mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-30 04:39:38 +00:00
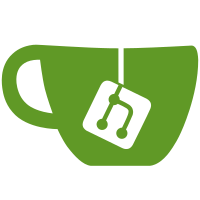
Replace GEM VRAM helpers with GEM SHMEM helpers in ast. Avoids OOM errors when allocating video memory. Also adds support for dma-buf functionality. Aspeed display hardware supports display resolutions of FullHD and higher at 32-bit pixel depth. But the amount of video memory is in the range of 8 MiB to 32 MiB, which adds constraints to the actually available resolutions. As atomic modesetting with VRAM helpers requires double buffering in video memory, ast fails to pageflip in some configurations. For example, FullHD with an active cursor plane does not work on devices with 16 MiB of video memory. Resolve this problem by converting the ast driver to GEM SHMEM helpers. Keep the buffer objects in system memory and copy to video memory on pageflips via shadow-plane helpers. Userspace used to require shadow planes for decent performance, but that's now provided by the driver. To replace the memory management, the patch also implements damage handling for the primary plane. With GEM SHMEM helpers, dma-buf import and export is now supported by ast. This allows easier screen mirroring across devices or with an Aspeed-based BMC. A corresponding feature request is available at [1]. v2: * fix typos in commit message (Jocelyn) Signed-off-by: Thomas Zimmermann <tzimmermann@suse.de> Reviewed-by: Jocelyn Falempe <jfalempe@redhat.com> Tested-by: Jocelyn Falempe <jfalempe@redhat.com> Link: https://lore.kernel.org/dri-devel/20220901124451.2523077-1-oushixiong@kylinos.cn/ # [1] Link: https://patchwork.freedesktop.org/patch/msgid/20221013112923.769-8-tzimmermann@suse.de
101 lines
2.6 KiB
C
101 lines
2.6 KiB
C
/*
|
|
* Copyright 2012 Red Hat Inc.
|
|
*
|
|
* Permission is hereby granted, free of charge, to any person obtaining a
|
|
* copy of this software and associated documentation files (the
|
|
* "Software"), to deal in the Software without restriction, including
|
|
* without limitation the rights to use, copy, modify, merge, publish,
|
|
* distribute, sub license, and/or sell copies of the Software, and to
|
|
* permit persons to whom the Software is furnished to do so, subject to
|
|
* the following conditions:
|
|
*
|
|
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
|
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
|
* FITNESS FOR A PARTICULAR PURPOSE AND NON-INFRINGEMENT. IN NO EVENT SHALL
|
|
* THE COPYRIGHT HOLDERS, AUTHORS AND/OR ITS SUPPLIERS BE LIABLE FOR ANY CLAIM,
|
|
* DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
|
|
* OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE
|
|
* USE OR OTHER DEALINGS IN THE SOFTWARE.
|
|
*
|
|
* The above copyright notice and this permission notice (including the
|
|
* next paragraph) shall be included in all copies or substantial portions
|
|
* of the Software.
|
|
*
|
|
*/
|
|
/*
|
|
* Authors: Dave Airlie <airlied@redhat.com>
|
|
*/
|
|
|
|
#include <linux/pci.h>
|
|
|
|
#include <drm/drm_managed.h>
|
|
#include <drm/drm_print.h>
|
|
|
|
#include "ast_drv.h"
|
|
|
|
static u32 ast_get_vram_size(struct ast_private *ast)
|
|
{
|
|
u8 jreg;
|
|
u32 vram_size;
|
|
|
|
ast_open_key(ast);
|
|
|
|
vram_size = AST_VIDMEM_DEFAULT_SIZE;
|
|
jreg = ast_get_index_reg_mask(ast, AST_IO_CRTC_PORT, 0xaa, 0xff);
|
|
switch (jreg & 3) {
|
|
case 0:
|
|
vram_size = AST_VIDMEM_SIZE_8M;
|
|
break;
|
|
case 1:
|
|
vram_size = AST_VIDMEM_SIZE_16M;
|
|
break;
|
|
case 2:
|
|
vram_size = AST_VIDMEM_SIZE_32M;
|
|
break;
|
|
case 3:
|
|
vram_size = AST_VIDMEM_SIZE_64M;
|
|
break;
|
|
}
|
|
|
|
jreg = ast_get_index_reg_mask(ast, AST_IO_CRTC_PORT, 0x99, 0xff);
|
|
switch (jreg & 0x03) {
|
|
case 1:
|
|
vram_size -= 0x100000;
|
|
break;
|
|
case 2:
|
|
vram_size -= 0x200000;
|
|
break;
|
|
case 3:
|
|
vram_size -= 0x400000;
|
|
break;
|
|
}
|
|
|
|
return vram_size;
|
|
}
|
|
|
|
int ast_mm_init(struct ast_private *ast)
|
|
{
|
|
struct drm_device *dev = &ast->base;
|
|
struct pci_dev *pdev = to_pci_dev(dev->dev);
|
|
resource_size_t base, size;
|
|
u32 vram_size;
|
|
|
|
base = pci_resource_start(pdev, 0);
|
|
size = pci_resource_len(pdev, 0);
|
|
|
|
/* Don't fail on errors, but performance might be reduced. */
|
|
devm_arch_io_reserve_memtype_wc(dev->dev, base, size);
|
|
devm_arch_phys_wc_add(dev->dev, base, size);
|
|
|
|
vram_size = ast_get_vram_size(ast);
|
|
|
|
ast->vram = devm_ioremap_wc(dev->dev, base, vram_size);
|
|
if (!ast->vram)
|
|
return -ENOMEM;
|
|
|
|
ast->vram_base = base;
|
|
ast->vram_size = vram_size;
|
|
ast->vram_fb_available = vram_size;
|
|
|
|
return 0;
|
|
}
|