mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-13 14:14:37 +00:00
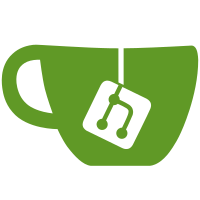
For the individual CCU drivers to be built as modules, the ops structs, helper functions, and callback registration functions must be exported. These symbols are intended for use only by the adjacent CCU drivers, so export them into the SUNXI_CCU namespace. of_sunxi_ccu_probe is not exported because it is only used by built-in OF clock providers. Signed-off-by: Samuel Holland <samuel@sholland.org> Signed-off-by: Maxime Ripard <maxime@cerno.tech> Link: https://lore.kernel.org/r/20211119033338.25486-2-samuel@sholland.org
78 lines
1.8 KiB
C
78 lines
1.8 KiB
C
// SPDX-License-Identifier: GPL-2.0-or-later
|
|
/*
|
|
* Copyright (C) 2016 Maxime Ripard
|
|
* Maxime Ripard <maxime.ripard@free-electrons.com>
|
|
*/
|
|
|
|
#include <linux/delay.h>
|
|
#include <linux/io.h>
|
|
#include <linux/reset-controller.h>
|
|
|
|
#include "ccu_reset.h"
|
|
|
|
static int ccu_reset_assert(struct reset_controller_dev *rcdev,
|
|
unsigned long id)
|
|
{
|
|
struct ccu_reset *ccu = rcdev_to_ccu_reset(rcdev);
|
|
const struct ccu_reset_map *map = &ccu->reset_map[id];
|
|
unsigned long flags;
|
|
u32 reg;
|
|
|
|
spin_lock_irqsave(ccu->lock, flags);
|
|
|
|
reg = readl(ccu->base + map->reg);
|
|
writel(reg & ~map->bit, ccu->base + map->reg);
|
|
|
|
spin_unlock_irqrestore(ccu->lock, flags);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int ccu_reset_deassert(struct reset_controller_dev *rcdev,
|
|
unsigned long id)
|
|
{
|
|
struct ccu_reset *ccu = rcdev_to_ccu_reset(rcdev);
|
|
const struct ccu_reset_map *map = &ccu->reset_map[id];
|
|
unsigned long flags;
|
|
u32 reg;
|
|
|
|
spin_lock_irqsave(ccu->lock, flags);
|
|
|
|
reg = readl(ccu->base + map->reg);
|
|
writel(reg | map->bit, ccu->base + map->reg);
|
|
|
|
spin_unlock_irqrestore(ccu->lock, flags);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int ccu_reset_reset(struct reset_controller_dev *rcdev,
|
|
unsigned long id)
|
|
{
|
|
ccu_reset_assert(rcdev, id);
|
|
udelay(10);
|
|
ccu_reset_deassert(rcdev, id);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int ccu_reset_status(struct reset_controller_dev *rcdev,
|
|
unsigned long id)
|
|
{
|
|
struct ccu_reset *ccu = rcdev_to_ccu_reset(rcdev);
|
|
const struct ccu_reset_map *map = &ccu->reset_map[id];
|
|
|
|
/*
|
|
* The reset control API expects 0 if reset is not asserted,
|
|
* which is the opposite of what our hardware uses.
|
|
*/
|
|
return !(map->bit & readl(ccu->base + map->reg));
|
|
}
|
|
|
|
const struct reset_control_ops ccu_reset_ops = {
|
|
.assert = ccu_reset_assert,
|
|
.deassert = ccu_reset_deassert,
|
|
.reset = ccu_reset_reset,
|
|
.status = ccu_reset_status,
|
|
};
|
|
EXPORT_SYMBOL_NS_GPL(ccu_reset_ops, SUNXI_CCU);
|