mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 00:48:50 +00:00
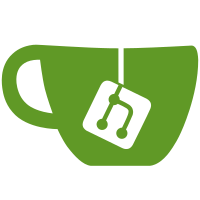
it shouldn't contain space letters and special letters like parentheses. aplay will be "Segmentation fault" without this patch. special thanks to Takashi. Signed-off-by: Kuninori Morimoto <kuninori.morimoto.gx@renesas.com> Acked-by: Liam Girdwood <lrg@ti.com> Signed-off-by: Mark Brown <broonie@opensource.wolfsonmicro.com>
79 lines
1.9 KiB
C
79 lines
1.9 KiB
C
/*
|
|
* fsi-da7210.c
|
|
*
|
|
* Copyright (C) 2009 Renesas Solutions Corp.
|
|
* Kuninori Morimoto <morimoto.kuninori@renesas.com>
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify it
|
|
* under the terms of the GNU General Public License as published by the
|
|
* Free Software Foundation; either version 2 of the License, or (at your
|
|
* option) any later version.
|
|
*/
|
|
|
|
#include <linux/platform_device.h>
|
|
#include <sound/sh_fsi.h>
|
|
|
|
static int fsi_da7210_init(struct snd_soc_pcm_runtime *rtd)
|
|
{
|
|
struct snd_soc_dai *codec = rtd->codec_dai;
|
|
struct snd_soc_dai *cpu = rtd->cpu_dai;
|
|
int ret;
|
|
|
|
ret = snd_soc_dai_set_fmt(codec,
|
|
SND_SOC_DAIFMT_I2S |
|
|
SND_SOC_DAIFMT_CBM_CFM);
|
|
if (ret < 0)
|
|
return ret;
|
|
|
|
ret = snd_soc_dai_set_fmt(cpu, SND_SOC_DAIFMT_I2S |
|
|
SND_SOC_DAIFMT_CBS_CFS);
|
|
|
|
return ret;
|
|
}
|
|
|
|
static struct snd_soc_dai_link fsi_da7210_dai = {
|
|
.name = "DA7210",
|
|
.stream_name = "DA7210",
|
|
.cpu_dai_name = "fsib-dai", /* FSI B */
|
|
.codec_dai_name = "da7210-hifi",
|
|
.platform_name = "sh_fsi.0",
|
|
.codec_name = "da7210-codec.0-001a",
|
|
.init = fsi_da7210_init,
|
|
};
|
|
|
|
static struct snd_soc_card fsi_soc_card = {
|
|
.name = "FSI-DA7210",
|
|
.dai_link = &fsi_da7210_dai,
|
|
.num_links = 1,
|
|
};
|
|
|
|
static struct platform_device *fsi_da7210_snd_device;
|
|
|
|
static int __init fsi_da7210_sound_init(void)
|
|
{
|
|
int ret;
|
|
|
|
fsi_da7210_snd_device = platform_device_alloc("soc-audio", FSI_PORT_B);
|
|
if (!fsi_da7210_snd_device)
|
|
return -ENOMEM;
|
|
|
|
platform_set_drvdata(fsi_da7210_snd_device, &fsi_soc_card);
|
|
ret = platform_device_add(fsi_da7210_snd_device);
|
|
if (ret)
|
|
platform_device_put(fsi_da7210_snd_device);
|
|
|
|
return ret;
|
|
}
|
|
|
|
static void __exit fsi_da7210_sound_exit(void)
|
|
{
|
|
platform_device_unregister(fsi_da7210_snd_device);
|
|
}
|
|
|
|
module_init(fsi_da7210_sound_init);
|
|
module_exit(fsi_da7210_sound_exit);
|
|
|
|
/* Module information */
|
|
MODULE_DESCRIPTION("ALSA SoC FSI DA2710");
|
|
MODULE_AUTHOR("Kuninori Morimoto <morimoto.kuninori@renesas.com>");
|
|
MODULE_LICENSE("GPL");
|