mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-10-08 17:49:45 +00:00
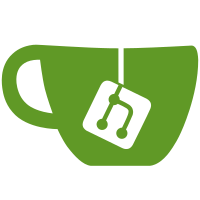
We unified the Freescale pci/pcie initialization by changing the fsl_pci to a platform driver. In previous PCI code architecture the initialization routine is called at board_setup_arch stage. Now the initialization is done in probe function which is architectural better. Also It's convenient for adding PM support for PCI controller in later patch. Now we registered pci controllers as platform devices. So we combine two initialization code as one platform driver. Signed-off-by: Jia Hongtao <B38951@freescale.com> Signed-off-by: Li Yang <leoli@freescale.com> Signed-off-by: Chunhe Lan <Chunhe.Lan@freescale.com> Signed-off-by: Kumar Gala <galak@kernel.crashing.org>
111 lines
2.5 KiB
C
111 lines
2.5 KiB
C
/*
|
|
* Based on MPC8560 ADS and arch/ppc stx_gp3 ports
|
|
*
|
|
* Maintained by Kumar Gala (see MAINTAINERS for contact information)
|
|
*
|
|
* Copyright 2008 Freescale Semiconductor Inc.
|
|
*
|
|
* Dan Malek <dan@embeddededge.com>
|
|
* Copyright 2004 Embedded Edge, LLC
|
|
*
|
|
* Copied from mpc8560_ads.c
|
|
* Copyright 2002, 2003 Motorola Inc.
|
|
*
|
|
* Ported to 2.6, Matt Porter <mporter@kernel.crashing.org>
|
|
* Copyright 2004-2005 MontaVista Software, Inc.
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify it
|
|
* under the terms of the GNU General Public License as published by the
|
|
* Free Software Foundation; either version 2 of the License, or (at your
|
|
* option) any later version.
|
|
*/
|
|
|
|
#include <linux/stddef.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/pci.h>
|
|
#include <linux/kdev_t.h>
|
|
#include <linux/delay.h>
|
|
#include <linux/seq_file.h>
|
|
#include <linux/of_platform.h>
|
|
|
|
#include <asm/time.h>
|
|
#include <asm/machdep.h>
|
|
#include <asm/pci-bridge.h>
|
|
#include <asm/mpic.h>
|
|
#include <asm/prom.h>
|
|
#include <mm/mmu_decl.h>
|
|
#include <asm/udbg.h>
|
|
|
|
#include <sysdev/fsl_soc.h>
|
|
#include <sysdev/fsl_pci.h>
|
|
|
|
#include "mpc85xx.h"
|
|
|
|
#ifdef CONFIG_CPM2
|
|
#include <asm/cpm2.h>
|
|
#endif /* CONFIG_CPM2 */
|
|
|
|
static void __init stx_gp3_pic_init(void)
|
|
{
|
|
struct mpic *mpic = mpic_alloc(NULL, 0, MPIC_BIG_ENDIAN,
|
|
0, 256, " OpenPIC ");
|
|
BUG_ON(mpic == NULL);
|
|
mpic_init(mpic);
|
|
|
|
mpc85xx_cpm2_pic_init();
|
|
}
|
|
|
|
/*
|
|
* Setup the architecture
|
|
*/
|
|
static void __init stx_gp3_setup_arch(void)
|
|
{
|
|
if (ppc_md.progress)
|
|
ppc_md.progress("stx_gp3_setup_arch()", 0);
|
|
|
|
fsl_pci_assign_primary();
|
|
|
|
#ifdef CONFIG_CPM2
|
|
cpm2_reset();
|
|
#endif
|
|
}
|
|
|
|
static void stx_gp3_show_cpuinfo(struct seq_file *m)
|
|
{
|
|
uint pvid, svid, phid1;
|
|
|
|
pvid = mfspr(SPRN_PVR);
|
|
svid = mfspr(SPRN_SVR);
|
|
|
|
seq_printf(m, "Vendor\t\t: RPC Electronics STx\n");
|
|
seq_printf(m, "PVR\t\t: 0x%x\n", pvid);
|
|
seq_printf(m, "SVR\t\t: 0x%x\n", svid);
|
|
|
|
/* Display cpu Pll setting */
|
|
phid1 = mfspr(SPRN_HID1);
|
|
seq_printf(m, "PLL setting\t: 0x%x\n", ((phid1 >> 24) & 0x3f));
|
|
}
|
|
|
|
machine_arch_initcall(stx_gp3, mpc85xx_common_publish_devices);
|
|
|
|
/*
|
|
* Called very early, device-tree isn't unflattened
|
|
*/
|
|
static int __init stx_gp3_probe(void)
|
|
{
|
|
unsigned long root = of_get_flat_dt_root();
|
|
|
|
return of_flat_dt_is_compatible(root, "stx,gp3-8560");
|
|
}
|
|
|
|
define_machine(stx_gp3) {
|
|
.name = "STX GP3",
|
|
.probe = stx_gp3_probe,
|
|
.setup_arch = stx_gp3_setup_arch,
|
|
.init_IRQ = stx_gp3_pic_init,
|
|
.show_cpuinfo = stx_gp3_show_cpuinfo,
|
|
.get_irq = mpic_get_irq,
|
|
.restart = fsl_rstcr_restart,
|
|
.calibrate_decr = generic_calibrate_decr,
|
|
.progress = udbg_progress,
|
|
};
|