mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-30 06:10:56 +00:00
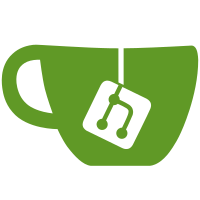
The replacement of <asm/pgrable.h> with <linux/pgtable.h> made the include of the latter in the middle of asm includes. Fix this up with the aid of the below script and manual adjustments here and there. import sys import re if len(sys.argv) is not 3: print "USAGE: %s <file> <header>" % (sys.argv[0]) sys.exit(1) hdr_to_move="#include <linux/%s>" % sys.argv[2] moved = False in_hdrs = False with open(sys.argv[1], "r") as f: lines = f.readlines() for _line in lines: line = _line.rstrip(' ') if line == hdr_to_move: continue if line.startswith("#include <linux/"): in_hdrs = True elif not moved and in_hdrs: moved = True print hdr_to_move print line Signed-off-by: Mike Rapoport <rppt@linux.ibm.com> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Cc: Arnd Bergmann <arnd@arndb.de> Cc: Borislav Petkov <bp@alien8.de> Cc: Brian Cain <bcain@codeaurora.org> Cc: Catalin Marinas <catalin.marinas@arm.com> Cc: Chris Zankel <chris@zankel.net> Cc: "David S. Miller" <davem@davemloft.net> Cc: Geert Uytterhoeven <geert@linux-m68k.org> Cc: Greentime Hu <green.hu@gmail.com> Cc: Greg Ungerer <gerg@linux-m68k.org> Cc: Guan Xuetao <gxt@pku.edu.cn> Cc: Guo Ren <guoren@kernel.org> Cc: Heiko Carstens <heiko.carstens@de.ibm.com> Cc: Helge Deller <deller@gmx.de> Cc: Ingo Molnar <mingo@redhat.com> Cc: Ley Foon Tan <ley.foon.tan@intel.com> Cc: Mark Salter <msalter@redhat.com> Cc: Matthew Wilcox <willy@infradead.org> Cc: Matt Turner <mattst88@gmail.com> Cc: Max Filippov <jcmvbkbc@gmail.com> Cc: Michael Ellerman <mpe@ellerman.id.au> Cc: Michal Simek <monstr@monstr.eu> Cc: Nick Hu <nickhu@andestech.com> Cc: Paul Walmsley <paul.walmsley@sifive.com> Cc: Richard Weinberger <richard@nod.at> Cc: Rich Felker <dalias@libc.org> Cc: Russell King <linux@armlinux.org.uk> Cc: Stafford Horne <shorne@gmail.com> Cc: Thomas Bogendoerfer <tsbogend@alpha.franken.de> Cc: Thomas Gleixner <tglx@linutronix.de> Cc: Tony Luck <tony.luck@intel.com> Cc: Vincent Chen <deanbo422@gmail.com> Cc: Vineet Gupta <vgupta@synopsys.com> Cc: Will Deacon <will@kernel.org> Cc: Yoshinori Sato <ysato@users.sourceforge.jp> Link: http://lkml.kernel.org/r/20200514170327.31389-4-rppt@kernel.org Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
96 lines
2.1 KiB
C
96 lines
2.1 KiB
C
// SPDX-License-Identifier: GPL-2.0-only
|
|
/*
|
|
* linux/arch/arm/mach-integrator/core.c
|
|
*
|
|
* Copyright (C) 2000-2003 Deep Blue Solutions Ltd
|
|
*/
|
|
#include <linux/types.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/init.h>
|
|
#include <linux/device.h>
|
|
#include <linux/export.h>
|
|
#include <linux/spinlock.h>
|
|
#include <linux/interrupt.h>
|
|
#include <linux/irq.h>
|
|
#include <linux/memblock.h>
|
|
#include <linux/sched.h>
|
|
#include <linux/smp.h>
|
|
#include <linux/amba/bus.h>
|
|
#include <linux/amba/serial.h>
|
|
#include <linux/io.h>
|
|
#include <linux/stat.h>
|
|
#include <linux/of.h>
|
|
#include <linux/of_address.h>
|
|
#include <linux/pgtable.h>
|
|
|
|
#include <asm/mach-types.h>
|
|
#include <asm/mach/time.h>
|
|
|
|
#include "hardware.h"
|
|
#include "cm.h"
|
|
#include "common.h"
|
|
|
|
static DEFINE_RAW_SPINLOCK(cm_lock);
|
|
static void __iomem *cm_base;
|
|
|
|
/**
|
|
* cm_get - get the value from the CM_CTRL register
|
|
*/
|
|
u32 cm_get(void)
|
|
{
|
|
return readl(cm_base + INTEGRATOR_HDR_CTRL_OFFSET);
|
|
}
|
|
|
|
/**
|
|
* cm_control - update the CM_CTRL register.
|
|
* @mask: bits to change
|
|
* @set: bits to set
|
|
*/
|
|
void cm_control(u32 mask, u32 set)
|
|
{
|
|
unsigned long flags;
|
|
u32 val;
|
|
|
|
raw_spin_lock_irqsave(&cm_lock, flags);
|
|
val = readl(cm_base + INTEGRATOR_HDR_CTRL_OFFSET) & ~mask;
|
|
writel(val | set, cm_base + INTEGRATOR_HDR_CTRL_OFFSET);
|
|
raw_spin_unlock_irqrestore(&cm_lock, flags);
|
|
}
|
|
|
|
void cm_clear_irqs(void)
|
|
{
|
|
/* disable core module IRQs */
|
|
writel(0xffffffffU, cm_base + INTEGRATOR_HDR_IC_OFFSET +
|
|
IRQ_ENABLE_CLEAR);
|
|
}
|
|
|
|
static const struct of_device_id cm_match[] = {
|
|
{ .compatible = "arm,core-module-integrator"},
|
|
{ },
|
|
};
|
|
|
|
void cm_init(void)
|
|
{
|
|
struct device_node *cm = of_find_matching_node(NULL, cm_match);
|
|
|
|
if (!cm) {
|
|
pr_crit("no core module node found in device tree\n");
|
|
return;
|
|
}
|
|
cm_base = of_iomap(cm, 0);
|
|
if (!cm_base) {
|
|
pr_crit("could not remap core module\n");
|
|
return;
|
|
}
|
|
cm_clear_irqs();
|
|
}
|
|
|
|
/*
|
|
* We need to stop things allocating the low memory; ideally we need a
|
|
* better implementation of GFP_DMA which does not assume that DMA-able
|
|
* memory starts at zero.
|
|
*/
|
|
void __init integrator_reserve(void)
|
|
{
|
|
memblock_reserve(PHYS_OFFSET, __pa(swapper_pg_dir) - PHYS_OFFSET);
|
|
}
|