mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-10-01 06:33:07 +00:00
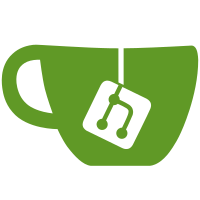
In some cases, nested locks may be needed, so {mtree,mas}_lock_nested is introduced. For example, when duplicating maple tree, we need to hold the locks of two trees, in which case nested locks are needed. At the same time, add the definition of spin_lock_nested() in tools for testing. Link: https://lkml.kernel.org/r/20231027033845.90608-3-zhangpeng.00@bytedance.com Signed-off-by: Peng Zhang <zhangpeng.00@bytedance.com> Reviewed-by: Liam R. Howlett <Liam.Howlett@oracle.com> Cc: Christian Brauner <brauner@kernel.org> Cc: Jonathan Corbet <corbet@lwn.net> Cc: Mateusz Guzik <mjguzik@gmail.com> Cc: Mathieu Desnoyers <mathieu.desnoyers@efficios.com> Cc: Matthew Wilcox <willy@infradead.org> Cc: Michael S. Tsirkin <mst@redhat.com> Cc: Mike Christie <michael.christie@oracle.com> Cc: Nicholas Piggin <npiggin@gmail.com> Cc: Peter Zijlstra <peterz@infradead.org> Cc: Suren Baghdasaryan <surenb@google.com> Signed-off-by: Andrew Morton <akpm@linux-foundation.org>
41 lines
1.2 KiB
C
41 lines
1.2 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef __LINUX_SPINLOCK_H_
|
|
#define __LINUX_SPINLOCK_H_
|
|
|
|
#include <pthread.h>
|
|
#include <stdbool.h>
|
|
|
|
#define spinlock_t pthread_mutex_t
|
|
#define DEFINE_SPINLOCK(x) pthread_mutex_t x = PTHREAD_MUTEX_INITIALIZER
|
|
#define __SPIN_LOCK_UNLOCKED(x) (pthread_mutex_t)PTHREAD_MUTEX_INITIALIZER
|
|
#define spin_lock_init(x) pthread_mutex_init(x, NULL)
|
|
|
|
#define spin_lock(x) pthread_mutex_lock(x)
|
|
#define spin_lock_nested(x, subclass) pthread_mutex_lock(x)
|
|
#define spin_unlock(x) pthread_mutex_unlock(x)
|
|
#define spin_lock_bh(x) pthread_mutex_lock(x)
|
|
#define spin_unlock_bh(x) pthread_mutex_unlock(x)
|
|
#define spin_lock_irq(x) pthread_mutex_lock(x)
|
|
#define spin_unlock_irq(x) pthread_mutex_unlock(x)
|
|
#define spin_lock_irqsave(x, f) (void)f, pthread_mutex_lock(x)
|
|
#define spin_unlock_irqrestore(x, f) (void)f, pthread_mutex_unlock(x)
|
|
|
|
#define arch_spinlock_t pthread_mutex_t
|
|
#define __ARCH_SPIN_LOCK_UNLOCKED PTHREAD_MUTEX_INITIALIZER
|
|
|
|
static inline void arch_spin_lock(arch_spinlock_t *mutex)
|
|
{
|
|
pthread_mutex_lock(mutex);
|
|
}
|
|
|
|
static inline void arch_spin_unlock(arch_spinlock_t *mutex)
|
|
{
|
|
pthread_mutex_unlock(mutex);
|
|
}
|
|
|
|
static inline bool arch_spin_is_locked(arch_spinlock_t *mutex)
|
|
{
|
|
return true;
|
|
}
|
|
|
|
#endif
|