mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-05 18:39:59 +00:00
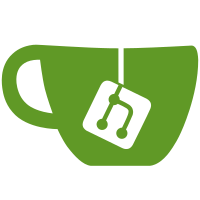
This code implements the transmitter which is currently implemented in the staging lirc_zilog driver. The new code does not need a signal database, iow. the haup-ir-blaster.bin firmware file is no longer needed, and the driver does not know anything about the keycodes in that file. Instead, the new driver can send raw IR, but the hardware is limited to few different lengths of pulse and spaces, so it is best to use generated IR rather than recorded IR. Signed-off-by: Sean Young <sean@mess.org> Signed-off-by: Mauro Carvalho Chehab <mchehab@s-opensource.com>
61 lines
1.5 KiB
C
61 lines
1.5 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef _IR_I2C
|
|
#define _IR_I2C
|
|
|
|
#include <media/rc-core.h>
|
|
|
|
#define DEFAULT_POLLING_INTERVAL 100 /* ms */
|
|
|
|
struct IR_i2c;
|
|
|
|
struct IR_i2c {
|
|
char *ir_codes;
|
|
struct i2c_client *c;
|
|
struct rc_dev *rc;
|
|
|
|
/* Used to avoid fast repeating */
|
|
unsigned char old;
|
|
|
|
u32 polling_interval; /* in ms */
|
|
|
|
struct delayed_work work;
|
|
char phys[32];
|
|
int (*get_key)(struct IR_i2c *ir,
|
|
enum rc_proto *protocol,
|
|
u32 *scancode, u8 *toggle);
|
|
/* tx */
|
|
struct i2c_client *tx_c;
|
|
struct mutex lock; /* do not poll Rx during Tx */
|
|
unsigned int carrier;
|
|
unsigned int duty_cycle;
|
|
};
|
|
|
|
enum ir_kbd_get_key_fn {
|
|
IR_KBD_GET_KEY_CUSTOM = 0,
|
|
IR_KBD_GET_KEY_PIXELVIEW,
|
|
IR_KBD_GET_KEY_HAUP,
|
|
IR_KBD_GET_KEY_KNC1,
|
|
IR_KBD_GET_KEY_FUSIONHDTV,
|
|
IR_KBD_GET_KEY_HAUP_XVR,
|
|
IR_KBD_GET_KEY_AVERMEDIA_CARDBUS,
|
|
};
|
|
|
|
/* Can be passed when instantiating an ir_video i2c device */
|
|
struct IR_i2c_init_data {
|
|
char *ir_codes;
|
|
const char *name;
|
|
u64 type; /* RC_PROTO_BIT_RC5, etc */
|
|
u32 polling_interval; /* 0 means DEFAULT_POLLING_INTERVAL */
|
|
|
|
/*
|
|
* Specify either a function pointer or a value indicating one of
|
|
* ir_kbd_i2c's internal get_key functions
|
|
*/
|
|
int (*get_key)(struct IR_i2c *ir,
|
|
enum rc_proto *protocol,
|
|
u32 *scancode, u8 *toggle);
|
|
enum ir_kbd_get_key_fn internal_get_key_func;
|
|
|
|
struct rc_dev *rc_dev;
|
|
};
|
|
#endif
|