mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-10-31 16:38:12 +00:00
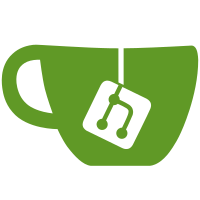
In preparation for removing HANDLE_DOMAIN_IRQ_IRQENTRY, have arch/openrisc perform all the irqentry accounting in its entry code. As arch/openrisc uses GENERIC_IRQ_MULTI_HANDLER, we can use generic_handle_arch_irq() to do so. There should be no functional change as a result of this patch. Signed-off-by: Mark Rutland <mark.rutland@arm.com> Reviewed-by: Marc Zyngier <maz@kernel.org> Reviewed-by: Stafford Horne <shorne@gmail.com> Cc: Jonas Bonn <jonas@southpole.se> Cc: Stefan Kristiansson <stefan.kristiansson@saunalahti.fi> Cc: Thomas Gleixner <tglx@linutronix.de>
38 lines
939 B
C
38 lines
939 B
C
// SPDX-License-Identifier: GPL-2.0-or-later
|
|
/*
|
|
* OpenRISC irq.c
|
|
*
|
|
* Linux architectural port borrowing liberally from similar works of
|
|
* others. All original copyrights apply as per the original source
|
|
* declaration.
|
|
*
|
|
* Modifications for the OpenRISC architecture:
|
|
* Copyright (C) 2010-2011 Jonas Bonn <jonas@southpole.se>
|
|
*/
|
|
|
|
#include <linux/interrupt.h>
|
|
#include <linux/init.h>
|
|
#include <linux/ftrace.h>
|
|
#include <linux/irq.h>
|
|
#include <linux/irqchip.h>
|
|
#include <linux/export.h>
|
|
#include <linux/irqflags.h>
|
|
|
|
/* read interrupt enabled status */
|
|
unsigned long arch_local_save_flags(void)
|
|
{
|
|
return mfspr(SPR_SR) & (SPR_SR_IEE|SPR_SR_TEE);
|
|
}
|
|
EXPORT_SYMBOL(arch_local_save_flags);
|
|
|
|
/* set interrupt enabled status */
|
|
void arch_local_irq_restore(unsigned long flags)
|
|
{
|
|
mtspr(SPR_SR, ((mfspr(SPR_SR) & ~(SPR_SR_IEE|SPR_SR_TEE)) | flags));
|
|
}
|
|
EXPORT_SYMBOL(arch_local_irq_restore);
|
|
|
|
void __init init_IRQ(void)
|
|
{
|
|
irqchip_init();
|
|
}
|