mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-20 09:31:09 +00:00
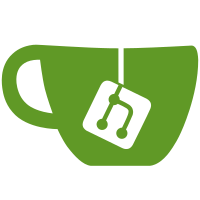
A client in the SOF (Sound Open Firmware) context is a driver that needs to communicate with the DSP via IPC messages. The SOF core is responsible for serializing the IPC messages to the DSP from the different clients. One example of an SOF client would be an IPC test client that floods the DSP with test IPC messages to validate if the serialization works as expected. Multi-client support will also add the ability to split the existing audio cards into multiple ones, so as to e.g. to deal with HDMI with a dedicated client instead of adding HDMI to all cards. This patch introduces descriptors for SOF client driver and SOF client device along with APIs for registering and unregistering a SOF client driver, sending IPCs from a client device and accessing the SOF core debugfs root entry. Along with this, add a couple of new members to struct snd_sof_dev that will be used for maintaining the list of clients. Signed-off-by: Ranjani Sridharan <ranjani.sridharan@linux.intel.com> Co-developed-by: Fred Oh <fred.oh@linux.intel.com> Signed-off-by: Fred Oh <fred.oh@linux.intel.com> Signed-off-by: Peter Ujfalusi <peter.ujfalusi@linux.intel.com> Reviewed-by: Kai Vehmanen <kai.vehmanen@linux.intel.com> Reviewed-by: Pierre-Louis Bossart <pierre-louis.bossart@linux.intel.com> Reviewed-by: Ranjani Sridharan <ranjani.sridharan@linux.intel.com> Link: https://lore.kernel.org/r/20220210150525.30756-6-peter.ujfalusi@linux.intel.com Signed-off-by: Mark Brown <broonie@kernel.org>
67 lines
2.2 KiB
C
67 lines
2.2 KiB
C
/* SPDX-License-Identifier: GPL-2.0-only */
|
|
|
|
#ifndef __SOC_SOF_CLIENT_H
|
|
#define __SOC_SOF_CLIENT_H
|
|
|
|
#include <linux/auxiliary_bus.h>
|
|
#include <linux/device.h>
|
|
#include <linux/list.h>
|
|
#include <sound/sof.h>
|
|
|
|
struct sof_ipc_fw_version;
|
|
struct sof_ipc_cmd_hdr;
|
|
struct snd_sof_dev;
|
|
struct dentry;
|
|
|
|
/**
|
|
* struct sof_client_dev - SOF client device
|
|
* @auxdev: auxiliary device
|
|
* @sdev: pointer to SOF core device struct
|
|
* @list: item in SOF core client dev list
|
|
* @data: device specific data
|
|
*/
|
|
struct sof_client_dev {
|
|
struct auxiliary_device auxdev;
|
|
struct snd_sof_dev *sdev;
|
|
struct list_head list;
|
|
void *data;
|
|
};
|
|
|
|
#define sof_client_dev_to_sof_dev(cdev) ((cdev)->sdev)
|
|
|
|
#define auxiliary_dev_to_sof_client_dev(auxiliary_dev) \
|
|
container_of(auxiliary_dev, struct sof_client_dev, auxdev)
|
|
|
|
#define dev_to_sof_client_dev(dev) \
|
|
container_of(to_auxiliary_dev(dev), struct sof_client_dev, auxdev)
|
|
|
|
int sof_client_ipc_tx_message(struct sof_client_dev *cdev, void *ipc_msg,
|
|
void *reply_data, size_t reply_bytes);
|
|
|
|
struct dentry *sof_client_get_debugfs_root(struct sof_client_dev *cdev);
|
|
struct device *sof_client_get_dma_dev(struct sof_client_dev *cdev);
|
|
const struct sof_ipc_fw_version *sof_client_get_fw_version(struct sof_client_dev *cdev);
|
|
|
|
/* module refcount management of SOF core */
|
|
int sof_client_core_module_get(struct sof_client_dev *cdev);
|
|
void sof_client_core_module_put(struct sof_client_dev *cdev);
|
|
|
|
/* IPC notification */
|
|
typedef void (*sof_client_event_callback)(struct sof_client_dev *cdev, void *msg_buf);
|
|
|
|
int sof_client_register_ipc_rx_handler(struct sof_client_dev *cdev,
|
|
u32 ipc_msg_type,
|
|
sof_client_event_callback callback);
|
|
void sof_client_unregister_ipc_rx_handler(struct sof_client_dev *cdev,
|
|
u32 ipc_msg_type);
|
|
|
|
/* DSP state notification and query */
|
|
typedef void (*sof_client_fw_state_callback)(struct sof_client_dev *cdev,
|
|
enum sof_fw_state state);
|
|
|
|
int sof_client_register_fw_state_handler(struct sof_client_dev *cdev,
|
|
sof_client_fw_state_callback callback);
|
|
void sof_client_unregister_fw_state_handler(struct sof_client_dev *cdev);
|
|
enum sof_fw_state sof_client_get_fw_state(struct sof_client_dev *cdev);
|
|
|
|
#endif /* __SOC_SOF_CLIENT_H */
|