mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 00:48:50 +00:00
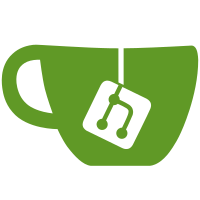
Add the `static_assert!` macro, which is a compile-time assert, similar to the C11 `_Static_assert` and C++11 `static_assert` declarations [1,2]. Do so in a new module, called `static_assert`. For instance: static_assert!(42 > 24); static_assert!(core::mem::size_of::<u8>() == 1); const X: &[u8] = b"bar"; static_assert!(X[1] == b'a'); const fn f(x: i32) -> i32 { x + 2 } static_assert!(f(40) == 42); Link: https://en.cppreference.com/w/c/language/_Static_assert [1] Link: https://en.cppreference.com/w/cpp/language/static_assert [2] Co-developed-by: Alex Gaynor <alex.gaynor@gmail.com> Signed-off-by: Alex Gaynor <alex.gaynor@gmail.com> Signed-off-by: Miguel Ojeda <ojeda@kernel.org>
34 lines
879 B
Rust
34 lines
879 B
Rust
// SPDX-License-Identifier: GPL-2.0
|
|
|
|
//! Static assert.
|
|
|
|
/// Static assert (i.e. compile-time assert).
|
|
///
|
|
/// Similar to C11 [`_Static_assert`] and C++11 [`static_assert`].
|
|
///
|
|
/// The feature may be added to Rust in the future: see [RFC 2790].
|
|
///
|
|
/// [`_Static_assert`]: https://en.cppreference.com/w/c/language/_Static_assert
|
|
/// [`static_assert`]: https://en.cppreference.com/w/cpp/language/static_assert
|
|
/// [RFC 2790]: https://github.com/rust-lang/rfcs/issues/2790
|
|
///
|
|
/// # Examples
|
|
///
|
|
/// ```
|
|
/// static_assert!(42 > 24);
|
|
/// static_assert!(core::mem::size_of::<u8>() == 1);
|
|
///
|
|
/// const X: &[u8] = b"bar";
|
|
/// static_assert!(X[1] == b'a');
|
|
///
|
|
/// const fn f(x: i32) -> i32 {
|
|
/// x + 2
|
|
/// }
|
|
/// static_assert!(f(40) == 42);
|
|
/// ```
|
|
#[macro_export]
|
|
macro_rules! static_assert {
|
|
($condition:expr) => {
|
|
const _: () = core::assert!($condition);
|
|
};
|
|
}
|