mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-10-06 08:46:46 +00:00
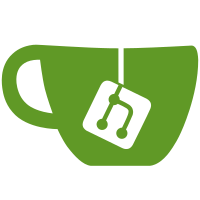
The ADXL375 is fully register map compatible to the ADXL345 (including the device ID register returning the same value ...). The only difference is the resolution of the acceleration sensor. The ADXL375 can measure up to +-200g of acceleration. Datasheet: http://www.analog.com/media/en/technical-documentation/data-sheets/ADXL375.PDF Signed-off-by: Lars-Peter Clausen <lars@metafoo.de> Signed-off-by: Mircea Caprioru <mircea.caprioru@analog.com> Signed-off-by: Jonathan Cameron <Jonathan.Cameron@huawei.com>
76 lines
1.9 KiB
C
76 lines
1.9 KiB
C
/*
|
|
* ADXL345 3-Axis Digital Accelerometer I2C driver
|
|
*
|
|
* Copyright (c) 2017 Eva Rachel Retuya <eraretuya@gmail.com>
|
|
*
|
|
* This file is subject to the terms and conditions of version 2 of
|
|
* the GNU General Public License. See the file COPYING in the main
|
|
* directory of this archive for more details.
|
|
*
|
|
* 7-bit I2C slave address: 0x1D (ALT ADDRESS pin tied to VDDIO) or
|
|
* 0x53 (ALT ADDRESS pin grounded)
|
|
*/
|
|
|
|
#include <linux/i2c.h>
|
|
#include <linux/module.h>
|
|
#include <linux/regmap.h>
|
|
|
|
#include "adxl345.h"
|
|
|
|
static const struct regmap_config adxl345_i2c_regmap_config = {
|
|
.reg_bits = 8,
|
|
.val_bits = 8,
|
|
};
|
|
|
|
static int adxl345_i2c_probe(struct i2c_client *client,
|
|
const struct i2c_device_id *id)
|
|
{
|
|
struct regmap *regmap;
|
|
|
|
regmap = devm_regmap_init_i2c(client, &adxl345_i2c_regmap_config);
|
|
if (IS_ERR(regmap)) {
|
|
dev_err(&client->dev, "Error initializing i2c regmap: %ld\n",
|
|
PTR_ERR(regmap));
|
|
return PTR_ERR(regmap);
|
|
}
|
|
|
|
return adxl345_core_probe(&client->dev, regmap, id->driver_data,
|
|
id ? id->name : NULL);
|
|
}
|
|
|
|
static int adxl345_i2c_remove(struct i2c_client *client)
|
|
{
|
|
return adxl345_core_remove(&client->dev);
|
|
}
|
|
|
|
static const struct i2c_device_id adxl345_i2c_id[] = {
|
|
{ "adxl345", ADXL345 },
|
|
{ "adxl375", ADXL375 },
|
|
{ }
|
|
};
|
|
|
|
MODULE_DEVICE_TABLE(i2c, adxl345_i2c_id);
|
|
|
|
static const struct of_device_id adxl345_of_match[] = {
|
|
{ .compatible = "adi,adxl345" },
|
|
{ .compatible = "adi,adxl375" },
|
|
{ },
|
|
};
|
|
|
|
MODULE_DEVICE_TABLE(of, adxl345_of_match);
|
|
|
|
static struct i2c_driver adxl345_i2c_driver = {
|
|
.driver = {
|
|
.name = "adxl345_i2c",
|
|
.of_match_table = adxl345_of_match,
|
|
},
|
|
.probe = adxl345_i2c_probe,
|
|
.remove = adxl345_i2c_remove,
|
|
.id_table = adxl345_i2c_id,
|
|
};
|
|
|
|
module_i2c_driver(adxl345_i2c_driver);
|
|
|
|
MODULE_AUTHOR("Eva Rachel Retuya <eraretuya@gmail.com>");
|
|
MODULE_DESCRIPTION("ADXL345 3-Axis Digital Accelerometer I2C driver");
|
|
MODULE_LICENSE("GPL v2");
|