mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-10-31 16:38:12 +00:00
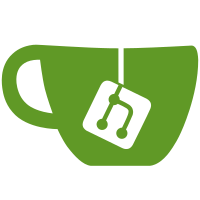
Allocation functions that return virtual addresses (with an exception of _raw variant) clear the allocated memory after reserving it. This requires valid memory ranges in memblock.memory. Introduce memory_block variable to store memory that can be registered with memblock data structure. Move assert.h and size.h includes to common.h to share them between the test files. Signed-off-by: Karolina Drobnik <karolinadrobnik@gmail.com> Signed-off-by: Mike Rapoport <rppt@linux.ibm.com> Link: https://lore.kernel.org/r/dce115503c74a6936c44694b00014658a1bb6522.1646055639.git.karolinadrobnik@gmail.com
48 lines
1.2 KiB
C
48 lines
1.2 KiB
C
// SPDX-License-Identifier: GPL-2.0-or-later
|
|
#include "tests/common.h"
|
|
#include <string.h>
|
|
|
|
#define INIT_MEMBLOCK_REGIONS 128
|
|
#define INIT_MEMBLOCK_RESERVED_REGIONS INIT_MEMBLOCK_REGIONS
|
|
|
|
static struct test_memory memory_block;
|
|
|
|
void reset_memblock_regions(void)
|
|
{
|
|
memset(memblock.memory.regions, 0,
|
|
memblock.memory.cnt * sizeof(struct memblock_region));
|
|
memblock.memory.cnt = 1;
|
|
memblock.memory.max = INIT_MEMBLOCK_REGIONS;
|
|
memblock.memory.total_size = 0;
|
|
|
|
memset(memblock.reserved.regions, 0,
|
|
memblock.reserved.cnt * sizeof(struct memblock_region));
|
|
memblock.reserved.cnt = 1;
|
|
memblock.reserved.max = INIT_MEMBLOCK_RESERVED_REGIONS;
|
|
memblock.reserved.total_size = 0;
|
|
}
|
|
|
|
void reset_memblock_attributes(void)
|
|
{
|
|
memblock.memory.name = "memory";
|
|
memblock.reserved.name = "reserved";
|
|
memblock.bottom_up = false;
|
|
memblock.current_limit = MEMBLOCK_ALLOC_ANYWHERE;
|
|
}
|
|
|
|
void setup_memblock(void)
|
|
{
|
|
reset_memblock_regions();
|
|
memblock_add((phys_addr_t)memory_block.base, MEM_SIZE);
|
|
}
|
|
|
|
void dummy_physical_memory_init(void)
|
|
{
|
|
memory_block.base = malloc(MEM_SIZE);
|
|
assert(memory_block.base);
|
|
}
|
|
|
|
void dummy_physical_memory_cleanup(void)
|
|
{
|
|
free(memory_block.base);
|
|
}
|