mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-12 13:55:32 +00:00
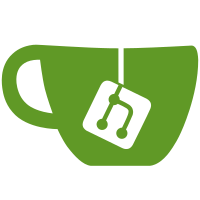
Since genpd at late init, will try to disable unused PM domains we don't need to do it from here as well. Signed-off-by: Ulf Hansson <ulf.hansson@linaro.org> Acked-by: Simon Horman <horms+renesas@verge.net.au> Reviewed-by: Kevin Hilman <khilman@linaro.org> Signed-off-by: Rafael J. Wysocki <rafael.j.wysocki@intel.com>
100 lines
2.4 KiB
C
100 lines
2.4 KiB
C
/*
|
|
* Runtime PM support code
|
|
*
|
|
* Copyright (C) 2009-2010 Magnus Damm
|
|
*
|
|
* This file is subject to the terms and conditions of the GNU General Public
|
|
* License. See the file "COPYING" in the main directory of this archive
|
|
* for more details.
|
|
*/
|
|
|
|
#include <linux/init.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/io.h>
|
|
#include <linux/pm_runtime.h>
|
|
#include <linux/pm_domain.h>
|
|
#include <linux/pm_clock.h>
|
|
#include <linux/platform_device.h>
|
|
#include <linux/clk.h>
|
|
#include <linux/sh_clk.h>
|
|
#include <linux/bitmap.h>
|
|
#include <linux/slab.h>
|
|
|
|
#ifdef CONFIG_PM_RUNTIME
|
|
static int sh_pm_runtime_suspend(struct device *dev)
|
|
{
|
|
int ret;
|
|
|
|
ret = pm_generic_runtime_suspend(dev);
|
|
if (ret) {
|
|
dev_err(dev, "failed to suspend device\n");
|
|
return ret;
|
|
}
|
|
|
|
ret = pm_clk_suspend(dev);
|
|
if (ret) {
|
|
dev_err(dev, "failed to suspend clock\n");
|
|
pm_generic_runtime_resume(dev);
|
|
return ret;
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int sh_pm_runtime_resume(struct device *dev)
|
|
{
|
|
int ret;
|
|
|
|
ret = pm_clk_resume(dev);
|
|
if (ret) {
|
|
dev_err(dev, "failed to resume clock\n");
|
|
return ret;
|
|
}
|
|
|
|
return pm_generic_runtime_resume(dev);
|
|
}
|
|
|
|
static struct dev_pm_domain default_pm_domain = {
|
|
.ops = {
|
|
.runtime_suspend = sh_pm_runtime_suspend,
|
|
.runtime_resume = sh_pm_runtime_resume,
|
|
USE_PLATFORM_PM_SLEEP_OPS
|
|
},
|
|
};
|
|
|
|
#define DEFAULT_PM_DOMAIN_PTR (&default_pm_domain)
|
|
|
|
#else
|
|
|
|
#define DEFAULT_PM_DOMAIN_PTR NULL
|
|
|
|
#endif /* CONFIG_PM_RUNTIME */
|
|
|
|
static struct pm_clk_notifier_block platform_bus_notifier = {
|
|
.pm_domain = DEFAULT_PM_DOMAIN_PTR,
|
|
.con_ids = { NULL, },
|
|
};
|
|
|
|
static int __init sh_pm_runtime_init(void)
|
|
{
|
|
if (IS_ENABLED(CONFIG_ARCH_SHMOBILE_MULTI)) {
|
|
if (!of_machine_is_compatible("renesas,emev2") &&
|
|
!of_machine_is_compatible("renesas,r7s72100") &&
|
|
!of_machine_is_compatible("renesas,r8a73a4") &&
|
|
!of_machine_is_compatible("renesas,r8a7740") &&
|
|
!of_machine_is_compatible("renesas,r8a7778") &&
|
|
!of_machine_is_compatible("renesas,r8a7779") &&
|
|
!of_machine_is_compatible("renesas,r8a7790") &&
|
|
!of_machine_is_compatible("renesas,r8a7791") &&
|
|
!of_machine_is_compatible("renesas,r8a7792") &&
|
|
!of_machine_is_compatible("renesas,r8a7793") &&
|
|
!of_machine_is_compatible("renesas,r8a7794") &&
|
|
!of_machine_is_compatible("renesas,sh7372") &&
|
|
!of_machine_is_compatible("renesas,sh73a0"))
|
|
return 0;
|
|
}
|
|
|
|
pm_clk_add_notifier(&platform_bus_type, &platform_bus_notifier);
|
|
return 0;
|
|
}
|
|
core_initcall(sh_pm_runtime_init);
|