mirror of
https://github.com/hay-kot/homebox.git
synced 2024-11-16 21:58:40 +00:00
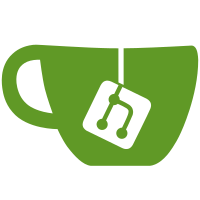
* format readme * update logo * format html * add logo to docs * repository for document and document tokens * add attachments type and repository * autogenerate types via scripts * use autogenerated types * attachment type updates * add insured and quantity fields for items * implement HasID interface for entities * implement label updates for items * implement service update method * WIP item update client side actions * check err on attachment * finish types for basic items editor * remove unused var * house keeping
98 lines
3.8 KiB
Go
98 lines
3.8 KiB
Go
// Code generated by ent, DO NOT EDIT.
|
|
|
|
package document
|
|
|
|
import (
|
|
"time"
|
|
|
|
"github.com/google/uuid"
|
|
)
|
|
|
|
const (
|
|
// Label holds the string label denoting the document type in the database.
|
|
Label = "document"
|
|
// FieldID holds the string denoting the id field in the database.
|
|
FieldID = "id"
|
|
// FieldCreatedAt holds the string denoting the created_at field in the database.
|
|
FieldCreatedAt = "created_at"
|
|
// FieldUpdatedAt holds the string denoting the updated_at field in the database.
|
|
FieldUpdatedAt = "updated_at"
|
|
// FieldTitle holds the string denoting the title field in the database.
|
|
FieldTitle = "title"
|
|
// FieldPath holds the string denoting the path field in the database.
|
|
FieldPath = "path"
|
|
// EdgeGroup holds the string denoting the group edge name in mutations.
|
|
EdgeGroup = "group"
|
|
// EdgeDocumentTokens holds the string denoting the document_tokens edge name in mutations.
|
|
EdgeDocumentTokens = "document_tokens"
|
|
// EdgeAttachments holds the string denoting the attachments edge name in mutations.
|
|
EdgeAttachments = "attachments"
|
|
// Table holds the table name of the document in the database.
|
|
Table = "documents"
|
|
// GroupTable is the table that holds the group relation/edge.
|
|
GroupTable = "documents"
|
|
// GroupInverseTable is the table name for the Group entity.
|
|
// It exists in this package in order to avoid circular dependency with the "group" package.
|
|
GroupInverseTable = "groups"
|
|
// GroupColumn is the table column denoting the group relation/edge.
|
|
GroupColumn = "group_documents"
|
|
// DocumentTokensTable is the table that holds the document_tokens relation/edge.
|
|
DocumentTokensTable = "document_tokens"
|
|
// DocumentTokensInverseTable is the table name for the DocumentToken entity.
|
|
// It exists in this package in order to avoid circular dependency with the "documenttoken" package.
|
|
DocumentTokensInverseTable = "document_tokens"
|
|
// DocumentTokensColumn is the table column denoting the document_tokens relation/edge.
|
|
DocumentTokensColumn = "document_document_tokens"
|
|
// AttachmentsTable is the table that holds the attachments relation/edge.
|
|
AttachmentsTable = "attachments"
|
|
// AttachmentsInverseTable is the table name for the Attachment entity.
|
|
// It exists in this package in order to avoid circular dependency with the "attachment" package.
|
|
AttachmentsInverseTable = "attachments"
|
|
// AttachmentsColumn is the table column denoting the attachments relation/edge.
|
|
AttachmentsColumn = "document_attachments"
|
|
)
|
|
|
|
// Columns holds all SQL columns for document fields.
|
|
var Columns = []string{
|
|
FieldID,
|
|
FieldCreatedAt,
|
|
FieldUpdatedAt,
|
|
FieldTitle,
|
|
FieldPath,
|
|
}
|
|
|
|
// ForeignKeys holds the SQL foreign-keys that are owned by the "documents"
|
|
// table and are not defined as standalone fields in the schema.
|
|
var ForeignKeys = []string{
|
|
"group_documents",
|
|
}
|
|
|
|
// ValidColumn reports if the column name is valid (part of the table columns).
|
|
func ValidColumn(column string) bool {
|
|
for i := range Columns {
|
|
if column == Columns[i] {
|
|
return true
|
|
}
|
|
}
|
|
for i := range ForeignKeys {
|
|
if column == ForeignKeys[i] {
|
|
return true
|
|
}
|
|
}
|
|
return false
|
|
}
|
|
|
|
var (
|
|
// DefaultCreatedAt holds the default value on creation for the "created_at" field.
|
|
DefaultCreatedAt func() time.Time
|
|
// DefaultUpdatedAt holds the default value on creation for the "updated_at" field.
|
|
DefaultUpdatedAt func() time.Time
|
|
// UpdateDefaultUpdatedAt holds the default value on update for the "updated_at" field.
|
|
UpdateDefaultUpdatedAt func() time.Time
|
|
// TitleValidator is a validator for the "title" field. It is called by the builders before save.
|
|
TitleValidator func(string) error
|
|
// PathValidator is a validator for the "path" field. It is called by the builders before save.
|
|
PathValidator func(string) error
|
|
// DefaultID holds the default value on creation for the "id" field.
|
|
DefaultID func() uuid.UUID
|
|
)
|