mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-15 23:25:07 +00:00
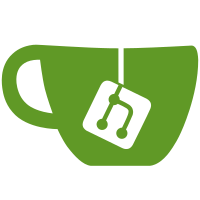
Patch enhances current metric infrastructure to handle "?" in the metric expression. The "?" can be use for parameters whose value not known while creating metric events and which can be replace later at runtime to the proper value. It also add flexibility to create multiple events out of single metric event added in JSON file. Patch adds function 'arch_get_runtimeparam' which is a arch specific function, returns the count of metric events need to be created. By default it return 1. This infrastructure needed for hv_24x7 socket/chip level events. "hv_24x7" chip level events needs specific chip-id to which the data is requested. Function 'arch_get_runtimeparam' implemented in header.c which extract number of sockets from sysfs file "sockets" under "/sys/devices/hv_24x7/interface/". With this patch basically we are trying to create as many metric events as define by runtime_param. For that one loop is added in function 'metricgroup__add_metric', which create multiple events at run time depend on return value of 'arch_get_runtimeparam' and merge that event in 'group_list'. To achieve that we are actually passing this parameter value as part of `expr__find_other` function and changing "?" present in metric expression with this value. As in our JSON file, there gonna be single metric event, and out of which we are creating multiple events. To understand which data count belongs to which parameter value, we also printing param value in generic_metric function. For example, command:# ./perf stat -M PowerBUS_Frequency -C 0 -I 1000 1.000101867 9,356,933 hv_24x7/pm_pb_cyc,chip=0/ # 2.3 GHz PowerBUS_Frequency_0 1.000101867 9,366,134 hv_24x7/pm_pb_cyc,chip=1/ # 2.3 GHz PowerBUS_Frequency_1 2.000314878 9,365,868 hv_24x7/pm_pb_cyc,chip=0/ # 2.3 GHz PowerBUS_Frequency_0 2.000314878 9,366,092 hv_24x7/pm_pb_cyc,chip=1/ # 2.3 GHz PowerBUS_Frequency_1 So, here _0 and _1 after PowerBUS_Frequency specify parameter value. Signed-off-by: Kajol Jain <kjain@linux.ibm.com> Acked-by: Jiri Olsa <jolsa@redhat.com> Cc: Alexander Shishkin <alexander.shishkin@linux.intel.com> Cc: Andi Kleen <ak@linux.intel.com> Cc: Anju T Sudhakar <anju@linux.vnet.ibm.com> Cc: Benjamin Herrenschmidt <benh@kernel.crashing.org> Cc: Greg Kroah-Hartman <gregkh@linuxfoundation.org> Cc: Jin Yao <yao.jin@linux.intel.com> Cc: Joe Mario <jmario@redhat.com> Cc: Kan Liang <kan.liang@linux.intel.com> Cc: Madhavan Srinivasan <maddy@linux.vnet.ibm.com> Cc: Mamatha Inamdar <mamatha4@linux.vnet.ibm.com> Cc: Mark Rutland <mark.rutland@arm.com> Cc: Michael Ellerman <mpe@ellerman.id.au> Cc: Michael Petlan <mpetlan@redhat.com> Cc: Namhyung Kim <namhyung@kernel.org> Cc: Paul Mackerras <paulus@ozlabs.org> Cc: Peter Zijlstra <peterz@infradead.org> Cc: Ravi Bangoria <ravi.bangoria@linux.ibm.com> Cc: Sukadev Bhattiprolu <sukadev@linux.vnet.ibm.com> Cc: Thomas Gleixner <tglx@linutronix.de> Cc: linuxppc-dev@lists.ozlabs.org Link: http://lore.kernel.org/lkml/20200401203340.31402-5-kjain@linux.ibm.com Signed-off-by: Arnaldo Carvalho de Melo <acme@redhat.com>
127 lines
2.3 KiB
Text
127 lines
2.3 KiB
Text
%option prefix="expr_"
|
|
%option reentrant
|
|
%option bison-bridge
|
|
|
|
%{
|
|
#include <linux/compiler.h>
|
|
#include "expr.h"
|
|
#include "expr-bison.h"
|
|
|
|
char *expr_get_text(yyscan_t yyscanner);
|
|
YYSTYPE *expr_get_lval(yyscan_t yyscanner);
|
|
|
|
static int __value(YYSTYPE *yylval, char *str, int base, int token)
|
|
{
|
|
u64 num;
|
|
|
|
errno = 0;
|
|
num = strtoull(str, NULL, base);
|
|
if (errno)
|
|
return EXPR_ERROR;
|
|
|
|
yylval->num = num;
|
|
return token;
|
|
}
|
|
|
|
static int value(yyscan_t scanner, int base)
|
|
{
|
|
YYSTYPE *yylval = expr_get_lval(scanner);
|
|
char *text = expr_get_text(scanner);
|
|
|
|
return __value(yylval, text, base, NUMBER);
|
|
}
|
|
|
|
/*
|
|
* Allow @ instead of / to be able to specify pmu/event/ without
|
|
* conflicts with normal division.
|
|
*/
|
|
static char *normalize(char *str, int runtime)
|
|
{
|
|
char *ret = str;
|
|
char *dst = str;
|
|
|
|
while (*str) {
|
|
if (*str == '@')
|
|
*dst++ = '/';
|
|
else if (*str == '\\')
|
|
*dst++ = *++str;
|
|
else if (*str == '?') {
|
|
char *paramval;
|
|
int i = 0;
|
|
int size = asprintf(¶mval, "%d", runtime);
|
|
|
|
if (size < 0)
|
|
*dst++ = '0';
|
|
else {
|
|
while (i < size)
|
|
*dst++ = paramval[i++];
|
|
free(paramval);
|
|
}
|
|
}
|
|
else
|
|
*dst++ = *str;
|
|
str++;
|
|
}
|
|
|
|
*dst = 0x0;
|
|
return ret;
|
|
}
|
|
|
|
static int str(yyscan_t scanner, int token, int runtime)
|
|
{
|
|
YYSTYPE *yylval = expr_get_lval(scanner);
|
|
char *text = expr_get_text(scanner);
|
|
|
|
yylval->str = normalize(strdup(text), runtime);
|
|
if (!yylval->str)
|
|
return EXPR_ERROR;
|
|
|
|
yylval->str = normalize(yylval->str, runtime);
|
|
return token;
|
|
}
|
|
%}
|
|
|
|
number [0-9]+
|
|
|
|
sch [-,=]
|
|
spec \\{sch}
|
|
sym [0-9a-zA-Z_\.:@?]+
|
|
symbol {spec}*{sym}*{spec}*{sym}*{spec}*{sym}
|
|
|
|
%%
|
|
struct expr_scanner_ctx *sctx = expr_get_extra(yyscanner);
|
|
|
|
{
|
|
int start_token = sctx->start_token;
|
|
|
|
if (sctx->start_token) {
|
|
sctx->start_token = 0;
|
|
return start_token;
|
|
}
|
|
}
|
|
|
|
max { return MAX; }
|
|
min { return MIN; }
|
|
if { return IF; }
|
|
else { return ELSE; }
|
|
#smt_on { return SMT_ON; }
|
|
{number} { return value(yyscanner, 10); }
|
|
{symbol} { return str(yyscanner, ID, sctx->runtime); }
|
|
"|" { return '|'; }
|
|
"^" { return '^'; }
|
|
"&" { return '&'; }
|
|
"-" { return '-'; }
|
|
"+" { return '+'; }
|
|
"*" { return '*'; }
|
|
"/" { return '/'; }
|
|
"%" { return '%'; }
|
|
"(" { return '('; }
|
|
")" { return ')'; }
|
|
"," { return ','; }
|
|
. { }
|
|
%%
|
|
|
|
int expr_wrap(void *scanner __maybe_unused)
|
|
{
|
|
return 1;
|
|
}
|