mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-24 01:41:39 +00:00
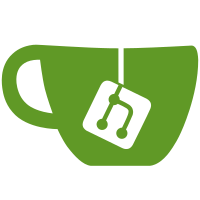
Add a HID transport driver to support integrated HID devices on newer Microsoft Surface models (specifically 7th-generation, i.e. Surface Laptop 3, Surface Book 3, and later). On those models, the internal keyboard and touchpad (as well as some other HID devices with currently unknown function) are connected via the generic HID subsystem (TC=0x15) of the Surface System Aggregator Module (SSAM). This subsystem provides a generic HID transport layer, support for which is implemented by this driver. Co-developed-by: Blaž Hrastnik <blaz@mxxn.io> Signed-off-by: Blaž Hrastnik <blaz@mxxn.io> Signed-off-by: Maximilian Luz <luzmaximilian@gmail.com> Signed-off-by: Jiri Kosina <jkosina@suse.cz>
77 lines
2 KiB
C
77 lines
2 KiB
C
/* SPDX-License-Identifier: GPL-2.0+ */
|
|
/*
|
|
* Common/core components for the Surface System Aggregator Module (SSAM) HID
|
|
* transport driver. Provides support for integrated HID devices on Microsoft
|
|
* Surface models.
|
|
*
|
|
* Copyright (C) 2019-2021 Maximilian Luz <luzmaximilian@gmail.com>
|
|
*/
|
|
|
|
#ifndef SURFACE_HID_CORE_H
|
|
#define SURFACE_HID_CORE_H
|
|
|
|
#include <linux/hid.h>
|
|
#include <linux/pm.h>
|
|
#include <linux/types.h>
|
|
|
|
#include <linux/surface_aggregator/controller.h>
|
|
#include <linux/surface_aggregator/device.h>
|
|
|
|
enum surface_hid_descriptor_entry {
|
|
SURFACE_HID_DESC_HID = 0,
|
|
SURFACE_HID_DESC_REPORT = 1,
|
|
SURFACE_HID_DESC_ATTRS = 2,
|
|
};
|
|
|
|
struct surface_hid_descriptor {
|
|
__u8 desc_len; /* = 9 */
|
|
__u8 desc_type; /* = HID_DT_HID */
|
|
__le16 hid_version;
|
|
__u8 country_code;
|
|
__u8 num_descriptors; /* = 1 */
|
|
|
|
__u8 report_desc_type; /* = HID_DT_REPORT */
|
|
__le16 report_desc_len;
|
|
} __packed;
|
|
|
|
static_assert(sizeof(struct surface_hid_descriptor) == 9);
|
|
|
|
struct surface_hid_attributes {
|
|
__le32 length;
|
|
__le16 vendor;
|
|
__le16 product;
|
|
__le16 version;
|
|
__u8 _unknown[22];
|
|
} __packed;
|
|
|
|
static_assert(sizeof(struct surface_hid_attributes) == 32);
|
|
|
|
struct surface_hid_device;
|
|
|
|
struct surface_hid_device_ops {
|
|
int (*get_descriptor)(struct surface_hid_device *shid, u8 entry, u8 *buf, size_t len);
|
|
int (*output_report)(struct surface_hid_device *shid, u8 rprt_id, u8 *buf, size_t len);
|
|
int (*get_feature_report)(struct surface_hid_device *shid, u8 rprt_id, u8 *buf, size_t len);
|
|
int (*set_feature_report)(struct surface_hid_device *shid, u8 rprt_id, u8 *buf, size_t len);
|
|
};
|
|
|
|
struct surface_hid_device {
|
|
struct device *dev;
|
|
struct ssam_controller *ctrl;
|
|
struct ssam_device_uid uid;
|
|
|
|
struct surface_hid_descriptor hid_desc;
|
|
struct surface_hid_attributes attrs;
|
|
|
|
struct ssam_event_notifier notif;
|
|
struct hid_device *hid;
|
|
|
|
struct surface_hid_device_ops ops;
|
|
};
|
|
|
|
int surface_hid_device_add(struct surface_hid_device *shid);
|
|
void surface_hid_device_destroy(struct surface_hid_device *shid);
|
|
|
|
extern const struct dev_pm_ops surface_hid_pm_ops;
|
|
|
|
#endif /* SURFACE_HID_CORE_H */
|