mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-23 01:10:04 +00:00
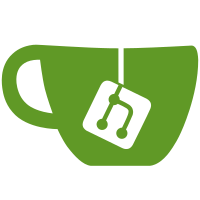
module_layout manages different types of memory (text, data, rodata, etc.) in one allocation, which is problematic for some reasons: 1. It is hard to enable CONFIG_STRICT_MODULE_RWX. 2. It is hard to use huge pages in modules (and not break strict rwx). 3. Many archs uses module_layout for arch-specific data, but it is not obvious how these data are used (are they RO, RX, or RW?) Improve the scenario by replacing 2 (or 3) module_layout per module with up to 7 module_memory per module: MOD_TEXT, MOD_DATA, MOD_RODATA, MOD_RO_AFTER_INIT, MOD_INIT_TEXT, MOD_INIT_DATA, MOD_INIT_RODATA, and allocating them separately. This adds slightly more entries to mod_tree (from up to 3 entries per module, to up to 7 entries per module). However, this at most adds a small constant overhead to __module_address(), which is expected to be fast. Various archs use module_layout for different data. These data are put into different module_memory based on their location in module_layout. IOW, data that used to go with text is allocated with MOD_MEM_TYPE_TEXT; data that used to go with data is allocated with MOD_MEM_TYPE_DATA, etc. module_memory simplifies quite some of the module code. For example, ARCH_WANTS_MODULES_DATA_IN_VMALLOC is a lot cleaner, as it just uses a different allocator for the data. kernel/module/strict_rwx.c is also much cleaner with module_memory. Signed-off-by: Song Liu <song@kernel.org> Cc: Luis Chamberlain <mcgrof@kernel.org> Cc: Thomas Gleixner <tglx@linutronix.de> Cc: Peter Zijlstra <peterz@infradead.org> Cc: Guenter Roeck <linux@roeck-us.net> Cc: Christophe Leroy <christophe.leroy@csgroup.eu> Reviewed-by: Thomas Gleixner <tglx@linutronix.de> Reviewed-by: Christophe Leroy <christophe.leroy@csgroup.eu> Reviewed-by: Luis Chamberlain <mcgrof@kernel.org> Signed-off-by: Luis Chamberlain <mcgrof@kernel.org>
63 lines
1.5 KiB
C
63 lines
1.5 KiB
C
// SPDX-License-Identifier: GPL-2.0-or-later
|
|
/*
|
|
* Module kdb support
|
|
*
|
|
* Copyright (C) 2010 Jason Wessel
|
|
*/
|
|
|
|
#include <linux/module.h>
|
|
#include <linux/kdb.h>
|
|
#include "internal.h"
|
|
|
|
/*
|
|
* kdb_lsmod - This function implements the 'lsmod' command. Lists
|
|
* currently loaded kernel modules.
|
|
* Mostly taken from userland lsmod.
|
|
*/
|
|
int kdb_lsmod(int argc, const char **argv)
|
|
{
|
|
struct module *mod;
|
|
|
|
if (argc != 0)
|
|
return KDB_ARGCOUNT;
|
|
|
|
kdb_printf("Module Size modstruct Used by\n");
|
|
list_for_each_entry(mod, &modules, list) {
|
|
if (mod->state == MODULE_STATE_UNFORMED)
|
|
continue;
|
|
|
|
kdb_printf("%-20s%8u", mod->name, mod->mem[MOD_TEXT].size);
|
|
kdb_printf("/%8u", mod->mem[MOD_RODATA].size);
|
|
kdb_printf("/%8u", mod->mem[MOD_RO_AFTER_INIT].size);
|
|
kdb_printf("/%8u", mod->mem[MOD_DATA].size);
|
|
|
|
kdb_printf(" 0x%px ", (void *)mod);
|
|
#ifdef CONFIG_MODULE_UNLOAD
|
|
kdb_printf("%4d ", module_refcount(mod));
|
|
#endif
|
|
if (mod->state == MODULE_STATE_GOING)
|
|
kdb_printf(" (Unloading)");
|
|
else if (mod->state == MODULE_STATE_COMING)
|
|
kdb_printf(" (Loading)");
|
|
else
|
|
kdb_printf(" (Live)");
|
|
kdb_printf(" 0x%px", mod->mem[MOD_TEXT].base);
|
|
kdb_printf("/0x%px", mod->mem[MOD_RODATA].base);
|
|
kdb_printf("/0x%px", mod->mem[MOD_RO_AFTER_INIT].base);
|
|
kdb_printf("/0x%px", mod->mem[MOD_DATA].base);
|
|
|
|
#ifdef CONFIG_MODULE_UNLOAD
|
|
{
|
|
struct module_use *use;
|
|
|
|
kdb_printf(" [ ");
|
|
list_for_each_entry(use, &mod->source_list,
|
|
source_list)
|
|
kdb_printf("%s ", use->target->name);
|
|
kdb_printf("]\n");
|
|
}
|
|
#endif
|
|
}
|
|
|
|
return 0;
|
|
}
|