mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-12 21:57:43 +00:00
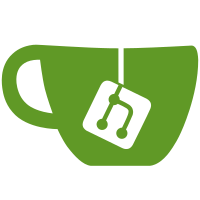
All the current architecture specific defines for these are the same. Refactor these common defines to a common header file. The new common linux/compat_time.h is also useful as it will eventually be used to hold all the defines that are needed for compat time types that support non y2038 safe types. New architectures need not have to define these new types as they will only use new y2038 safe syscalls. This file can be deleted after y2038 when we stop supporting non y2038 safe syscalls. The patch also requires an operation similar to: git grep "asm/compat\.h" | cut -d ":" -f 1 | xargs -n 1 sed -i -e "s%asm/compat.h%linux/compat.h%g" Cc: acme@kernel.org Cc: benh@kernel.crashing.org Cc: borntraeger@de.ibm.com Cc: catalin.marinas@arm.com Cc: cmetcalf@mellanox.com Cc: cohuck@redhat.com Cc: davem@davemloft.net Cc: deller@gmx.de Cc: devel@driverdev.osuosl.org Cc: gerald.schaefer@de.ibm.com Cc: gregkh@linuxfoundation.org Cc: heiko.carstens@de.ibm.com Cc: hoeppner@linux.vnet.ibm.com Cc: hpa@zytor.com Cc: jejb@parisc-linux.org Cc: jwi@linux.vnet.ibm.com Cc: linux-kernel@vger.kernel.org Cc: linux-mips@linux-mips.org Cc: linux-parisc@vger.kernel.org Cc: linuxppc-dev@lists.ozlabs.org Cc: linux-s390@vger.kernel.org Cc: mark.rutland@arm.com Cc: mingo@redhat.com Cc: mpe@ellerman.id.au Cc: oberpar@linux.vnet.ibm.com Cc: oprofile-list@lists.sf.net Cc: paulus@samba.org Cc: peterz@infradead.org Cc: ralf@linux-mips.org Cc: rostedt@goodmis.org Cc: rric@kernel.org Cc: schwidefsky@de.ibm.com Cc: sebott@linux.vnet.ibm.com Cc: sparclinux@vger.kernel.org Cc: sth@linux.vnet.ibm.com Cc: ubraun@linux.vnet.ibm.com Cc: will.deacon@arm.com Cc: x86@kernel.org Signed-off-by: Arnd Bergmann <arnd@arndb.de> Signed-off-by: Deepa Dinamani <deepa.kernel@gmail.com> Acked-by: Steven Rostedt (VMware) <rostedt@goodmis.org> Acked-by: Catalin Marinas <catalin.marinas@arm.com> Acked-by: James Hogan <jhogan@kernel.org> Acked-by: Helge Deller <deller@gmx.de> Signed-off-by: Arnd Bergmann <arnd@arndb.de>
78 lines
2.1 KiB
C
78 lines
2.1 KiB
C
/*
|
|
* This file is subject to the terms and conditions of the GNU General Public
|
|
* License. See the file "COPYING" in the main directory of this archive
|
|
* for more details.
|
|
*
|
|
* Copyright (C) 1991, 1992 Linus Torvalds
|
|
* Copyright (C) 1994 - 2000, 2006 Ralf Baechle
|
|
* Copyright (C) 1999, 2000 Silicon Graphics, Inc.
|
|
* Copyright (C) 2016, Imagination Technologies Ltd.
|
|
*/
|
|
#include <linux/compat.h>
|
|
#include <linux/compiler.h>
|
|
#include <linux/errno.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/signal.h>
|
|
#include <linux/syscalls.h>
|
|
|
|
#include <asm/compat-signal.h>
|
|
#include <linux/uaccess.h>
|
|
#include <asm/unistd.h>
|
|
|
|
#include "signal-common.h"
|
|
|
|
/* 32-bit compatibility types */
|
|
|
|
typedef unsigned int __sighandler32_t;
|
|
typedef void (*vfptr_t)(void);
|
|
|
|
/*
|
|
* Atomically swap in the new signal mask, and wait for a signal.
|
|
*/
|
|
|
|
asmlinkage int sys32_sigsuspend(compat_sigset_t __user *uset)
|
|
{
|
|
return compat_sys_rt_sigsuspend(uset, sizeof(compat_sigset_t));
|
|
}
|
|
|
|
SYSCALL_DEFINE3(32_sigaction, long, sig, const struct compat_sigaction __user *, act,
|
|
struct compat_sigaction __user *, oact)
|
|
{
|
|
struct k_sigaction new_ka, old_ka;
|
|
int ret;
|
|
int err = 0;
|
|
|
|
if (act) {
|
|
old_sigset_t mask;
|
|
s32 handler;
|
|
|
|
if (!access_ok(VERIFY_READ, act, sizeof(*act)))
|
|
return -EFAULT;
|
|
err |= __get_user(handler, &act->sa_handler);
|
|
new_ka.sa.sa_handler = (void __user *)(s64)handler;
|
|
err |= __get_user(new_ka.sa.sa_flags, &act->sa_flags);
|
|
err |= __get_user(mask, &act->sa_mask.sig[0]);
|
|
if (err)
|
|
return -EFAULT;
|
|
|
|
siginitset(&new_ka.sa.sa_mask, mask);
|
|
}
|
|
|
|
ret = do_sigaction(sig, act ? &new_ka : NULL, oact ? &old_ka : NULL);
|
|
|
|
if (!ret && oact) {
|
|
if (!access_ok(VERIFY_WRITE, oact, sizeof(*oact)))
|
|
return -EFAULT;
|
|
err |= __put_user(old_ka.sa.sa_flags, &oact->sa_flags);
|
|
err |= __put_user((u32)(u64)old_ka.sa.sa_handler,
|
|
&oact->sa_handler);
|
|
err |= __put_user(old_ka.sa.sa_mask.sig[0], oact->sa_mask.sig);
|
|
err |= __put_user(0, &oact->sa_mask.sig[1]);
|
|
err |= __put_user(0, &oact->sa_mask.sig[2]);
|
|
err |= __put_user(0, &oact->sa_mask.sig[3]);
|
|
if (err)
|
|
return -EFAULT;
|
|
}
|
|
|
|
return ret;
|
|
}
|