mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-24 01:41:39 +00:00
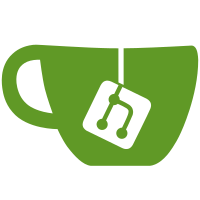
Perf shows we're spending a lot of time in find_insn() and the statistics show we have around 3.2 million instruction. Increase the hash table size to reduce the bucket load from around 50 to 3. This shaves about 2s off of objtool on vmlinux.o runtime, down to 16s. Signed-off-by: Peter Zijlstra (Intel) <peterz@infradead.org> Reviewed-by: Miroslav Benes <mbenes@suse.cz> Acked-by: Josh Poimboeuf <jpoimboe@redhat.com> Link: https://lkml.kernel.org/r/20200324160924.617882545@infradead.org
72 lines
1.7 KiB
C
72 lines
1.7 KiB
C
/* SPDX-License-Identifier: GPL-2.0-or-later */
|
|
/*
|
|
* Copyright (C) 2017 Josh Poimboeuf <jpoimboe@redhat.com>
|
|
*/
|
|
|
|
#ifndef _CHECK_H
|
|
#define _CHECK_H
|
|
|
|
#include <stdbool.h>
|
|
#include "elf.h"
|
|
#include "cfi.h"
|
|
#include "arch.h"
|
|
#include "orc.h"
|
|
#include <linux/hashtable.h>
|
|
|
|
struct insn_state {
|
|
struct cfi_reg cfa;
|
|
struct cfi_reg regs[CFI_NUM_REGS];
|
|
int stack_size;
|
|
unsigned char type;
|
|
bool bp_scratch;
|
|
bool drap, end, uaccess, df;
|
|
unsigned int uaccess_stack;
|
|
int drap_reg, drap_offset;
|
|
struct cfi_reg vals[CFI_NUM_REGS];
|
|
};
|
|
|
|
struct instruction {
|
|
struct list_head list;
|
|
struct hlist_node hash;
|
|
struct section *sec;
|
|
unsigned long offset;
|
|
unsigned int len;
|
|
enum insn_type type;
|
|
unsigned long immediate;
|
|
bool alt_group, dead_end, ignore, hint, save, restore, ignore_alts;
|
|
bool retpoline_safe;
|
|
u8 visited;
|
|
struct symbol *call_dest;
|
|
struct instruction *jump_dest;
|
|
struct instruction *first_jump_src;
|
|
struct rela *jump_table;
|
|
struct list_head alts;
|
|
struct symbol *func;
|
|
struct stack_op stack_op;
|
|
struct insn_state state;
|
|
struct orc_entry orc;
|
|
};
|
|
|
|
struct objtool_file {
|
|
struct elf *elf;
|
|
struct list_head insn_list;
|
|
DECLARE_HASHTABLE(insn_hash, 20);
|
|
bool ignore_unreachables, c_file, hints, rodata;
|
|
};
|
|
|
|
int check(const char *objname, bool orc);
|
|
|
|
struct instruction *find_insn(struct objtool_file *file,
|
|
struct section *sec, unsigned long offset);
|
|
|
|
#define for_each_insn(file, insn) \
|
|
list_for_each_entry(insn, &file->insn_list, list)
|
|
|
|
#define sec_for_each_insn(file, sec, insn) \
|
|
for (insn = find_insn(file, sec, 0); \
|
|
insn && &insn->list != &file->insn_list && \
|
|
insn->sec == sec; \
|
|
insn = list_next_entry(insn, list))
|
|
|
|
|
|
#endif /* _CHECK_H */
|