mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 00:48:50 +00:00
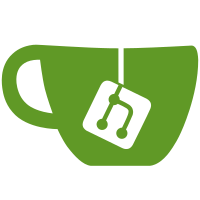
The object-size sanitizer is redundant to -Warray-bounds, and inappropriately performs its checks at run-time when all information needed for the evaluation is available at compile-time, making it quite difficult to use: https://bugzilla.kernel.org/show_bug.cgi?id=214861 With -Warray-bounds almost enabled globally, it doesn't make sense to keep this around. Link: https://lkml.kernel.org/r/20211203235346.110809-1-keescook@chromium.org Signed-off-by: Kees Cook <keescook@chromium.org> Reviewed-by: Marco Elver <elver@google.com> Cc: Masahiro Yamada <masahiroy@kernel.org> Cc: Michal Marek <michal.lkml@markovi.net> Cc: Nick Desaulniers <ndesaulniers@google.com> Cc: Nathan Chancellor <nathan@kernel.org> Cc: Andrey Ryabinin <ryabinin.a.a@gmail.com> Cc: "Peter Zijlstra (Intel)" <peterz@infradead.org> Cc: Stephen Rothwell <sfr@canb.auug.org.au> Cc: Arnd Bergmann <arnd@arndb.de> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
122 lines
2.4 KiB
C
122 lines
2.4 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
#include <linux/init.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/module.h>
|
|
|
|
typedef void(*test_ubsan_fp)(void);
|
|
|
|
#define UBSAN_TEST(config, ...) do { \
|
|
pr_info("%s " __VA_ARGS__ "%s(%s=%s)\n", __func__, \
|
|
sizeof(" " __VA_ARGS__) > 2 ? " " : "", \
|
|
#config, IS_ENABLED(config) ? "y" : "n"); \
|
|
} while (0)
|
|
|
|
static void test_ubsan_divrem_overflow(void)
|
|
{
|
|
volatile int val = 16;
|
|
volatile int val2 = 0;
|
|
|
|
UBSAN_TEST(CONFIG_UBSAN_DIV_ZERO);
|
|
val /= val2;
|
|
}
|
|
|
|
static void test_ubsan_shift_out_of_bounds(void)
|
|
{
|
|
volatile int neg = -1, wrap = 4;
|
|
int val1 = 10;
|
|
int val2 = INT_MAX;
|
|
|
|
UBSAN_TEST(CONFIG_UBSAN_SHIFT, "negative exponent");
|
|
val1 <<= neg;
|
|
|
|
UBSAN_TEST(CONFIG_UBSAN_SHIFT, "left overflow");
|
|
val2 <<= wrap;
|
|
}
|
|
|
|
static void test_ubsan_out_of_bounds(void)
|
|
{
|
|
volatile int i = 4, j = 5, k = -1;
|
|
volatile char above[4] = { }; /* Protect surrounding memory. */
|
|
volatile int arr[4];
|
|
volatile char below[4] = { }; /* Protect surrounding memory. */
|
|
|
|
above[0] = below[0];
|
|
|
|
UBSAN_TEST(CONFIG_UBSAN_BOUNDS, "above");
|
|
arr[j] = i;
|
|
|
|
UBSAN_TEST(CONFIG_UBSAN_BOUNDS, "below");
|
|
arr[k] = i;
|
|
}
|
|
|
|
enum ubsan_test_enum {
|
|
UBSAN_TEST_ZERO = 0,
|
|
UBSAN_TEST_ONE,
|
|
UBSAN_TEST_MAX,
|
|
};
|
|
|
|
static void test_ubsan_load_invalid_value(void)
|
|
{
|
|
volatile char *dst, *src;
|
|
bool val, val2, *ptr;
|
|
enum ubsan_test_enum eval, eval2, *eptr;
|
|
unsigned char c = 0xff;
|
|
|
|
UBSAN_TEST(CONFIG_UBSAN_BOOL, "bool");
|
|
dst = (char *)&val;
|
|
src = &c;
|
|
*dst = *src;
|
|
|
|
ptr = &val2;
|
|
val2 = val;
|
|
|
|
UBSAN_TEST(CONFIG_UBSAN_ENUM, "enum");
|
|
dst = (char *)&eval;
|
|
src = &c;
|
|
*dst = *src;
|
|
|
|
eptr = &eval2;
|
|
eval2 = eval;
|
|
}
|
|
|
|
static void test_ubsan_misaligned_access(void)
|
|
{
|
|
volatile char arr[5] __aligned(4) = {1, 2, 3, 4, 5};
|
|
volatile int *ptr, val = 6;
|
|
|
|
UBSAN_TEST(CONFIG_UBSAN_ALIGNMENT);
|
|
ptr = (int *)(arr + 1);
|
|
*ptr = val;
|
|
}
|
|
|
|
static const test_ubsan_fp test_ubsan_array[] = {
|
|
test_ubsan_shift_out_of_bounds,
|
|
test_ubsan_out_of_bounds,
|
|
test_ubsan_load_invalid_value,
|
|
test_ubsan_misaligned_access,
|
|
};
|
|
|
|
/* Excluded because they Oops the module. */
|
|
static const test_ubsan_fp skip_ubsan_array[] = {
|
|
test_ubsan_divrem_overflow,
|
|
};
|
|
|
|
static int __init test_ubsan_init(void)
|
|
{
|
|
unsigned int i;
|
|
|
|
for (i = 0; i < ARRAY_SIZE(test_ubsan_array); i++)
|
|
test_ubsan_array[i]();
|
|
|
|
return 0;
|
|
}
|
|
module_init(test_ubsan_init);
|
|
|
|
static void __exit test_ubsan_exit(void)
|
|
{
|
|
/* do nothing */
|
|
}
|
|
module_exit(test_ubsan_exit);
|
|
|
|
MODULE_AUTHOR("Jinbum Park <jinb.park7@gmail.com>");
|
|
MODULE_LICENSE("GPL v2");
|