mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-10-31 16:38:12 +00:00
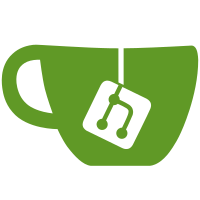
Change the asm/unistd.h header for arm64 to no longer include asm-generic/unistd.h itself, but instead generate both the asm/unistd.h contents and the list of entry points using the syscall.tbl scripts that we use on most other architectures. Once his is done for the remaining architectures, the generic unistd.h header can be removed and the generated tbl file put in its place. The Makefile changes are more complex than they should be, I need a little help to improve those. Ideally this should be done in an architecture-independent way as well. Acked-by: Catalin Marinas <catalin.marinas@arm.com> Signed-off-by: Arnd Bergmann <arnd@arndb.de>
63 lines
1.6 KiB
C
63 lines
1.6 KiB
C
// SPDX-License-Identifier: GPL-2.0-only
|
|
/*
|
|
* AArch64-specific system calls implementation
|
|
*
|
|
* Copyright (C) 2012 ARM Ltd.
|
|
* Author: Catalin Marinas <catalin.marinas@arm.com>
|
|
*/
|
|
|
|
#include <linux/compiler.h>
|
|
#include <linux/errno.h>
|
|
#include <linux/fs.h>
|
|
#include <linux/mm.h>
|
|
#include <linux/export.h>
|
|
#include <linux/sched.h>
|
|
#include <linux/slab.h>
|
|
#include <linux/syscalls.h>
|
|
|
|
#include <asm/cpufeature.h>
|
|
#include <asm/syscall.h>
|
|
|
|
SYSCALL_DEFINE6(mmap, unsigned long, addr, unsigned long, len,
|
|
unsigned long, prot, unsigned long, flags,
|
|
unsigned long, fd, unsigned long, off)
|
|
{
|
|
if (offset_in_page(off) != 0)
|
|
return -EINVAL;
|
|
|
|
return ksys_mmap_pgoff(addr, len, prot, flags, fd, off >> PAGE_SHIFT);
|
|
}
|
|
|
|
SYSCALL_DEFINE1(arm64_personality, unsigned int, personality)
|
|
{
|
|
if (personality(personality) == PER_LINUX32 &&
|
|
!system_supports_32bit_el0())
|
|
return -EINVAL;
|
|
return ksys_personality(personality);
|
|
}
|
|
|
|
asmlinkage long sys_ni_syscall(void);
|
|
|
|
asmlinkage long __arm64_sys_ni_syscall(const struct pt_regs *__unused)
|
|
{
|
|
return sys_ni_syscall();
|
|
}
|
|
|
|
/*
|
|
* Wrappers to pass the pt_regs argument.
|
|
*/
|
|
#define __arm64_sys_personality __arm64_sys_arm64_personality
|
|
|
|
#define __SYSCALL_WITH_COMPAT(nr, native, compat) __SYSCALL(nr, native)
|
|
|
|
#undef __SYSCALL
|
|
#define __SYSCALL(nr, sym) asmlinkage long __arm64_##sym(const struct pt_regs *);
|
|
#include <asm/syscall_table_64.h>
|
|
|
|
#undef __SYSCALL
|
|
#define __SYSCALL(nr, sym) [nr] = __arm64_##sym,
|
|
|
|
const syscall_fn_t sys_call_table[__NR_syscalls] = {
|
|
[0 ... __NR_syscalls - 1] = __arm64_sys_ni_syscall,
|
|
#include <asm/syscall_table_64.h>
|
|
};
|