mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-21 10:01:00 +00:00
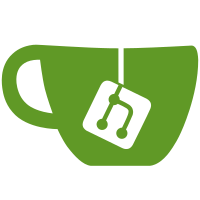
Some ARM platforms have the ability to program the interrupt controller to detect various interrupt edges and/or levels. For some platforms, this is critical to setup correctly, particularly those which the setting is dependent on the device. Currently, ARM drivers do (eg) the following: err = request_irq(irq, ...); set_irq_type(irq, IRQT_RISING); However, if the interrupt has previously been programmed to be level sensitive (for whatever reason) then this will cause an interrupt storm. Hence, if we combine set_irq_type() with request_irq(), we can then safely set the type prior to unmasking the interrupt. The unfortunate problem is that in order to support this, these flags need to be visible outside of the ARM architecture - drivers such as smc91x need these flags and they're cross-architecture. Finally, the SA_TRIGGER_* flag passed to request_irq() should reflect the property that the device would like. The IRQ controller code should do its best to select the most appropriate supported mode. Signed-off-by: Russell King <rmk+kernel@arm.linux.org.uk> Signed-off-by: Andrew Morton <akpm@osdl.org> Signed-off-by: Linus Torvalds <torvalds@osdl.org>
56 lines
1.2 KiB
C
56 lines
1.2 KiB
C
#ifndef __ASM_ARM_IRQ_H
|
|
#define __ASM_ARM_IRQ_H
|
|
|
|
#include <asm/arch/irqs.h>
|
|
|
|
#ifndef irq_canonicalize
|
|
#define irq_canonicalize(i) (i)
|
|
#endif
|
|
|
|
#ifndef NR_IRQS
|
|
#define NR_IRQS 128
|
|
#endif
|
|
|
|
/*
|
|
* Use this value to indicate lack of interrupt
|
|
* capability
|
|
*/
|
|
#ifndef NO_IRQ
|
|
#define NO_IRQ ((unsigned int)(-1))
|
|
#endif
|
|
|
|
struct irqaction;
|
|
|
|
extern void disable_irq_nosync(unsigned int);
|
|
extern void disable_irq(unsigned int);
|
|
extern void enable_irq(unsigned int);
|
|
|
|
/*
|
|
* These correspond with the SA_TRIGGER_* defines, and therefore the
|
|
* IRQRESOURCE_IRQ_* defines.
|
|
*/
|
|
#define __IRQT_RISEDGE (1 << 0)
|
|
#define __IRQT_FALEDGE (1 << 1)
|
|
#define __IRQT_HIGHLVL (1 << 2)
|
|
#define __IRQT_LOWLVL (1 << 3)
|
|
|
|
#define IRQT_NOEDGE (0)
|
|
#define IRQT_RISING (__IRQT_RISEDGE)
|
|
#define IRQT_FALLING (__IRQT_FALEDGE)
|
|
#define IRQT_BOTHEDGE (__IRQT_RISEDGE|__IRQT_FALEDGE)
|
|
#define IRQT_LOW (__IRQT_LOWLVL)
|
|
#define IRQT_HIGH (__IRQT_HIGHLVL)
|
|
#define IRQT_PROBE (1 << 4)
|
|
|
|
int set_irq_type(unsigned int irq, unsigned int type);
|
|
void disable_irq_wake(unsigned int irq);
|
|
void enable_irq_wake(unsigned int irq);
|
|
int setup_irq(unsigned int, struct irqaction *);
|
|
|
|
struct irqaction;
|
|
struct pt_regs;
|
|
int handle_IRQ_event(unsigned int, struct pt_regs *, struct irqaction *);
|
|
|
|
extern void migrate_irqs(void);
|
|
#endif
|
|
|