mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-10-31 16:38:12 +00:00
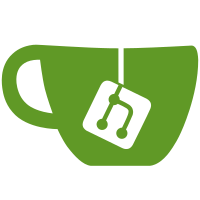
All OMAP IP blocks expect LE data, but CPU may operate in BE mode. Need to use endian neutral functions to read/write h/w registers. I.e instead of __raw_read[lw] and __raw_write[lw] functions code need to use read[lw]_relaxed and write[lw]_relaxed functions. If the first simply reads/writes register, the second will byteswap it if host operates in BE mode. Changes are trivial sed like replacement of __raw_xxx functions with xxx_relaxed variant. Signed-off-by: Victor Kamensky <victor.kamensky@linaro.org> Signed-off-by: Taras Kondratiuk <taras.kondratiuk@linaro.org> Signed-off-by: Tony Lindgren <tony@atomide.com>
62 lines
1.4 KiB
C
62 lines
1.4 KiB
C
/*
|
|
* OMAP4 PRCM_MPU module functions
|
|
*
|
|
* Copyright (C) 2009 Nokia Corporation
|
|
* Paul Walmsley
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License version 2 as
|
|
* published by the Free Software Foundation.
|
|
*/
|
|
|
|
#include <linux/kernel.h>
|
|
#include <linux/types.h>
|
|
#include <linux/errno.h>
|
|
#include <linux/err.h>
|
|
#include <linux/io.h>
|
|
|
|
#include "iomap.h"
|
|
#include "common.h"
|
|
#include "prcm_mpu44xx.h"
|
|
#include "cm-regbits-44xx.h"
|
|
|
|
/*
|
|
* prcm_mpu_base: the virtual address of the start of the PRCM_MPU IP
|
|
* block registers
|
|
*/
|
|
void __iomem *prcm_mpu_base;
|
|
|
|
/* PRCM_MPU low-level functions */
|
|
|
|
u32 omap4_prcm_mpu_read_inst_reg(s16 inst, u16 reg)
|
|
{
|
|
return readl_relaxed(OMAP44XX_PRCM_MPU_REGADDR(inst, reg));
|
|
}
|
|
|
|
void omap4_prcm_mpu_write_inst_reg(u32 val, s16 inst, u16 reg)
|
|
{
|
|
writel_relaxed(val, OMAP44XX_PRCM_MPU_REGADDR(inst, reg));
|
|
}
|
|
|
|
u32 omap4_prcm_mpu_rmw_inst_reg_bits(u32 mask, u32 bits, s16 inst, s16 reg)
|
|
{
|
|
u32 v;
|
|
|
|
v = omap4_prcm_mpu_read_inst_reg(inst, reg);
|
|
v &= ~mask;
|
|
v |= bits;
|
|
omap4_prcm_mpu_write_inst_reg(v, inst, reg);
|
|
|
|
return v;
|
|
}
|
|
|
|
/**
|
|
* omap2_set_globals_prcm_mpu - set the MPU PRCM base address (for early use)
|
|
* @prcm_mpu: PRCM_MPU base virtual address
|
|
*
|
|
* XXX Will be replaced when the PRM/CM drivers are completed.
|
|
*/
|
|
void __init omap2_set_globals_prcm_mpu(void __iomem *prcm_mpu)
|
|
{
|
|
prcm_mpu_base = prcm_mpu;
|
|
}
|