,
|
|
})
|
|
}
|
|
if value, ok := iuo.mutation.WarrantyDetails(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Value: value,
|
|
Column: item.FieldWarrantyDetails,
|
|
})
|
|
}
|
|
if iuo.mutation.WarrantyDetailsCleared() {
|
|
_spec.Fields.Clear = append(_spec.Fields.Clear, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Column: item.FieldWarrantyDetails,
|
|
})
|
|
}
|
|
if value, ok := iuo.mutation.PurchaseTime(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeTime,
|
|
Value: value,
|
|
Column: item.FieldPurchaseTime,
|
|
})
|
|
}
|
|
if iuo.mutation.PurchaseTimeCleared() {
|
|
_spec.Fields.Clear = append(_spec.Fields.Clear, &sqlgraph.FieldSpec{
|
|
Type: field.TypeTime,
|
|
Column: item.FieldPurchaseTime,
|
|
})
|
|
}
|
|
if value, ok := iuo.mutation.PurchaseFrom(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Value: value,
|
|
Column: item.FieldPurchaseFrom,
|
|
})
|
|
}
|
|
if iuo.mutation.PurchaseFromCleared() {
|
|
_spec.Fields.Clear = append(_spec.Fields.Clear, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Column: item.FieldPurchaseFrom,
|
|
})
|
|
}
|
|
if value, ok := iuo.mutation.PurchasePrice(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeFloat64,
|
|
Value: value,
|
|
Column: item.FieldPurchasePrice,
|
|
})
|
|
}
|
|
if value, ok := iuo.mutation.AddedPurchasePrice(); ok {
|
|
_spec.Fields.Add = append(_spec.Fields.Add, &sqlgraph.FieldSpec{
|
|
Type: field.TypeFloat64,
|
|
Value: value,
|
|
Column: item.FieldPurchasePrice,
|
|
})
|
|
}
|
|
if value, ok := iuo.mutation.SoldTime(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeTime,
|
|
Value: value,
|
|
Column: item.FieldSoldTime,
|
|
})
|
|
}
|
|
if iuo.mutation.SoldTimeCleared() {
|
|
_spec.Fields.Clear = append(_spec.Fields.Clear, &sqlgraph.FieldSpec{
|
|
Type: field.TypeTime,
|
|
Column: item.FieldSoldTime,
|
|
})
|
|
}
|
|
if value, ok := iuo.mutation.SoldTo(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Value: value,
|
|
Column: item.FieldSoldTo,
|
|
})
|
|
}
|
|
if iuo.mutation.SoldToCleared() {
|
|
_spec.Fields.Clear = append(_spec.Fields.Clear, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Column: item.FieldSoldTo,
|
|
})
|
|
}
|
|
if value, ok := iuo.mutation.SoldPrice(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeFloat64,
|
|
Value: value,
|
|
Column: item.FieldSoldPrice,
|
|
})
|
|
}
|
|
if value, ok := iuo.mutation.AddedSoldPrice(); ok {
|
|
_spec.Fields.Add = append(_spec.Fields.Add, &sqlgraph.FieldSpec{
|
|
Type: field.TypeFloat64,
|
|
Value: value,
|
|
Column: item.FieldSoldPrice,
|
|
})
|
|
}
|
|
if value, ok := iuo.mutation.SoldNotes(); ok {
|
|
_spec.Fields.Set = append(_spec.Fields.Set, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Value: value,
|
|
Column: item.FieldSoldNotes,
|
|
})
|
|
}
|
|
if iuo.mutation.SoldNotesCleared() {
|
|
_spec.Fields.Clear = append(_spec.Fields.Clear, &sqlgraph.FieldSpec{
|
|
Type: field.TypeString,
|
|
Column: item.FieldSoldNotes,
|
|
})
|
|
}
|
|
if iuo.mutation.ParentCleared() {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.M2O,
|
|
Inverse: true,
|
|
Table: item.ParentTable,
|
|
Columns: []string{item.ParentColumn},
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: item.FieldID,
|
|
},
|
|
},
|
|
}
|
|
_spec.Edges.Clear = append(_spec.Edges.Clear, edge)
|
|
}
|
|
if nodes := iuo.mutation.ParentIDs(); len(nodes) > 0 {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.M2O,
|
|
Inverse: true,
|
|
Table: item.ParentTable,
|
|
Columns: []string{item.ParentColumn},
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: item.FieldID,
|
|
},
|
|
},
|
|
}
|
|
for _, k := range nodes {
|
|
edge.Target.Nodes = append(edge.Target.Nodes, k)
|
|
}
|
|
_spec.Edges.Add = append(_spec.Edges.Add, edge)
|
|
}
|
|
if iuo.mutation.ChildrenCleared() {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.O2M,
|
|
Inverse: false,
|
|
Table: item.ChildrenTable,
|
|
Columns: []string{item.ChildrenColumn},
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: item.FieldID,
|
|
},
|
|
},
|
|
}
|
|
_spec.Edges.Clear = append(_spec.Edges.Clear, edge)
|
|
}
|
|
if nodes := iuo.mutation.RemovedChildrenIDs(); len(nodes) > 0 && !iuo.mutation.ChildrenCleared() {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.O2M,
|
|
Inverse: false,
|
|
Table: item.ChildrenTable,
|
|
Columns: []string{item.ChildrenColumn},
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: item.FieldID,
|
|
},
|
|
},
|
|
}
|
|
for _, k := range nodes {
|
|
edge.Target.Nodes = append(edge.Target.Nodes, k)
|
|
}
|
|
_spec.Edges.Clear = append(_spec.Edges.Clear, edge)
|
|
}
|
|
if nodes := iuo.mutation.ChildrenIDs(); len(nodes) > 0 {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.O2M,
|
|
Inverse: false,
|
|
Table: item.ChildrenTable,
|
|
Columns: []string{item.ChildrenColumn},
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: item.FieldID,
|
|
},
|
|
},
|
|
}
|
|
for _, k := range nodes {
|
|
edge.Target.Nodes = append(edge.Target.Nodes, k)
|
|
}
|
|
_spec.Edges.Add = append(_spec.Edges.Add, edge)
|
|
}
|
|
if iuo.mutation.GroupCleared() {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.M2O,
|
|
Inverse: true,
|
|
Table: item.GroupTable,
|
|
Columns: []string{item.GroupColumn},
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: group.FieldID,
|
|
},
|
|
},
|
|
}
|
|
_spec.Edges.Clear = append(_spec.Edges.Clear, edge)
|
|
}
|
|
if nodes := iuo.mutation.GroupIDs(); len(nodes) > 0 {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.M2O,
|
|
Inverse: true,
|
|
Table: item.GroupTable,
|
|
Columns: []string{item.GroupColumn},
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: group.FieldID,
|
|
},
|
|
},
|
|
}
|
|
for _, k := range nodes {
|
|
edge.Target.Nodes = append(edge.Target.Nodes, k)
|
|
}
|
|
_spec.Edges.Add = append(_spec.Edges.Add, edge)
|
|
}
|
|
if iuo.mutation.LabelCleared() {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.M2M,
|
|
Inverse: true,
|
|
Table: item.LabelTable,
|
|
Columns: item.LabelPrimaryKey,
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: label.FieldID,
|
|
},
|
|
},
|
|
}
|
|
_spec.Edges.Clear = append(_spec.Edges.Clear, edge)
|
|
}
|
|
if nodes := iuo.mutation.RemovedLabelIDs(); len(nodes) > 0 && !iuo.mutation.LabelCleared() {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.M2M,
|
|
Inverse: true,
|
|
Table: item.LabelTable,
|
|
Columns: item.LabelPrimaryKey,
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: label.FieldID,
|
|
},
|
|
},
|
|
}
|
|
for _, k := range nodes {
|
|
edge.Target.Nodes = append(edge.Target.Nodes, k)
|
|
}
|
|
_spec.Edges.Clear = append(_spec.Edges.Clear, edge)
|
|
}
|
|
if nodes := iuo.mutation.LabelIDs(); len(nodes) > 0 {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.M2M,
|
|
Inverse: true,
|
|
Table: item.LabelTable,
|
|
Columns: item.LabelPrimaryKey,
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: label.FieldID,
|
|
},
|
|
},
|
|
}
|
|
for _, k := range nodes {
|
|
edge.Target.Nodes = append(edge.Target.Nodes, k)
|
|
}
|
|
_spec.Edges.Add = append(_spec.Edges.Add, edge)
|
|
}
|
|
if iuo.mutation.LocationCleared() {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.M2O,
|
|
Inverse: true,
|
|
Table: item.LocationTable,
|
|
Columns: []string{item.LocationColumn},
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: location.FieldID,
|
|
},
|
|
},
|
|
}
|
|
_spec.Edges.Clear = append(_spec.Edges.Clear, edge)
|
|
}
|
|
if nodes := iuo.mutation.LocationIDs(); len(nodes) > 0 {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.M2O,
|
|
Inverse: true,
|
|
Table: item.LocationTable,
|
|
Columns: []string{item.LocationColumn},
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: location.FieldID,
|
|
},
|
|
},
|
|
}
|
|
for _, k := range nodes {
|
|
edge.Target.Nodes = append(edge.Target.Nodes, k)
|
|
}
|
|
_spec.Edges.Add = append(_spec.Edges.Add, edge)
|
|
}
|
|
if iuo.mutation.FieldsCleared() {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.O2M,
|
|
Inverse: false,
|
|
Table: item.FieldsTable,
|
|
Columns: []string{item.FieldsColumn},
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: itemfield.FieldID,
|
|
},
|
|
},
|
|
}
|
|
_spec.Edges.Clear = append(_spec.Edges.Clear, edge)
|
|
}
|
|
if nodes := iuo.mutation.RemovedFieldsIDs(); len(nodes) > 0 && !iuo.mutation.FieldsCleared() {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.O2M,
|
|
Inverse: false,
|
|
Table: item.FieldsTable,
|
|
Columns: []string{item.FieldsColumn},
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: itemfield.FieldID,
|
|
},
|
|
},
|
|
}
|
|
for _, k := range nodes {
|
|
edge.Target.Nodes = append(edge.Target.Nodes, k)
|
|
}
|
|
_spec.Edges.Clear = append(_spec.Edges.Clear, edge)
|
|
}
|
|
if nodes := iuo.mutation.FieldsIDs(); len(nodes) > 0 {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.O2M,
|
|
Inverse: false,
|
|
Table: item.FieldsTable,
|
|
Columns: []string{item.FieldsColumn},
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: itemfield.FieldID,
|
|
},
|
|
},
|
|
}
|
|
for _, k := range nodes {
|
|
edge.Target.Nodes = append(edge.Target.Nodes, k)
|
|
}
|
|
_spec.Edges.Add = append(_spec.Edges.Add, edge)
|
|
}
|
|
if iuo.mutation.AttachmentsCleared() {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.O2M,
|
|
Inverse: false,
|
|
Table: item.AttachmentsTable,
|
|
Columns: []string{item.AttachmentsColumn},
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: attachment.FieldID,
|
|
},
|
|
},
|
|
}
|
|
_spec.Edges.Clear = append(_spec.Edges.Clear, edge)
|
|
}
|
|
if nodes := iuo.mutation.RemovedAttachmentsIDs(); len(nodes) > 0 && !iuo.mutation.AttachmentsCleared() {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.O2M,
|
|
Inverse: false,
|
|
Table: item.AttachmentsTable,
|
|
Columns: []string{item.AttachmentsColumn},
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: attachment.FieldID,
|
|
},
|
|
},
|
|
}
|
|
for _, k := range nodes {
|
|
edge.Target.Nodes = append(edge.Target.Nodes, k)
|
|
}
|
|
_spec.Edges.Clear = append(_spec.Edges.Clear, edge)
|
|
}
|
|
if nodes := iuo.mutation.AttachmentsIDs(); len(nodes) > 0 {
|
|
edge := &sqlgraph.EdgeSpec{
|
|
Rel: sqlgraph.O2M,
|
|
Inverse: false,
|
|
Table: item.AttachmentsTable,
|
|
Columns: []string{item.AttachmentsColumn},
|
|
Bidi: false,
|
|
Target: &sqlgraph.EdgeTarget{
|
|
IDSpec: &sqlgraph.FieldSpec{
|
|
Type: field.TypeUUID,
|
|
Column: attachment.FieldID,
|
|
},
|
|
},
|
|
}
|
|
for _, k := range nodes {
|
|
edge.Target.Nodes = append(edge.Target.Nodes, k)
|
|
}
|
|
_spec.Edges.Add = append(_spec.Edges.Add, edge)
|
|
}
|
|
_node = &Item{config: iuo.config}
|
|
_spec.Assign = _node.assignValues
|
|
_spec.ScanValues = _node.scanValues
|
|
if err = sqlgraph.UpdateNode(ctx, iuo.driver, _spec); err != nil {
|
|
if _, ok := err.(*sqlgraph.NotFoundError); ok {
|
|
err = &NotFoundError{item.Label}
|
|
} else if sqlgraph.IsConstraintError(err) {
|
|
err = &ConstraintError{msg: err.Error(), wrap: err}
|
|
}
|
|
return nil, err
|
|
}
|
|
return _node, nil
|
|
}
|