mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-22 08:49:45 +00:00
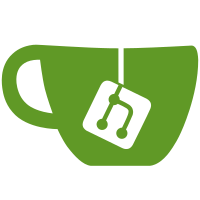
In the current scheme, on submitting a request we take a single global GEM wakeref, which trickles down to wake up all GT power domains. This is undesirable as we would like to be able to localise our power management to the available power domains and to remove the global GEM operations from the heart of the driver. (The intent there is to push global GEM decisions to the boundary as used by the GEM user interface.) Now during request construction, each request is responsible via its logical context to acquire a wakeref on each power domain it intends to utilize. Currently, each request takes a wakeref on the engine(s) and the engines themselves take a chipset wakeref. This gives us a transition on each engine which we can extend if we want to insert more powermangement control (such as soft rc6). The global GEM operations that currently require a struct_mutex are reduced to listening to pm events from the chipset GT wakeref. As we reduce the struct_mutex requirement, these listeners should evaporate. Perhaps the biggest immediate change is that this removes the struct_mutex requirement around GT power management, allowing us greater flexibility in request construction. Another important knock-on effect, is that by tracking engine usage, we can insert a switch back to the kernel context on that engine immediately, avoiding any extra delay or inserting global synchronisation barriers. This makes tracking when an engine and its associated contexts are idle much easier -- important for when we forgo our assumed execution ordering and need idle barriers to unpin used contexts. In the process, it means we remove a large chunk of code whose only purpose was to switch back to the kernel context. Signed-off-by: Chris Wilson <chris@chris-wilson.co.uk> Cc: Tvrtko Ursulin <tvrtko.ursulin@intel.com> Cc: Imre Deak <imre.deak@intel.com> Reviewed-by: Tvrtko Ursulin <tvrtko.ursulin@intel.com> Link: https://patchwork.freedesktop.org/patch/msgid/20190424200717.1686-5-chris@chris-wilson.co.uk
63 lines
2.5 KiB
C
63 lines
2.5 KiB
C
/*
|
|
* Copyright © 2014 Intel Corporation
|
|
*
|
|
* Permission is hereby granted, free of charge, to any person obtaining a
|
|
* copy of this software and associated documentation files (the "Software"),
|
|
* to deal in the Software without restriction, including without limitation
|
|
* the rights to use, copy, modify, merge, publish, distribute, sublicense,
|
|
* and/or sell copies of the Software, and to permit persons to whom the
|
|
* Software is furnished to do so, subject to the following conditions:
|
|
*
|
|
* The above copyright notice and this permission notice (including the next
|
|
* paragraph) shall be included in all copies or substantial portions of the
|
|
* Software.
|
|
*
|
|
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
|
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
|
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL
|
|
* THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
|
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
|
|
* FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS
|
|
* IN THE SOFTWARE.
|
|
*
|
|
*/
|
|
#ifndef _INTEL_UC_H_
|
|
#define _INTEL_UC_H_
|
|
|
|
#include "intel_guc.h"
|
|
#include "intel_huc.h"
|
|
#include "i915_params.h"
|
|
|
|
void intel_uc_init_early(struct drm_i915_private *dev_priv);
|
|
void intel_uc_cleanup_early(struct drm_i915_private *dev_priv);
|
|
void intel_uc_init_mmio(struct drm_i915_private *dev_priv);
|
|
int intel_uc_init_misc(struct drm_i915_private *dev_priv);
|
|
void intel_uc_fini_misc(struct drm_i915_private *dev_priv);
|
|
void intel_uc_sanitize(struct drm_i915_private *dev_priv);
|
|
int intel_uc_init_hw(struct drm_i915_private *dev_priv);
|
|
void intel_uc_fini_hw(struct drm_i915_private *dev_priv);
|
|
int intel_uc_init(struct drm_i915_private *dev_priv);
|
|
void intel_uc_fini(struct drm_i915_private *dev_priv);
|
|
void intel_uc_reset_prepare(struct drm_i915_private *i915);
|
|
void intel_uc_suspend(struct drm_i915_private *i915);
|
|
int intel_uc_resume(struct drm_i915_private *dev_priv);
|
|
|
|
static inline bool intel_uc_is_using_guc(struct drm_i915_private *i915)
|
|
{
|
|
GEM_BUG_ON(i915_modparams.enable_guc < 0);
|
|
return i915_modparams.enable_guc > 0;
|
|
}
|
|
|
|
static inline bool intel_uc_is_using_guc_submission(struct drm_i915_private *i915)
|
|
{
|
|
GEM_BUG_ON(i915_modparams.enable_guc < 0);
|
|
return i915_modparams.enable_guc & ENABLE_GUC_SUBMISSION;
|
|
}
|
|
|
|
static inline bool intel_uc_is_using_huc(struct drm_i915_private *i915)
|
|
{
|
|
GEM_BUG_ON(i915_modparams.enable_guc < 0);
|
|
return i915_modparams.enable_guc & ENABLE_GUC_LOAD_HUC;
|
|
}
|
|
|
|
#endif
|