mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-21 00:10:09 +00:00
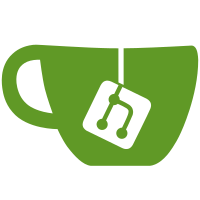
To deal with forwarding the strerror_r (GNU) return we need to check if the returned value is the buffer we passed or maybe some constant (unknown error), simplify that action by using scnprintf, that will do all the buflen size checks, trimming if needed. Acked-by: Jiri Olsa <jolsa@redhat.com> Acked-by: Namhyung Kim <namhyung@kernel.org> Cc: Adrian Hunter <adrian.hunter@intel.com> Cc: Borislav Petkov <bp@suse.de> Cc: David Ahern <dsahern@gmail.com> Cc: Don Zickus <dzickus@redhat.com> Cc: Frederic Weisbecker <fweisbec@gmail.com> Cc: Stephane Eranian <eranian@google.com> Link: http://lkml.kernel.org/n/tip-d0ik6i5gjew56j0qphql28ou@git.kernel.org Signed-off-by: Arnaldo Carvalho de Melo <acme@redhat.com>
155 lines
3.4 KiB
C
155 lines
3.4 KiB
C
/*
|
|
* Helper functions for handling target threads/cpus
|
|
*
|
|
* Copyright (C) 2012, LG Electronics, Namhyung Kim <namhyung.kim@lge.com>
|
|
*
|
|
* Released under the GPL v2.
|
|
*/
|
|
|
|
#include "target.h"
|
|
#include "debug.h"
|
|
|
|
#include <pwd.h>
|
|
#include <string.h>
|
|
|
|
|
|
enum target_errno target__validate(struct target *target)
|
|
{
|
|
enum target_errno ret = TARGET_ERRNO__SUCCESS;
|
|
|
|
if (target->pid)
|
|
target->tid = target->pid;
|
|
|
|
/* CPU and PID are mutually exclusive */
|
|
if (target->tid && target->cpu_list) {
|
|
target->cpu_list = NULL;
|
|
if (ret == TARGET_ERRNO__SUCCESS)
|
|
ret = TARGET_ERRNO__PID_OVERRIDE_CPU;
|
|
}
|
|
|
|
/* UID and PID are mutually exclusive */
|
|
if (target->tid && target->uid_str) {
|
|
target->uid_str = NULL;
|
|
if (ret == TARGET_ERRNO__SUCCESS)
|
|
ret = TARGET_ERRNO__PID_OVERRIDE_UID;
|
|
}
|
|
|
|
/* UID and CPU are mutually exclusive */
|
|
if (target->uid_str && target->cpu_list) {
|
|
target->cpu_list = NULL;
|
|
if (ret == TARGET_ERRNO__SUCCESS)
|
|
ret = TARGET_ERRNO__UID_OVERRIDE_CPU;
|
|
}
|
|
|
|
/* PID and SYSTEM are mutually exclusive */
|
|
if (target->tid && target->system_wide) {
|
|
target->system_wide = false;
|
|
if (ret == TARGET_ERRNO__SUCCESS)
|
|
ret = TARGET_ERRNO__PID_OVERRIDE_SYSTEM;
|
|
}
|
|
|
|
/* UID and SYSTEM are mutually exclusive */
|
|
if (target->uid_str && target->system_wide) {
|
|
target->system_wide = false;
|
|
if (ret == TARGET_ERRNO__SUCCESS)
|
|
ret = TARGET_ERRNO__UID_OVERRIDE_SYSTEM;
|
|
}
|
|
|
|
/* THREAD and SYSTEM/CPU are mutually exclusive */
|
|
if (target->per_thread && (target->system_wide || target->cpu_list)) {
|
|
target->per_thread = false;
|
|
if (ret == TARGET_ERRNO__SUCCESS)
|
|
ret = TARGET_ERRNO__SYSTEM_OVERRIDE_THREAD;
|
|
}
|
|
|
|
return ret;
|
|
}
|
|
|
|
enum target_errno target__parse_uid(struct target *target)
|
|
{
|
|
struct passwd pwd, *result;
|
|
char buf[1024];
|
|
const char *str = target->uid_str;
|
|
|
|
target->uid = UINT_MAX;
|
|
if (str == NULL)
|
|
return TARGET_ERRNO__SUCCESS;
|
|
|
|
/* Try user name first */
|
|
getpwnam_r(str, &pwd, buf, sizeof(buf), &result);
|
|
|
|
if (result == NULL) {
|
|
/*
|
|
* The user name not found. Maybe it's a UID number.
|
|
*/
|
|
char *endptr;
|
|
int uid = strtol(str, &endptr, 10);
|
|
|
|
if (*endptr != '\0')
|
|
return TARGET_ERRNO__INVALID_UID;
|
|
|
|
getpwuid_r(uid, &pwd, buf, sizeof(buf), &result);
|
|
|
|
if (result == NULL)
|
|
return TARGET_ERRNO__USER_NOT_FOUND;
|
|
}
|
|
|
|
target->uid = result->pw_uid;
|
|
return TARGET_ERRNO__SUCCESS;
|
|
}
|
|
|
|
/*
|
|
* This must have a same ordering as the enum target_errno.
|
|
*/
|
|
static const char *target__error_str[] = {
|
|
"PID/TID switch overriding CPU",
|
|
"PID/TID switch overriding UID",
|
|
"UID switch overriding CPU",
|
|
"PID/TID switch overriding SYSTEM",
|
|
"UID switch overriding SYSTEM",
|
|
"SYSTEM/CPU switch overriding PER-THREAD",
|
|
"Invalid User: %s",
|
|
"Problems obtaining information for user %s",
|
|
};
|
|
|
|
int target__strerror(struct target *target, int errnum,
|
|
char *buf, size_t buflen)
|
|
{
|
|
int idx;
|
|
const char *msg;
|
|
|
|
BUG_ON(buflen == 0);
|
|
|
|
if (errnum >= 0) {
|
|
const char *err = strerror_r(errnum, buf, buflen);
|
|
|
|
if (err != buf)
|
|
scnprintf(buf, buflen, "%s", err);
|
|
|
|
return 0;
|
|
}
|
|
|
|
if (errnum < __TARGET_ERRNO__START || errnum >= __TARGET_ERRNO__END)
|
|
return -1;
|
|
|
|
idx = errnum - __TARGET_ERRNO__START;
|
|
msg = target__error_str[idx];
|
|
|
|
switch (errnum) {
|
|
case TARGET_ERRNO__PID_OVERRIDE_CPU ...
|
|
TARGET_ERRNO__SYSTEM_OVERRIDE_THREAD:
|
|
snprintf(buf, buflen, "%s", msg);
|
|
break;
|
|
|
|
case TARGET_ERRNO__INVALID_UID:
|
|
case TARGET_ERRNO__USER_NOT_FOUND:
|
|
snprintf(buf, buflen, msg, target->uid_str);
|
|
break;
|
|
|
|
default:
|
|
/* cannot reach here */
|
|
break;
|
|
}
|
|
|
|
return 0;
|
|
}
|