mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-12 13:55:32 +00:00
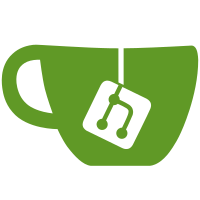
Patch series "sections: Unify kernel sections range check and use", v4.
There are three head files(kallsyms.h, kernel.h and sections.h) which
include the kernel sections range check, let's make some cleanup and unify
them.
1. cleanup arch specific text/data check and fix address boundary check
in kallsyms.h
2. make all the basic/core kernel range check function into sections.h
3. update all the callers, and use the helper in sections.h to simplify
the code
After this series, we have 5 APIs about kernel sections range check in
sections.h
* is_kernel_rodata() --- already in sections.h
* is_kernel_core_data() --- come from core_kernel_data() in kernel.h
* is_kernel_inittext() --- come from kernel.h and kallsyms.h
* __is_kernel_text() --- add new internal helper
* __is_kernel() --- add new internal helper
Note: For the last two helpers, people should not use directly, consider to
use corresponding function in kallsyms.h.
This patch (of 11):
Remove arch specific text and data check after commit 4ba66a9760
("arch:
remove blackfin port"), no need arch-specific text/data check.
Link: https://lkml.kernel.org/r/20210930071143.63410-1-wangkefeng.wang@huawei.com
Link: https://lkml.kernel.org/r/20210930071143.63410-2-wangkefeng.wang@huawei.com
Signed-off-by: Kefeng Wang <wangkefeng.wang@huawei.com>
Reviewed-by: Sergey Senozhatsky <senozhatsky@chromium.org>
Cc: Arnd Bergmann <arnd@arndb.de>
Cc: Steven Rostedt <rostedt@goodmis.org>
Cc: Ingo Molnar <mingo@redhat.com>
Cc: David S. Miller <davem@davemloft.net>
Cc: Alexei Starovoitov <ast@kernel.org>
Cc: Andrey Ryabinin <ryabinin.a.a@gmail.com>
Cc: Michael Ellerman <mpe@ellerman.id.au>
Cc: Benjamin Herrenschmidt <benh@kernel.crashing.org>
Cc: Paul Mackerras <paulus@samba.org>
Cc: Christophe Leroy <christophe.leroy@csgroup.eu>
Cc: Kefeng Wang <wangkefeng.wang@huawei.com>
Cc: Alexander Potapenko <glider@google.com>
Cc: Andrey Konovalov <andreyknvl@gmail.com>
Cc: Borislav Petkov <bp@alien8.de>
Cc: Dmitry Vyukov <dvyukov@google.com>
Cc: Ivan Kokshaysky <ink@jurassic.park.msu.ru>
Cc: Matt Turner <mattst88@gmail.com>
Cc: Michal Simek <monstr@monstr.eu>
Cc: Petr Mladek <pmladek@suse.com>
Cc: Richard Henderson <rth@twiddle.net>
Cc: Thomas Gleixner <tglx@linutronix.de>
Signed-off-by: Andrew Morton <akpm@linux-foundation.org>
Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
181 lines
4.5 KiB
C
181 lines
4.5 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
/* Rewritten and vastly simplified by Rusty Russell for in-kernel
|
|
* module loader:
|
|
* Copyright 2002 Rusty Russell <rusty@rustcorp.com.au> IBM Corporation
|
|
*/
|
|
#ifndef _LINUX_KALLSYMS_H
|
|
#define _LINUX_KALLSYMS_H
|
|
|
|
#include <linux/errno.h>
|
|
#include <linux/buildid.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/stddef.h>
|
|
#include <linux/mm.h>
|
|
#include <linux/module.h>
|
|
|
|
#include <asm/sections.h>
|
|
|
|
#define KSYM_NAME_LEN 128
|
|
#define KSYM_SYMBOL_LEN (sizeof("%s+%#lx/%#lx [%s %s]") + \
|
|
(KSYM_NAME_LEN - 1) + \
|
|
2*(BITS_PER_LONG*3/10) + (MODULE_NAME_LEN - 1) + \
|
|
(BUILD_ID_SIZE_MAX * 2) + 1)
|
|
|
|
struct cred;
|
|
struct module;
|
|
|
|
static inline int is_kernel_inittext(unsigned long addr)
|
|
{
|
|
if (addr >= (unsigned long)_sinittext
|
|
&& addr <= (unsigned long)_einittext)
|
|
return 1;
|
|
return 0;
|
|
}
|
|
|
|
static inline int is_kernel_text(unsigned long addr)
|
|
{
|
|
if ((addr >= (unsigned long)_stext && addr <= (unsigned long)_etext))
|
|
return 1;
|
|
return in_gate_area_no_mm(addr);
|
|
}
|
|
|
|
static inline int is_kernel(unsigned long addr)
|
|
{
|
|
if (addr >= (unsigned long)_stext && addr <= (unsigned long)_end)
|
|
return 1;
|
|
return in_gate_area_no_mm(addr);
|
|
}
|
|
|
|
static inline int is_ksym_addr(unsigned long addr)
|
|
{
|
|
if (IS_ENABLED(CONFIG_KALLSYMS_ALL))
|
|
return is_kernel(addr);
|
|
|
|
return is_kernel_text(addr) || is_kernel_inittext(addr);
|
|
}
|
|
|
|
static inline void *dereference_symbol_descriptor(void *ptr)
|
|
{
|
|
#ifdef HAVE_DEREFERENCE_FUNCTION_DESCRIPTOR
|
|
struct module *mod;
|
|
|
|
ptr = dereference_kernel_function_descriptor(ptr);
|
|
if (is_ksym_addr((unsigned long)ptr))
|
|
return ptr;
|
|
|
|
preempt_disable();
|
|
mod = __module_address((unsigned long)ptr);
|
|
preempt_enable();
|
|
|
|
if (mod)
|
|
ptr = dereference_module_function_descriptor(mod, ptr);
|
|
#endif
|
|
return ptr;
|
|
}
|
|
|
|
int kallsyms_on_each_symbol(int (*fn)(void *, const char *, struct module *,
|
|
unsigned long),
|
|
void *data);
|
|
|
|
#ifdef CONFIG_KALLSYMS
|
|
/* Lookup the address for a symbol. Returns 0 if not found. */
|
|
unsigned long kallsyms_lookup_name(const char *name);
|
|
|
|
extern int kallsyms_lookup_size_offset(unsigned long addr,
|
|
unsigned long *symbolsize,
|
|
unsigned long *offset);
|
|
|
|
/* Lookup an address. modname is set to NULL if it's in the kernel. */
|
|
const char *kallsyms_lookup(unsigned long addr,
|
|
unsigned long *symbolsize,
|
|
unsigned long *offset,
|
|
char **modname, char *namebuf);
|
|
|
|
/* Look up a kernel symbol and return it in a text buffer. */
|
|
extern int sprint_symbol(char *buffer, unsigned long address);
|
|
extern int sprint_symbol_build_id(char *buffer, unsigned long address);
|
|
extern int sprint_symbol_no_offset(char *buffer, unsigned long address);
|
|
extern int sprint_backtrace(char *buffer, unsigned long address);
|
|
extern int sprint_backtrace_build_id(char *buffer, unsigned long address);
|
|
|
|
int lookup_symbol_name(unsigned long addr, char *symname);
|
|
int lookup_symbol_attrs(unsigned long addr, unsigned long *size, unsigned long *offset, char *modname, char *name);
|
|
|
|
/* How and when do we show kallsyms values? */
|
|
extern bool kallsyms_show_value(const struct cred *cred);
|
|
|
|
#else /* !CONFIG_KALLSYMS */
|
|
|
|
static inline unsigned long kallsyms_lookup_name(const char *name)
|
|
{
|
|
return 0;
|
|
}
|
|
|
|
static inline int kallsyms_lookup_size_offset(unsigned long addr,
|
|
unsigned long *symbolsize,
|
|
unsigned long *offset)
|
|
{
|
|
return 0;
|
|
}
|
|
|
|
static inline const char *kallsyms_lookup(unsigned long addr,
|
|
unsigned long *symbolsize,
|
|
unsigned long *offset,
|
|
char **modname, char *namebuf)
|
|
{
|
|
return NULL;
|
|
}
|
|
|
|
static inline int sprint_symbol(char *buffer, unsigned long addr)
|
|
{
|
|
*buffer = '\0';
|
|
return 0;
|
|
}
|
|
|
|
static inline int sprint_symbol_build_id(char *buffer, unsigned long address)
|
|
{
|
|
*buffer = '\0';
|
|
return 0;
|
|
}
|
|
|
|
static inline int sprint_symbol_no_offset(char *buffer, unsigned long addr)
|
|
{
|
|
*buffer = '\0';
|
|
return 0;
|
|
}
|
|
|
|
static inline int sprint_backtrace(char *buffer, unsigned long addr)
|
|
{
|
|
*buffer = '\0';
|
|
return 0;
|
|
}
|
|
|
|
static inline int sprint_backtrace_build_id(char *buffer, unsigned long addr)
|
|
{
|
|
*buffer = '\0';
|
|
return 0;
|
|
}
|
|
|
|
static inline int lookup_symbol_name(unsigned long addr, char *symname)
|
|
{
|
|
return -ERANGE;
|
|
}
|
|
|
|
static inline int lookup_symbol_attrs(unsigned long addr, unsigned long *size, unsigned long *offset, char *modname, char *name)
|
|
{
|
|
return -ERANGE;
|
|
}
|
|
|
|
static inline bool kallsyms_show_value(const struct cred *cred)
|
|
{
|
|
return false;
|
|
}
|
|
|
|
#endif /*CONFIG_KALLSYMS*/
|
|
|
|
static inline void print_ip_sym(const char *loglvl, unsigned long ip)
|
|
{
|
|
printk("%s[<%px>] %pS\n", loglvl, (void *) ip, (void *) ip);
|
|
}
|
|
|
|
#endif /*_LINUX_KALLSYMS_H*/
|