mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-12 21:57:43 +00:00
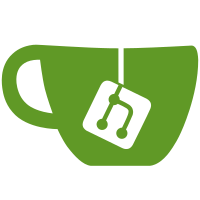
Commit0fa1c57934
("of/fdt: use memblock_virt_alloc for early alloc") inadvertently switched the DT unflattening allocations from memblock to bootmem which doesn't work because the unflattening happens before bootmem is initialized. Swapping the order of bootmem init and unflattening could also fix this, but removing bootmem is desired. So enable NO_BOOTMEM on SH like other architectures have done. Fixes:0fa1c57934
("of/fdt: use memblock_virt_alloc for early alloc") Reported-by: Rich Felker <dalias@libc.org> Cc: Yoshinori Sato <ysato@users.sourceforge.jp> Signed-off-by: Rob Herring <robh@kernel.org> Signed-off-by: Rich Felker <dalias@libc.org>
56 lines
1.6 KiB
C
56 lines
1.6 KiB
C
/*
|
|
* arch/sh/mm/numa.c - Multiple node support for SH machines
|
|
*
|
|
* Copyright (C) 2007 Paul Mundt
|
|
*
|
|
* This file is subject to the terms and conditions of the GNU General Public
|
|
* License. See the file "COPYING" in the main directory of this archive
|
|
* for more details.
|
|
*/
|
|
#include <linux/module.h>
|
|
#include <linux/memblock.h>
|
|
#include <linux/mm.h>
|
|
#include <linux/numa.h>
|
|
#include <linux/pfn.h>
|
|
#include <asm/sections.h>
|
|
|
|
struct pglist_data *node_data[MAX_NUMNODES] __read_mostly;
|
|
EXPORT_SYMBOL_GPL(node_data);
|
|
|
|
/*
|
|
* On SH machines the conventional approach is to stash system RAM
|
|
* in node 0, and other memory blocks in to node 1 and up, ordered by
|
|
* latency. Each node's pgdat is node-local at the beginning of the node,
|
|
* immediately followed by the node mem map.
|
|
*/
|
|
void __init setup_bootmem_node(int nid, unsigned long start, unsigned long end)
|
|
{
|
|
unsigned long start_pfn, end_pfn;
|
|
|
|
/* Don't allow bogus node assignment */
|
|
BUG_ON(nid >= MAX_NUMNODES || nid <= 0);
|
|
|
|
start_pfn = PFN_DOWN(start);
|
|
end_pfn = PFN_DOWN(end);
|
|
|
|
pmb_bolt_mapping((unsigned long)__va(start), start, end - start,
|
|
PAGE_KERNEL);
|
|
|
|
memblock_add(start, end - start);
|
|
|
|
__add_active_range(nid, start_pfn, end_pfn);
|
|
|
|
/* Node-local pgdat */
|
|
NODE_DATA(nid) = __va(memblock_alloc_base(sizeof(struct pglist_data),
|
|
SMP_CACHE_BYTES, end));
|
|
memset(NODE_DATA(nid), 0, sizeof(struct pglist_data));
|
|
|
|
NODE_DATA(nid)->node_start_pfn = start_pfn;
|
|
NODE_DATA(nid)->node_spanned_pages = end_pfn - start_pfn;
|
|
|
|
/* It's up */
|
|
node_set_online(nid);
|
|
|
|
/* Kick sparsemem */
|
|
sparse_memory_present_with_active_regions(nid);
|
|
}
|