mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-10-01 06:33:07 +00:00
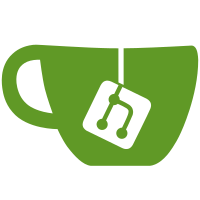
In SoundWire mode leave hard RESET asserted when exiting probe, and wait for an UNATTACHED notification before deasserting RESET. If the boot state of the reset GPIO was deasserted it is possible that the SoundWire core had already enumerated the CS42L42 before cs42l42_sdw_probe() is called. When cs42l42_common_probe() hard resets the CS42L42 it triggers a race condition: 1) After cs42l42_sdw_probe() returns the thread that called it will call cs42l42_sdw_update_status() to report the last status recorded by the SoundWire core. 2) The SoundWire bus master will see a PING with the CS42L42 now reporting as unenumerated and will trigger the core SoundWire code to start enumerating CS42L42. These two threads are racing against each other. If (1) happens before (2) a stale ATTACHED notification will be reported to the cs42l42 driver when in fact the status of cs42l42 is now unattached. To avoid this race condition: - Leave RESET asserted on exit from cs42l42_sdw_probe(). This ensures that an UNATTACHED notification must be sent to the cs42l42 driver. If cs42l42 was already enumerated it will be seen to drop off the bus, causing an UNATTACH notification. If it was never enumerated the status is already UNATTACHED and this will be reported by thread (1). - When the UNATTACH notification is received, release RESET. This will cause CS42L42 to be enumerated and eventually report an ATTACHED notification. - The ATTACHED notification is now valid. Signed-off-by: Richard Fitzgerald <rf@opensource.cirrus.com> Signed-off-by: Stefan Binding <sbinding@opensource.cirrus.com> Link: https://lore.kernel.org/r/20230913150012.604775-4-sbinding@opensource.cirrus.com Signed-off-by: Mark Brown <broonie@kernel.org>
82 lines
2.4 KiB
C
82 lines
2.4 KiB
C
/* SPDX-License-Identifier: GPL-2.0-only */
|
|
/*
|
|
* cs42l42.h -- CS42L42 ALSA SoC audio driver header
|
|
*
|
|
* Copyright 2016-2022 Cirrus Logic, Inc.
|
|
*
|
|
* Author: James Schulman <james.schulman@cirrus.com>
|
|
* Author: Brian Austin <brian.austin@cirrus.com>
|
|
* Author: Michael White <michael.white@cirrus.com>
|
|
*/
|
|
|
|
#ifndef __CS42L42_H__
|
|
#define __CS42L42_H__
|
|
|
|
#include <dt-bindings/sound/cs42l42.h>
|
|
#include <linux/device.h>
|
|
#include <linux/gpio.h>
|
|
#include <linux/mutex.h>
|
|
#include <linux/regmap.h>
|
|
#include <linux/regulator/consumer.h>
|
|
#include <linux/soundwire/sdw.h>
|
|
#include <sound/jack.h>
|
|
#include <sound/cs42l42.h>
|
|
#include <sound/soc-component.h>
|
|
#include <sound/soc-dai.h>
|
|
|
|
struct cs42l42_private {
|
|
struct regmap *regmap;
|
|
struct device *dev;
|
|
struct regulator_bulk_data supplies[CS42L42_NUM_SUPPLIES];
|
|
struct gpio_desc *reset_gpio;
|
|
struct completion pdn_done;
|
|
struct snd_soc_jack *jack;
|
|
struct sdw_slave *sdw_peripheral;
|
|
struct mutex irq_lock;
|
|
int devid;
|
|
int irq;
|
|
int pll_config;
|
|
u32 sclk;
|
|
u32 sample_rate;
|
|
u32 bclk_ratio;
|
|
u8 plug_state;
|
|
u8 hs_type;
|
|
u8 ts_inv;
|
|
u8 ts_dbnc_rise;
|
|
u8 ts_dbnc_fall;
|
|
u8 btn_det_init_dbnce;
|
|
u8 btn_det_event_dbnce;
|
|
u8 bias_thresholds[CS42L42_NUM_BIASES];
|
|
u8 hs_bias_ramp_rate;
|
|
u8 hs_bias_ramp_time;
|
|
u8 hs_bias_sense_en;
|
|
u8 stream_use;
|
|
bool hp_adc_up_pending;
|
|
bool suspended;
|
|
bool sdw_waiting_first_unattach;
|
|
bool init_done;
|
|
};
|
|
|
|
extern const struct regmap_range_cfg cs42l42_page_range;
|
|
extern const struct regmap_config cs42l42_regmap;
|
|
extern const struct snd_soc_component_driver cs42l42_soc_component;
|
|
extern struct snd_soc_dai_driver cs42l42_dai;
|
|
|
|
bool cs42l42_readable_register(struct device *dev, unsigned int reg);
|
|
bool cs42l42_volatile_register(struct device *dev, unsigned int reg);
|
|
|
|
int cs42l42_pll_config(struct snd_soc_component *component,
|
|
unsigned int clk, unsigned int sample_rate);
|
|
void cs42l42_src_config(struct snd_soc_component *component, unsigned int sample_rate);
|
|
int cs42l42_mute_stream(struct snd_soc_dai *dai, int mute, int stream);
|
|
irqreturn_t cs42l42_irq_thread(int irq, void *data);
|
|
int cs42l42_suspend(struct device *dev);
|
|
int cs42l42_resume(struct device *dev);
|
|
void cs42l42_resume_restore(struct device *dev);
|
|
int cs42l42_common_probe(struct cs42l42_private *cs42l42,
|
|
const struct snd_soc_component_driver *component_drv,
|
|
struct snd_soc_dai_driver *dai);
|
|
int cs42l42_init(struct cs42l42_private *cs42l42);
|
|
void cs42l42_common_remove(struct cs42l42_private *cs42l42);
|
|
|
|
#endif /* __CS42L42_H__ */
|