mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-29 22:02:02 +00:00
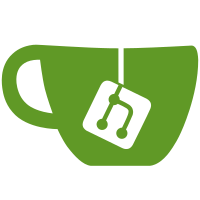
We can now init system timers using the dmtimer and 32k counter based on only devicetree data and drivers/clocksource timers. Let's configure the clocksource and clockevent, and drop the old unused platform data. As we're just dropping platform data, and the early platform data init is based on the custom ti,hwmods property, we want to drop both the platform data and ti,hwmods property in a single patch. Since the dmtimer can use both 32k clock and system clock as the source, let's also configure the SoC specific default values. The board specific dts files can reconfigure these with assigned-clocks and assigned-clock-parents as needed. Cc: devicetree@vger.kernel.org Cc: Grygorii Strashko <grygorii.strashko@ti.com> Cc: Keerthy <j-keerthy@ti.com> Cc: Lokesh Vutla <lokeshvutla@ti.com> Cc: Rob Herring <robh@kernel.org> Cc: Tero Kristo <t-kristo@ti.com> Signed-off-by: Tony Lindgren <tony@atomide.com>
297 lines
7.3 KiB
C
297 lines
7.3 KiB
C
/*
|
|
* omap_hwmod_33xx_data.c: Hardware modules present on the AM33XX chips
|
|
*
|
|
* Copyright (C) {2012} Texas Instruments Incorporated - http://www.ti.com/
|
|
*
|
|
* This file is automatically generated from the AM33XX hardware databases.
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License as
|
|
* published by the Free Software Foundation version 2.
|
|
*
|
|
* This program is distributed "as is" WITHOUT ANY WARRANTY of any
|
|
* kind, whether express or implied; without even the implied warranty
|
|
* of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*/
|
|
|
|
#include "omap_hwmod.h"
|
|
#include "omap_hwmod_common_data.h"
|
|
|
|
#include "control.h"
|
|
#include "cm33xx.h"
|
|
#include "prm33xx.h"
|
|
#include "prm-regbits-33xx.h"
|
|
#include "omap_hwmod_33xx_43xx_common_data.h"
|
|
|
|
/*
|
|
* IP blocks
|
|
*/
|
|
|
|
/* emif */
|
|
static struct omap_hwmod am33xx_emif_hwmod = {
|
|
.name = "emif",
|
|
.class = &am33xx_emif_hwmod_class,
|
|
.clkdm_name = "l3_clkdm",
|
|
.flags = HWMOD_INIT_NO_IDLE,
|
|
.main_clk = "dpll_ddr_m2_div2_ck",
|
|
.prcm = {
|
|
.omap4 = {
|
|
.clkctrl_offs = AM33XX_CM_PER_EMIF_CLKCTRL_OFFSET,
|
|
.modulemode = MODULEMODE_SWCTRL,
|
|
},
|
|
},
|
|
};
|
|
|
|
/* l4_hs */
|
|
static struct omap_hwmod am33xx_l4_hs_hwmod = {
|
|
.name = "l4_hs",
|
|
.class = &am33xx_l4_hwmod_class,
|
|
.clkdm_name = "l4hs_clkdm",
|
|
.flags = HWMOD_INIT_NO_IDLE,
|
|
.main_clk = "l4hs_gclk",
|
|
.prcm = {
|
|
.omap4 = {
|
|
.clkctrl_offs = AM33XX_CM_PER_L4HS_CLKCTRL_OFFSET,
|
|
.modulemode = MODULEMODE_SWCTRL,
|
|
},
|
|
},
|
|
};
|
|
|
|
static struct omap_hwmod_rst_info am33xx_wkup_m3_resets[] = {
|
|
{ .name = "wkup_m3", .rst_shift = 3, .st_shift = 5 },
|
|
};
|
|
|
|
/* wkup_m3 */
|
|
static struct omap_hwmod am33xx_wkup_m3_hwmod = {
|
|
.name = "wkup_m3",
|
|
.class = &am33xx_wkup_m3_hwmod_class,
|
|
.clkdm_name = "l4_wkup_aon_clkdm",
|
|
/* Keep hardreset asserted */
|
|
.flags = HWMOD_INIT_NO_RESET | HWMOD_NO_IDLEST,
|
|
.main_clk = "dpll_core_m4_div2_ck",
|
|
.prcm = {
|
|
.omap4 = {
|
|
.clkctrl_offs = AM33XX_CM_WKUP_WKUP_M3_CLKCTRL_OFFSET,
|
|
.rstctrl_offs = AM33XX_RM_WKUP_RSTCTRL_OFFSET,
|
|
.rstst_offs = AM33XX_RM_WKUP_RSTST_OFFSET,
|
|
.modulemode = MODULEMODE_SWCTRL,
|
|
},
|
|
},
|
|
.rst_lines = am33xx_wkup_m3_resets,
|
|
.rst_lines_cnt = ARRAY_SIZE(am33xx_wkup_m3_resets),
|
|
};
|
|
|
|
|
|
/*
|
|
* Modules omap_hwmod structures
|
|
*
|
|
* The following IPs are excluded for the moment because:
|
|
* - They do not need an explicit SW control using omap_hwmod API.
|
|
* - They still need to be validated with the driver
|
|
* properly adapted to omap_hwmod / omap_device
|
|
*
|
|
* - cEFUSE (doesn't fall under any ocp_if)
|
|
* - clkdiv32k
|
|
* - ocp watch point
|
|
*/
|
|
#if 0
|
|
/*
|
|
* 'cefuse' class
|
|
*/
|
|
static struct omap_hwmod_class am33xx_cefuse_hwmod_class = {
|
|
.name = "cefuse",
|
|
};
|
|
|
|
static struct omap_hwmod am33xx_cefuse_hwmod = {
|
|
.name = "cefuse",
|
|
.class = &am33xx_cefuse_hwmod_class,
|
|
.clkdm_name = "l4_cefuse_clkdm",
|
|
.main_clk = "cefuse_fck",
|
|
.prcm = {
|
|
.omap4 = {
|
|
.clkctrl_offs = AM33XX_CM_CEFUSE_CEFUSE_CLKCTRL_OFFSET,
|
|
.modulemode = MODULEMODE_SWCTRL,
|
|
},
|
|
},
|
|
};
|
|
|
|
/*
|
|
* 'clkdiv32k' class
|
|
*/
|
|
static struct omap_hwmod_class am33xx_clkdiv32k_hwmod_class = {
|
|
.name = "clkdiv32k",
|
|
};
|
|
|
|
static struct omap_hwmod am33xx_clkdiv32k_hwmod = {
|
|
.name = "clkdiv32k",
|
|
.class = &am33xx_clkdiv32k_hwmod_class,
|
|
.clkdm_name = "clk_24mhz_clkdm",
|
|
.main_clk = "clkdiv32k_ick",
|
|
.prcm = {
|
|
.omap4 = {
|
|
.clkctrl_offs = AM33XX_CM_PER_CLKDIV32K_CLKCTRL_OFFSET,
|
|
.modulemode = MODULEMODE_SWCTRL,
|
|
},
|
|
},
|
|
};
|
|
|
|
/* ocpwp */
|
|
static struct omap_hwmod_class am33xx_ocpwp_hwmod_class = {
|
|
.name = "ocpwp",
|
|
};
|
|
|
|
static struct omap_hwmod am33xx_ocpwp_hwmod = {
|
|
.name = "ocpwp",
|
|
.class = &am33xx_ocpwp_hwmod_class,
|
|
.clkdm_name = "l4ls_clkdm",
|
|
.main_clk = "l4ls_gclk",
|
|
.prcm = {
|
|
.omap4 = {
|
|
.clkctrl_offs = AM33XX_CM_PER_OCPWP_CLKCTRL_OFFSET,
|
|
.modulemode = MODULEMODE_SWCTRL,
|
|
},
|
|
},
|
|
};
|
|
#endif
|
|
|
|
/*
|
|
* 'debugss' class
|
|
* debug sub system
|
|
*/
|
|
static struct omap_hwmod_opt_clk debugss_opt_clks[] = {
|
|
{ .role = "dbg_sysclk", .clk = "dbg_sysclk_ck" },
|
|
{ .role = "dbg_clka", .clk = "dbg_clka_ck" },
|
|
};
|
|
|
|
static struct omap_hwmod_class am33xx_debugss_hwmod_class = {
|
|
.name = "debugss",
|
|
};
|
|
|
|
static struct omap_hwmod am33xx_debugss_hwmod = {
|
|
.name = "debugss",
|
|
.class = &am33xx_debugss_hwmod_class,
|
|
.clkdm_name = "l3_aon_clkdm",
|
|
.main_clk = "trace_clk_div_ck",
|
|
.prcm = {
|
|
.omap4 = {
|
|
.clkctrl_offs = AM33XX_CM_WKUP_DEBUGSS_CLKCTRL_OFFSET,
|
|
.modulemode = MODULEMODE_SWCTRL,
|
|
},
|
|
},
|
|
.opt_clks = debugss_opt_clks,
|
|
.opt_clks_cnt = ARRAY_SIZE(debugss_opt_clks),
|
|
};
|
|
|
|
static struct omap_hwmod am33xx_control_hwmod = {
|
|
.name = "control",
|
|
.class = &am33xx_control_hwmod_class,
|
|
.clkdm_name = "l4_wkup_clkdm",
|
|
.flags = HWMOD_INIT_NO_IDLE,
|
|
.main_clk = "dpll_core_m4_div2_ck",
|
|
.prcm = {
|
|
.omap4 = {
|
|
.clkctrl_offs = AM33XX_CM_WKUP_CONTROL_CLKCTRL_OFFSET,
|
|
.modulemode = MODULEMODE_SWCTRL,
|
|
},
|
|
},
|
|
};
|
|
|
|
|
|
/*
|
|
* Interfaces
|
|
*/
|
|
|
|
/* l3 main -> emif */
|
|
static struct omap_hwmod_ocp_if am33xx_l3_main__emif = {
|
|
.master = &am33xx_l3_main_hwmod,
|
|
.slave = &am33xx_emif_hwmod,
|
|
.clk = "dpll_core_m4_ck",
|
|
.user = OCP_USER_MPU | OCP_USER_SDMA,
|
|
};
|
|
|
|
/* l3 main -> l4 hs */
|
|
static struct omap_hwmod_ocp_if am33xx_l3_main__l4_hs = {
|
|
.master = &am33xx_l3_main_hwmod,
|
|
.slave = &am33xx_l4_hs_hwmod,
|
|
.clk = "l3s_gclk",
|
|
.user = OCP_USER_MPU | OCP_USER_SDMA,
|
|
};
|
|
|
|
/* wkup m3 -> l4 wkup */
|
|
static struct omap_hwmod_ocp_if am33xx_wkup_m3__l4_wkup = {
|
|
.master = &am33xx_wkup_m3_hwmod,
|
|
.slave = &am33xx_l4_wkup_hwmod,
|
|
.clk = "dpll_core_m4_div2_ck",
|
|
.user = OCP_USER_MPU | OCP_USER_SDMA,
|
|
};
|
|
|
|
/* l4 wkup -> wkup m3 */
|
|
static struct omap_hwmod_ocp_if am33xx_l4_wkup__wkup_m3 = {
|
|
.master = &am33xx_l4_wkup_hwmod,
|
|
.slave = &am33xx_wkup_m3_hwmod,
|
|
.clk = "dpll_core_m4_div2_ck",
|
|
.user = OCP_USER_MPU | OCP_USER_SDMA,
|
|
};
|
|
|
|
/* l3_main -> debugss */
|
|
static struct omap_hwmod_ocp_if am33xx_l3_main__debugss = {
|
|
.master = &am33xx_l3_main_hwmod,
|
|
.slave = &am33xx_debugss_hwmod,
|
|
.clk = "dpll_core_m4_ck",
|
|
.user = OCP_USER_MPU,
|
|
};
|
|
|
|
/* l4 wkup -> smartreflex0 */
|
|
static struct omap_hwmod_ocp_if am33xx_l4_wkup__smartreflex0 = {
|
|
.master = &am33xx_l4_wkup_hwmod,
|
|
.slave = &am33xx_smartreflex0_hwmod,
|
|
.clk = "dpll_core_m4_div2_ck",
|
|
.user = OCP_USER_MPU,
|
|
};
|
|
|
|
/* l4 wkup -> smartreflex1 */
|
|
static struct omap_hwmod_ocp_if am33xx_l4_wkup__smartreflex1 = {
|
|
.master = &am33xx_l4_wkup_hwmod,
|
|
.slave = &am33xx_smartreflex1_hwmod,
|
|
.clk = "dpll_core_m4_div2_ck",
|
|
.user = OCP_USER_MPU,
|
|
};
|
|
|
|
/* l4 wkup -> control */
|
|
static struct omap_hwmod_ocp_if am33xx_l4_wkup__control = {
|
|
.master = &am33xx_l4_wkup_hwmod,
|
|
.slave = &am33xx_control_hwmod,
|
|
.clk = "dpll_core_m4_div2_ck",
|
|
.user = OCP_USER_MPU,
|
|
};
|
|
|
|
static struct omap_hwmod_ocp_if *am33xx_hwmod_ocp_ifs[] __initdata = {
|
|
&am33xx_l3_main__emif,
|
|
&am33xx_mpu__l3_main,
|
|
&am33xx_mpu__prcm,
|
|
&am33xx_l3_s__l4_ls,
|
|
&am33xx_l3_s__l4_wkup,
|
|
&am33xx_l3_main__l4_hs,
|
|
&am33xx_l3_main__l3_s,
|
|
&am33xx_l3_main__l3_instr,
|
|
&am33xx_l3_main__gfx,
|
|
&am33xx_l3_s__l3_main,
|
|
&am33xx_wkup_m3__l4_wkup,
|
|
&am33xx_gfx__l3_main,
|
|
&am33xx_l3_main__debugss,
|
|
&am33xx_l4_wkup__wkup_m3,
|
|
&am33xx_l4_wkup__control,
|
|
&am33xx_l4_wkup__smartreflex0,
|
|
&am33xx_l4_wkup__smartreflex1,
|
|
&am33xx_l4_wkup__rtc,
|
|
&am33xx_l3_s__gpmc,
|
|
&am33xx_l3_main__ocmc,
|
|
NULL,
|
|
};
|
|
|
|
int __init am33xx_hwmod_init(void)
|
|
{
|
|
omap_hwmod_am33xx_reg();
|
|
omap_hwmod_init();
|
|
return omap_hwmod_register_links(am33xx_hwmod_ocp_ifs);
|
|
}
|