mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 00:48:50 +00:00
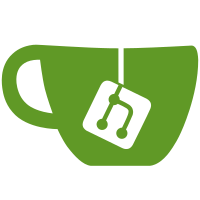
Add SGX1 and SGX2 feature flags, via CPUID.0x12.0x0.EAX, as scattered features, since adding a new leaf for only two bits would be wasteful. As part of virtualizing SGX, KVM will expose the SGX CPUID leafs to its guest, and to do so correctly needs to query hardware and kernel support for SGX1 and SGX2. Suppress both SGX1 and SGX2 from /proc/cpuinfo. SGX1 basically means SGX, and for SGX2 there is no concrete use case of using it in /proc/cpuinfo. Signed-off-by: Sean Christopherson <seanjc@google.com> Signed-off-by: Kai Huang <kai.huang@intel.com> Signed-off-by: Borislav Petkov <bp@suse.de> Acked-by: Dave Hansen <dave.hansen@intel.com> Acked-by: Jarkko Sakkinen <jarkko@kernel.org> Link: https://lkml.kernel.org/r/d787827dbfca6b3210ac3e432e3ac1202727e786.1616136308.git.kai.huang@intel.com
69 lines
2.1 KiB
C
69 lines
2.1 KiB
C
/*
|
|
* Routines to identify additional cpu features that are scattered in
|
|
* cpuid space.
|
|
*/
|
|
#include <linux/cpu.h>
|
|
|
|
#include <asm/memtype.h>
|
|
#include <asm/apic.h>
|
|
#include <asm/processor.h>
|
|
|
|
#include "cpu.h"
|
|
|
|
struct cpuid_bit {
|
|
u16 feature;
|
|
u8 reg;
|
|
u8 bit;
|
|
u32 level;
|
|
u32 sub_leaf;
|
|
};
|
|
|
|
/*
|
|
* Please keep the leaf sorted by cpuid_bit.level for faster search.
|
|
* X86_FEATURE_MBA is supported by both Intel and AMD. But the CPUID
|
|
* levels are different and there is a separate entry for each.
|
|
*/
|
|
static const struct cpuid_bit cpuid_bits[] = {
|
|
{ X86_FEATURE_APERFMPERF, CPUID_ECX, 0, 0x00000006, 0 },
|
|
{ X86_FEATURE_EPB, CPUID_ECX, 3, 0x00000006, 0 },
|
|
{ X86_FEATURE_CQM_LLC, CPUID_EDX, 1, 0x0000000f, 0 },
|
|
{ X86_FEATURE_CQM_OCCUP_LLC, CPUID_EDX, 0, 0x0000000f, 1 },
|
|
{ X86_FEATURE_CQM_MBM_TOTAL, CPUID_EDX, 1, 0x0000000f, 1 },
|
|
{ X86_FEATURE_CQM_MBM_LOCAL, CPUID_EDX, 2, 0x0000000f, 1 },
|
|
{ X86_FEATURE_CAT_L3, CPUID_EBX, 1, 0x00000010, 0 },
|
|
{ X86_FEATURE_CAT_L2, CPUID_EBX, 2, 0x00000010, 0 },
|
|
{ X86_FEATURE_CDP_L3, CPUID_ECX, 2, 0x00000010, 1 },
|
|
{ X86_FEATURE_CDP_L2, CPUID_ECX, 2, 0x00000010, 2 },
|
|
{ X86_FEATURE_MBA, CPUID_EBX, 3, 0x00000010, 0 },
|
|
{ X86_FEATURE_PER_THREAD_MBA, CPUID_ECX, 0, 0x00000010, 3 },
|
|
{ X86_FEATURE_SGX1, CPUID_EAX, 0, 0x00000012, 0 },
|
|
{ X86_FEATURE_SGX2, CPUID_EAX, 1, 0x00000012, 0 },
|
|
{ X86_FEATURE_HW_PSTATE, CPUID_EDX, 7, 0x80000007, 0 },
|
|
{ X86_FEATURE_CPB, CPUID_EDX, 9, 0x80000007, 0 },
|
|
{ X86_FEATURE_PROC_FEEDBACK, CPUID_EDX, 11, 0x80000007, 0 },
|
|
{ X86_FEATURE_MBA, CPUID_EBX, 6, 0x80000008, 0 },
|
|
{ 0, 0, 0, 0, 0 }
|
|
};
|
|
|
|
void init_scattered_cpuid_features(struct cpuinfo_x86 *c)
|
|
{
|
|
u32 max_level;
|
|
u32 regs[4];
|
|
const struct cpuid_bit *cb;
|
|
|
|
for (cb = cpuid_bits; cb->feature; cb++) {
|
|
|
|
/* Verify that the level is valid */
|
|
max_level = cpuid_eax(cb->level & 0xffff0000);
|
|
if (max_level < cb->level ||
|
|
max_level > (cb->level | 0xffff))
|
|
continue;
|
|
|
|
cpuid_count(cb->level, cb->sub_leaf, ®s[CPUID_EAX],
|
|
®s[CPUID_EBX], ®s[CPUID_ECX],
|
|
®s[CPUID_EDX]);
|
|
|
|
if (regs[cb->reg] & (1 << cb->bit))
|
|
set_cpu_cap(c, cb->feature);
|
|
}
|
|
}
|