mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-03 17:40:04 +00:00
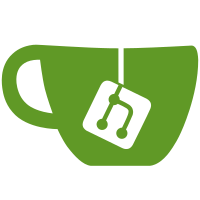
It's nice to be able to test a tagging protocol with dsa_loop, but not
at the cost of losing the ability of building the tagging protocol and
switch driver as modules, because as things stand, there is a circular
dependency between the two. Tagging protocol drivers cannot depend on
switch drivers, that is a hard fact.
The reasoning behind the blamed patch was that accessing dp->priv should
first make sure that the structure behind that pointer is what we really
think it is.
Currently the "sja1105" and "sja1110" tagging protocols only operate
with the sja1105 switch driver, just like any other tagging protocol and
switch combination. The only way to mix and match them is by modifying
the code, and this applies to dsa_loop as well (by default that uses
DSA_TAG_PROTO_NONE). So while in principle there is an issue, in
practice there isn't one.
Until we extend dsa_loop to allow user space configuration, treat the
problem as a non-issue and just say that DSA ports found by tag_sja1105
are always sja1105 ports, which is in fact true. But keep the
dsa_port_is_sja1105 function so that it's easy to patch it during
testing, and rely on dead code elimination.
Fixes: 994d2cbb08
("net: dsa: tag_sja1105: be dsa_loop-safe")
Link: https://lore.kernel.org/netdev/20210908220834.d7gmtnwrorhharna@skbuf/
Signed-off-by: Vladimir Oltean <vladimir.oltean@nxp.com>
Signed-off-by: David S. Miller <davem@davemloft.net>
95 lines
2.6 KiB
C
95 lines
2.6 KiB
C
/* SPDX-License-Identifier: GPL-2.0
|
|
* Copyright (c) 2019, Vladimir Oltean <olteanv@gmail.com>
|
|
*/
|
|
|
|
/* Included by drivers/net/dsa/sja1105/sja1105.h and net/dsa/tag_sja1105.c */
|
|
|
|
#ifndef _NET_DSA_SJA1105_H
|
|
#define _NET_DSA_SJA1105_H
|
|
|
|
#include <linux/skbuff.h>
|
|
#include <linux/etherdevice.h>
|
|
#include <linux/dsa/8021q.h>
|
|
#include <net/dsa.h>
|
|
|
|
#define ETH_P_SJA1105 ETH_P_DSA_8021Q
|
|
#define ETH_P_SJA1105_META 0x0008
|
|
#define ETH_P_SJA1110 0xdadc
|
|
|
|
#define SJA1105_DEFAULT_VLAN (VLAN_N_VID - 1)
|
|
|
|
/* IEEE 802.3 Annex 57A: Slow Protocols PDUs (01:80:C2:xx:xx:xx) */
|
|
#define SJA1105_LINKLOCAL_FILTER_A 0x0180C2000000ull
|
|
#define SJA1105_LINKLOCAL_FILTER_A_MASK 0xFFFFFF000000ull
|
|
/* IEEE 1588 Annex F: Transport of PTP over Ethernet (01:1B:19:xx:xx:xx) */
|
|
#define SJA1105_LINKLOCAL_FILTER_B 0x011B19000000ull
|
|
#define SJA1105_LINKLOCAL_FILTER_B_MASK 0xFFFFFF000000ull
|
|
|
|
/* Source and Destination MAC of follow-up meta frames.
|
|
* Whereas the choice of SMAC only affects the unique identification of the
|
|
* switch as sender of meta frames, the DMAC must be an address that is present
|
|
* in the DSA master port's multicast MAC filter.
|
|
* 01-80-C2-00-00-0E is a good choice for this, as all profiles of IEEE 1588
|
|
* over L2 use this address for some purpose already.
|
|
*/
|
|
#define SJA1105_META_SMAC 0x222222222222ull
|
|
#define SJA1105_META_DMAC 0x0180C200000Eull
|
|
|
|
#define SJA1105_HWTS_RX_EN 0
|
|
|
|
/* Global tagger data: each struct sja1105_port has a reference to
|
|
* the structure defined in struct sja1105_private.
|
|
*/
|
|
struct sja1105_tagger_data {
|
|
struct sk_buff *stampable_skb;
|
|
/* Protects concurrent access to the meta state machine
|
|
* from taggers running on multiple ports on SMP systems
|
|
*/
|
|
spinlock_t meta_lock;
|
|
unsigned long state;
|
|
u8 ts_id;
|
|
/* Used on SJA1110 where meta frames are generated only for
|
|
* 2-step TX timestamps
|
|
*/
|
|
struct sk_buff_head skb_txtstamp_queue;
|
|
};
|
|
|
|
struct sja1105_skb_cb {
|
|
struct sk_buff *clone;
|
|
u64 tstamp;
|
|
/* Only valid for packets cloned for 2-step TX timestamping */
|
|
u8 ts_id;
|
|
};
|
|
|
|
#define SJA1105_SKB_CB(skb) \
|
|
((struct sja1105_skb_cb *)((skb)->cb))
|
|
|
|
struct sja1105_port {
|
|
struct kthread_worker *xmit_worker;
|
|
struct kthread_work xmit_work;
|
|
struct sk_buff_head xmit_queue;
|
|
struct sja1105_tagger_data *data;
|
|
bool hwts_tx_en;
|
|
};
|
|
|
|
/* Timestamps are in units of 8 ns clock ticks (equivalent to
|
|
* a fixed 125 MHz clock).
|
|
*/
|
|
#define SJA1105_TICK_NS 8
|
|
|
|
static inline s64 ns_to_sja1105_ticks(s64 ns)
|
|
{
|
|
return ns / SJA1105_TICK_NS;
|
|
}
|
|
|
|
static inline s64 sja1105_ticks_to_ns(s64 ticks)
|
|
{
|
|
return ticks * SJA1105_TICK_NS;
|
|
}
|
|
|
|
static inline bool dsa_port_is_sja1105(struct dsa_port *dp)
|
|
{
|
|
return true;
|
|
}
|
|
|
|
#endif /* _NET_DSA_SJA1105_H */
|