mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 00:48:50 +00:00
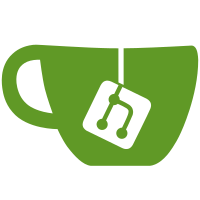
A few architectures, like OMAP, allow you to set a debouncing time for the gpio before generating the IRQ. Teach gpiolib about that. Mark said: : This would be generally useful for embedded systems, especially where : the interrupt concerned is a wake source. It allows drivers to avoid : spurious interrupts from noisy sources so if the hardware supports it : the driver can avoid having to explicitly wait for the signal to become : stable and software has to cope with fewer events. We've lived without : it for quite some time, though. David said: : I looked at adding debounce support to the generic GPIO calls (and thus : gpiolib) some time back, but decided against it. I forget why at this : time (check list archives) but it wasn't because of lack of utility in : certain contexts. : : One thing to watch out for is just how variable the hardware capabilities : are. Atmel GPIOs have something like a fixed number of 32K clock cycles : for debounce, twl4030 had something odd, OMAPs were more like the Atmel : chips but with a different clock. In some cases debouncing had to be : ganged, not per-GPIO. And so forth. Signed-off-by: Felipe Balbi <felipe.balbi@nokia.com> Cc: Tony Lindgren <tony@atomide.com> Cc: David Brownell <david-b@pacbell.net> Reviewed-by: Mark Brown <broonie@opensource.wolfsonmicro.com> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
136 lines
2.6 KiB
C
136 lines
2.6 KiB
C
#ifndef __LINUX_GPIO_H
|
|
#define __LINUX_GPIO_H
|
|
|
|
/* see Documentation/gpio.txt */
|
|
|
|
#ifdef CONFIG_GENERIC_GPIO
|
|
#include <asm/gpio.h>
|
|
|
|
#else
|
|
|
|
#include <linux/kernel.h>
|
|
#include <linux/types.h>
|
|
#include <linux/errno.h>
|
|
|
|
struct device;
|
|
|
|
/*
|
|
* Some platforms don't support the GPIO programming interface.
|
|
*
|
|
* In case some driver uses it anyway (it should normally have
|
|
* depended on GENERIC_GPIO), these routines help the compiler
|
|
* optimize out much GPIO-related code ... or trigger a runtime
|
|
* warning when something is wrongly called.
|
|
*/
|
|
|
|
static inline int gpio_is_valid(int number)
|
|
{
|
|
return 0;
|
|
}
|
|
|
|
static inline int gpio_request(unsigned gpio, const char *label)
|
|
{
|
|
return -ENOSYS;
|
|
}
|
|
|
|
static inline void gpio_free(unsigned gpio)
|
|
{
|
|
might_sleep();
|
|
|
|
/* GPIO can never have been requested */
|
|
WARN_ON(1);
|
|
}
|
|
|
|
static inline int gpio_direction_input(unsigned gpio)
|
|
{
|
|
return -ENOSYS;
|
|
}
|
|
|
|
static inline int gpio_direction_output(unsigned gpio, int value)
|
|
{
|
|
return -ENOSYS;
|
|
}
|
|
|
|
static inline int gpio_set_debounce(unsigned gpio, unsigned debounce)
|
|
{
|
|
return -ENOSYS;
|
|
}
|
|
|
|
static inline int gpio_get_value(unsigned gpio)
|
|
{
|
|
/* GPIO can never have been requested or set as {in,out}put */
|
|
WARN_ON(1);
|
|
return 0;
|
|
}
|
|
|
|
static inline void gpio_set_value(unsigned gpio, int value)
|
|
{
|
|
/* GPIO can never have been requested or set as output */
|
|
WARN_ON(1);
|
|
}
|
|
|
|
static inline int gpio_cansleep(unsigned gpio)
|
|
{
|
|
/* GPIO can never have been requested or set as {in,out}put */
|
|
WARN_ON(1);
|
|
return 0;
|
|
}
|
|
|
|
static inline int gpio_get_value_cansleep(unsigned gpio)
|
|
{
|
|
/* GPIO can never have been requested or set as {in,out}put */
|
|
WARN_ON(1);
|
|
return 0;
|
|
}
|
|
|
|
static inline void gpio_set_value_cansleep(unsigned gpio, int value)
|
|
{
|
|
/* GPIO can never have been requested or set as output */
|
|
WARN_ON(1);
|
|
}
|
|
|
|
static inline int gpio_export(unsigned gpio, bool direction_may_change)
|
|
{
|
|
/* GPIO can never have been requested or set as {in,out}put */
|
|
WARN_ON(1);
|
|
return -EINVAL;
|
|
}
|
|
|
|
static inline int gpio_export_link(struct device *dev, const char *name,
|
|
unsigned gpio)
|
|
{
|
|
/* GPIO can never have been exported */
|
|
WARN_ON(1);
|
|
return -EINVAL;
|
|
}
|
|
|
|
static inline int gpio_sysfs_set_active_low(unsigned gpio, int value)
|
|
{
|
|
/* GPIO can never have been requested */
|
|
WARN_ON(1);
|
|
return -EINVAL;
|
|
}
|
|
|
|
static inline void gpio_unexport(unsigned gpio)
|
|
{
|
|
/* GPIO can never have been exported */
|
|
WARN_ON(1);
|
|
}
|
|
|
|
static inline int gpio_to_irq(unsigned gpio)
|
|
{
|
|
/* GPIO can never have been requested or set as input */
|
|
WARN_ON(1);
|
|
return -EINVAL;
|
|
}
|
|
|
|
static inline int irq_to_gpio(unsigned irq)
|
|
{
|
|
/* irq can never have been returned from gpio_to_irq() */
|
|
WARN_ON(1);
|
|
return -EINVAL;
|
|
}
|
|
|
|
#endif
|
|
|
|
#endif /* __LINUX_GPIO_H */
|