mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-21 16:29:59 +00:00
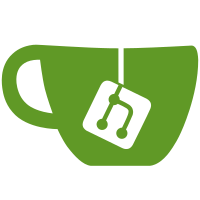
A sorted flow list is only needed to drop packets in the biggest flow when hitting the overmemory condition. By scanning flows only when needed, we can avoid paying the cost of maintaining the list under normal conditions In order to avoid scanning lots of empty flows and touching too many cold cache lines, a bitmap of flows with backlog is maintained Signed-off-by: Felix Fietkau <nbd@nbd.name> Link: https://lore.kernel.org/r/20201218184718.93650-3-nbd@nbd.name Signed-off-by: Johannes Berg <johannes.berg@intel.com>
102 lines
2.5 KiB
C
102 lines
2.5 KiB
C
/* SPDX-License-Identifier: GPL-2.0-only */
|
|
/*
|
|
* Copyright (c) 2016 Qualcomm Atheros, Inc
|
|
*
|
|
* Based on net/sched/sch_fq_codel.c
|
|
*/
|
|
#ifndef __NET_SCHED_FQ_H
|
|
#define __NET_SCHED_FQ_H
|
|
|
|
struct fq_tin;
|
|
|
|
/**
|
|
* struct fq_flow - per traffic flow queue
|
|
*
|
|
* @tin: owner of this flow. Used to manage collisions, i.e. when a packet
|
|
* hashes to an index which points to a flow that is already owned by a
|
|
* different tin the packet is destined to. In such case the implementer
|
|
* must provide a fallback flow
|
|
* @flowchain: can be linked to fq_tin's new_flows or old_flows. Used for DRR++
|
|
* (deficit round robin) based round robin queuing similar to the one
|
|
* found in net/sched/sch_fq_codel.c
|
|
* @queue: sk_buff queue to hold packets
|
|
* @backlog: number of bytes pending in the queue. The number of packets can be
|
|
* found in @queue.qlen
|
|
* @deficit: used for DRR++
|
|
*/
|
|
struct fq_flow {
|
|
struct fq_tin *tin;
|
|
struct list_head flowchain;
|
|
struct sk_buff_head queue;
|
|
u32 backlog;
|
|
int deficit;
|
|
};
|
|
|
|
/**
|
|
* struct fq_tin - a logical container of fq_flows
|
|
*
|
|
* Used to group fq_flows into a logical aggregate. DRR++ scheme is used to
|
|
* pull interleaved packets out of the associated flows.
|
|
*
|
|
* @new_flows: linked list of fq_flow
|
|
* @old_flows: linked list of fq_flow
|
|
*/
|
|
struct fq_tin {
|
|
struct list_head new_flows;
|
|
struct list_head old_flows;
|
|
struct list_head tin_list;
|
|
struct fq_flow default_flow;
|
|
u32 backlog_bytes;
|
|
u32 backlog_packets;
|
|
u32 overlimit;
|
|
u32 collisions;
|
|
u32 flows;
|
|
u32 tx_bytes;
|
|
u32 tx_packets;
|
|
};
|
|
|
|
/**
|
|
* struct fq - main container for fair queuing purposes
|
|
*
|
|
* @limit: max number of packets that can be queued across all flows
|
|
* @backlog: number of packets queued across all flows
|
|
*/
|
|
struct fq {
|
|
struct fq_flow *flows;
|
|
unsigned long *flows_bitmap;
|
|
|
|
struct list_head tin_backlog;
|
|
spinlock_t lock;
|
|
u32 flows_cnt;
|
|
u32 limit;
|
|
u32 memory_limit;
|
|
u32 memory_usage;
|
|
u32 quantum;
|
|
u32 backlog;
|
|
u32 overlimit;
|
|
u32 overmemory;
|
|
u32 collisions;
|
|
};
|
|
|
|
typedef struct sk_buff *fq_tin_dequeue_t(struct fq *,
|
|
struct fq_tin *,
|
|
struct fq_flow *flow);
|
|
|
|
typedef void fq_skb_free_t(struct fq *,
|
|
struct fq_tin *,
|
|
struct fq_flow *,
|
|
struct sk_buff *);
|
|
|
|
/* Return %true to filter (drop) the frame. */
|
|
typedef bool fq_skb_filter_t(struct fq *,
|
|
struct fq_tin *,
|
|
struct fq_flow *,
|
|
struct sk_buff *,
|
|
void *);
|
|
|
|
typedef struct fq_flow *fq_flow_get_default_t(struct fq *,
|
|
struct fq_tin *,
|
|
int idx,
|
|
struct sk_buff *);
|
|
|
|
#endif
|