mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-10-02 15:18:19 +00:00
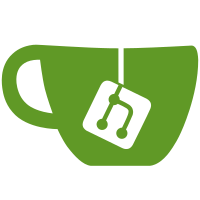
Enabling MSR[EE] in interrupt handlers while interrupts are still soft masked allows PMIs to profile interrupt handlers to some degree, beyond what SIAR latching allows. When perf is not being used, this is almost useless work. It requires an extra mtmsrd in the irq handler, and it also opens the door to masked interrupts hitting and requiring replay, which is more expensive than just taking them directly. This effect can be noticable in high IRQ workloads. Avoid enabling MSR[EE] unless perf is currently in use. This saves about 60 cycles (or 8%) on a simple decrementer interrupt microbenchmark. Replayed interrupts drop from 1.4% of all interrupts taken, to 0.003%. This does prevent the soft-nmi interrupt being taken in these handlers, but that's not too reliable anyway. The SMP watchdog will continue to be the reliable way to catch lockups. Signed-off-by: Nicholas Piggin <npiggin@gmail.com> Signed-off-by: Michael Ellerman <mpe@ellerman.id.au> Link: https://lore.kernel.org/r/20210922145452.352571-5-npiggin@gmail.com
47 lines
1,002 B
C
47 lines
1,002 B
C
// SPDX-License-Identifier: GPL-2.0-or-later
|
|
/*
|
|
* Author: Kumar Gala <galak@kernel.crashing.org>
|
|
*
|
|
* Copyright 2009 Freescale Semiconductor Inc.
|
|
*/
|
|
|
|
#include <linux/stddef.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/smp.h>
|
|
#include <linux/threads.h>
|
|
#include <linux/hardirq.h>
|
|
|
|
#include <asm/dbell.h>
|
|
#include <asm/interrupt.h>
|
|
#include <asm/irq_regs.h>
|
|
#include <asm/kvm_ppc.h>
|
|
#include <asm/trace.h>
|
|
|
|
#ifdef CONFIG_SMP
|
|
|
|
DEFINE_INTERRUPT_HANDLER_ASYNC(doorbell_exception)
|
|
{
|
|
struct pt_regs *old_regs = set_irq_regs(regs);
|
|
|
|
trace_doorbell_entry(regs);
|
|
|
|
ppc_msgsync();
|
|
|
|
if (should_hard_irq_enable())
|
|
do_hard_irq_enable();
|
|
|
|
kvmppc_clear_host_ipi(smp_processor_id());
|
|
__this_cpu_inc(irq_stat.doorbell_irqs);
|
|
|
|
smp_ipi_demux_relaxed(); /* already performed the barrier */
|
|
|
|
trace_doorbell_exit(regs);
|
|
|
|
set_irq_regs(old_regs);
|
|
}
|
|
#else /* CONFIG_SMP */
|
|
DEFINE_INTERRUPT_HANDLER_ASYNC(doorbell_exception)
|
|
{
|
|
printk(KERN_WARNING "Received doorbell on non-smp system\n");
|
|
}
|
|
#endif /* CONFIG_SMP */
|