mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-23 01:10:04 +00:00
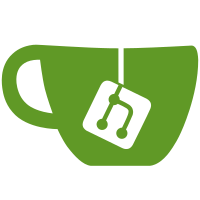
regulator: fixed/gpio: Revert GPIO descriptor changes due to platform breakage Commit6059577cb2
"regulator: fixed: Convert to use GPIO descriptor only" broke at least the ams-delta platform since the lookup tables added to the board files use the function name "enable" while the driver uses NULL causing the regulator to not acquire and control the enable GPIOs. Revert that and a couple of other commits that are caught up with it to fix the issue:2b6c00c157
"ARM: pxa, regulator: fix building ezx e680"6059577cb2
"regulator: fixed: Convert to use GPIO descriptor only"37bed97f00
"regulator: gpio: Get enable GPIO using GPIO descriptor" Reported-by: Janusz Krzysztofik <jmkrzyszt@gmail.com> Signed-off-by: Mark Brown <broonie@kernel.org>
62 lines
1.6 KiB
C
62 lines
1.6 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
#include <linux/slab.h>
|
|
#include <linux/string.h>
|
|
#include <linux/platform_device.h>
|
|
#include <linux/regulator/machine.h>
|
|
#include <linux/regulator/fixed.h>
|
|
|
|
struct fixed_regulator_data {
|
|
struct fixed_voltage_config cfg;
|
|
struct regulator_init_data init_data;
|
|
struct platform_device pdev;
|
|
};
|
|
|
|
static void regulator_fixed_release(struct device *dev)
|
|
{
|
|
struct fixed_regulator_data *data = container_of(dev,
|
|
struct fixed_regulator_data, pdev.dev);
|
|
kfree(data->cfg.supply_name);
|
|
kfree(data);
|
|
}
|
|
|
|
/**
|
|
* regulator_register_fixed_name - register a no-op fixed regulator
|
|
* @id: platform device id
|
|
* @name: name to be used for the regulator
|
|
* @supplies: consumers for this regulator
|
|
* @num_supplies: number of consumers
|
|
* @uv: voltage in microvolts
|
|
*/
|
|
struct platform_device *regulator_register_always_on(int id, const char *name,
|
|
struct regulator_consumer_supply *supplies, int num_supplies, int uv)
|
|
{
|
|
struct fixed_regulator_data *data;
|
|
|
|
data = kzalloc(sizeof(*data), GFP_KERNEL);
|
|
if (!data)
|
|
return NULL;
|
|
|
|
data->cfg.supply_name = kstrdup(name, GFP_KERNEL);
|
|
if (!data->cfg.supply_name) {
|
|
kfree(data);
|
|
return NULL;
|
|
}
|
|
|
|
data->cfg.microvolts = uv;
|
|
data->cfg.gpio = -EINVAL;
|
|
data->cfg.enabled_at_boot = 1;
|
|
data->cfg.init_data = &data->init_data;
|
|
|
|
data->init_data.constraints.always_on = 1;
|
|
data->init_data.consumer_supplies = supplies;
|
|
data->init_data.num_consumer_supplies = num_supplies;
|
|
|
|
data->pdev.name = "reg-fixed-voltage";
|
|
data->pdev.id = id;
|
|
data->pdev.dev.platform_data = &data->cfg;
|
|
data->pdev.dev.release = regulator_fixed_release;
|
|
|
|
platform_device_register(&data->pdev);
|
|
|
|
return &data->pdev;
|
|
}
|