mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-16 07:35:14 +00:00
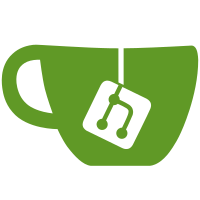
commit1ba0e9d69b
upstream. In8e9fad0e70
"io_uring: Add io_uring command support for sockets" you've got an include of asm-generic/ioctls.h done in io_uring/uring_cmd.c. That had been done for the sake of this chunk - + ret = prot->ioctl(sk, SIOCINQ, &arg); + if (ret) + return ret; + return arg; + case SOCKET_URING_OP_SIOCOUTQ: + ret = prot->ioctl(sk, SIOCOUTQ, &arg); SIOC{IN,OUT}Q are defined to symbols (FIONREAD and TIOCOUTQ) that come from ioctls.h, all right, but the values vary by the architecture. FIONREAD is 0x467F on mips 0x4004667F on alpha, powerpc and sparc 0x8004667F on sh and xtensa 0x541B everywhere else TIOCOUTQ is 0x7472 on mips 0x40047473 on alpha, powerpc and sparc 0x80047473 on sh and xtensa 0x5411 everywhere else ->ioctl() expects the same values it would've gotten from userland; all places where we compare with SIOC{IN,OUT}Q are using asm/ioctls.h, so they pick the correct values. io_uring_cmd_sock(), OTOH, ends up passing the default ones. Fixes:8e9fad0e70
("io_uring: Add io_uring command support for sockets") Cc: <stable@vger.kernel.org> Signed-off-by: Al Viro <viro@zeniv.linux.org.uk> Link: https://lore.kernel.org/r/20231214213408.GT1674809@ZenIV Signed-off-by: Jens Axboe <axboe@kernel.dk> Signed-off-by: Greg Kroah-Hartman <gregkh@linuxfoundation.org>
193 lines
4.9 KiB
C
193 lines
4.9 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
#include <linux/kernel.h>
|
|
#include <linux/errno.h>
|
|
#include <linux/file.h>
|
|
#include <linux/io_uring.h>
|
|
#include <linux/security.h>
|
|
#include <linux/nospec.h>
|
|
|
|
#include <uapi/linux/io_uring.h>
|
|
#include <asm/ioctls.h>
|
|
|
|
#include "io_uring.h"
|
|
#include "rsrc.h"
|
|
#include "uring_cmd.h"
|
|
|
|
static void io_uring_cmd_work(struct io_kiocb *req, struct io_tw_state *ts)
|
|
{
|
|
struct io_uring_cmd *ioucmd = io_kiocb_to_cmd(req, struct io_uring_cmd);
|
|
unsigned issue_flags = ts->locked ? 0 : IO_URING_F_UNLOCKED;
|
|
|
|
ioucmd->task_work_cb(ioucmd, issue_flags);
|
|
}
|
|
|
|
void __io_uring_cmd_do_in_task(struct io_uring_cmd *ioucmd,
|
|
void (*task_work_cb)(struct io_uring_cmd *, unsigned),
|
|
unsigned flags)
|
|
{
|
|
struct io_kiocb *req = cmd_to_io_kiocb(ioucmd);
|
|
|
|
ioucmd->task_work_cb = task_work_cb;
|
|
req->io_task_work.func = io_uring_cmd_work;
|
|
__io_req_task_work_add(req, flags);
|
|
}
|
|
EXPORT_SYMBOL_GPL(__io_uring_cmd_do_in_task);
|
|
|
|
void io_uring_cmd_do_in_task_lazy(struct io_uring_cmd *ioucmd,
|
|
void (*task_work_cb)(struct io_uring_cmd *, unsigned))
|
|
{
|
|
__io_uring_cmd_do_in_task(ioucmd, task_work_cb, IOU_F_TWQ_LAZY_WAKE);
|
|
}
|
|
EXPORT_SYMBOL_GPL(io_uring_cmd_do_in_task_lazy);
|
|
|
|
static inline void io_req_set_cqe32_extra(struct io_kiocb *req,
|
|
u64 extra1, u64 extra2)
|
|
{
|
|
req->big_cqe.extra1 = extra1;
|
|
req->big_cqe.extra2 = extra2;
|
|
}
|
|
|
|
/*
|
|
* Called by consumers of io_uring_cmd, if they originally returned
|
|
* -EIOCBQUEUED upon receiving the command.
|
|
*/
|
|
void io_uring_cmd_done(struct io_uring_cmd *ioucmd, ssize_t ret, ssize_t res2,
|
|
unsigned issue_flags)
|
|
{
|
|
struct io_kiocb *req = cmd_to_io_kiocb(ioucmd);
|
|
|
|
if (ret < 0)
|
|
req_set_fail(req);
|
|
|
|
io_req_set_res(req, ret, 0);
|
|
if (req->ctx->flags & IORING_SETUP_CQE32)
|
|
io_req_set_cqe32_extra(req, res2, 0);
|
|
if (req->ctx->flags & IORING_SETUP_IOPOLL) {
|
|
/* order with io_iopoll_req_issued() checking ->iopoll_complete */
|
|
smp_store_release(&req->iopoll_completed, 1);
|
|
} else {
|
|
struct io_tw_state ts = {
|
|
.locked = !(issue_flags & IO_URING_F_UNLOCKED),
|
|
};
|
|
io_req_task_complete(req, &ts);
|
|
}
|
|
}
|
|
EXPORT_SYMBOL_GPL(io_uring_cmd_done);
|
|
|
|
int io_uring_cmd_prep_async(struct io_kiocb *req)
|
|
{
|
|
struct io_uring_cmd *ioucmd = io_kiocb_to_cmd(req, struct io_uring_cmd);
|
|
|
|
memcpy(req->async_data, ioucmd->sqe, uring_sqe_size(req->ctx));
|
|
ioucmd->sqe = req->async_data;
|
|
return 0;
|
|
}
|
|
|
|
int io_uring_cmd_prep(struct io_kiocb *req, const struct io_uring_sqe *sqe)
|
|
{
|
|
struct io_uring_cmd *ioucmd = io_kiocb_to_cmd(req, struct io_uring_cmd);
|
|
|
|
if (sqe->__pad1)
|
|
return -EINVAL;
|
|
|
|
ioucmd->flags = READ_ONCE(sqe->uring_cmd_flags);
|
|
if (ioucmd->flags & ~IORING_URING_CMD_FIXED)
|
|
return -EINVAL;
|
|
|
|
if (ioucmd->flags & IORING_URING_CMD_FIXED) {
|
|
struct io_ring_ctx *ctx = req->ctx;
|
|
u16 index;
|
|
|
|
req->buf_index = READ_ONCE(sqe->buf_index);
|
|
if (unlikely(req->buf_index >= ctx->nr_user_bufs))
|
|
return -EFAULT;
|
|
index = array_index_nospec(req->buf_index, ctx->nr_user_bufs);
|
|
req->imu = ctx->user_bufs[index];
|
|
io_req_set_rsrc_node(req, ctx, 0);
|
|
}
|
|
ioucmd->sqe = sqe;
|
|
ioucmd->cmd_op = READ_ONCE(sqe->cmd_op);
|
|
return 0;
|
|
}
|
|
|
|
int io_uring_cmd(struct io_kiocb *req, unsigned int issue_flags)
|
|
{
|
|
struct io_uring_cmd *ioucmd = io_kiocb_to_cmd(req, struct io_uring_cmd);
|
|
struct io_ring_ctx *ctx = req->ctx;
|
|
struct file *file = req->file;
|
|
int ret;
|
|
|
|
if (!file->f_op->uring_cmd)
|
|
return -EOPNOTSUPP;
|
|
|
|
ret = security_uring_cmd(ioucmd);
|
|
if (ret)
|
|
return ret;
|
|
|
|
if (ctx->flags & IORING_SETUP_SQE128)
|
|
issue_flags |= IO_URING_F_SQE128;
|
|
if (ctx->flags & IORING_SETUP_CQE32)
|
|
issue_flags |= IO_URING_F_CQE32;
|
|
if (ctx->flags & IORING_SETUP_IOPOLL) {
|
|
if (!file->f_op->uring_cmd_iopoll)
|
|
return -EOPNOTSUPP;
|
|
issue_flags |= IO_URING_F_IOPOLL;
|
|
req->iopoll_completed = 0;
|
|
WRITE_ONCE(ioucmd->cookie, NULL);
|
|
}
|
|
|
|
ret = file->f_op->uring_cmd(ioucmd, issue_flags);
|
|
if (ret == -EAGAIN) {
|
|
if (!req_has_async_data(req)) {
|
|
if (io_alloc_async_data(req))
|
|
return -ENOMEM;
|
|
io_uring_cmd_prep_async(req);
|
|
}
|
|
return -EAGAIN;
|
|
}
|
|
|
|
if (ret != -EIOCBQUEUED) {
|
|
if (ret < 0)
|
|
req_set_fail(req);
|
|
io_req_set_res(req, ret, 0);
|
|
return ret;
|
|
}
|
|
|
|
return IOU_ISSUE_SKIP_COMPLETE;
|
|
}
|
|
|
|
int io_uring_cmd_import_fixed(u64 ubuf, unsigned long len, int rw,
|
|
struct iov_iter *iter, void *ioucmd)
|
|
{
|
|
struct io_kiocb *req = cmd_to_io_kiocb(ioucmd);
|
|
|
|
return io_import_fixed(rw, iter, req->imu, ubuf, len);
|
|
}
|
|
EXPORT_SYMBOL_GPL(io_uring_cmd_import_fixed);
|
|
|
|
int io_uring_cmd_sock(struct io_uring_cmd *cmd, unsigned int issue_flags)
|
|
{
|
|
struct socket *sock = cmd->file->private_data;
|
|
struct sock *sk = sock->sk;
|
|
struct proto *prot = READ_ONCE(sk->sk_prot);
|
|
int ret, arg = 0;
|
|
|
|
if (!prot || !prot->ioctl)
|
|
return -EOPNOTSUPP;
|
|
|
|
switch (cmd->sqe->cmd_op) {
|
|
case SOCKET_URING_OP_SIOCINQ:
|
|
ret = prot->ioctl(sk, SIOCINQ, &arg);
|
|
if (ret)
|
|
return ret;
|
|
return arg;
|
|
case SOCKET_URING_OP_SIOCOUTQ:
|
|
ret = prot->ioctl(sk, SIOCOUTQ, &arg);
|
|
if (ret)
|
|
return ret;
|
|
return arg;
|
|
default:
|
|
return -EOPNOTSUPP;
|
|
}
|
|
}
|
|
EXPORT_SYMBOL_GPL(io_uring_cmd_sock);
|