mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-29 12:19:39 +00:00
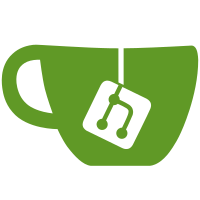
If an ipv4 packet (not locally generated with IP_DF flag not set) bigger than mtu size is supposed to go via a xfrm ipv6 tunnel, the packetsize check in xfrm4_tunnel_check_size() is omited and ipv6 drops the packet without sending a notice to the original sender of the ipv4 packet. Another issue is that ipv4 connection tracking does reassembling of incomming fragmented packets. If such a reassembled packet is supposed to go via a xfrm ipv6 tunnel it will be droped, even if the original sender did proper fragmentation. According to RFC 2473 (section 7) tunnel ipv6 packets resulting from the encapsulation of an original packet are considered as locally generated packets. If such a packet passed the checks in xfrm{4,6}_tunnel_check_size() fragmentation is allowed according to RFC 2473 (section 7.1/7.2). This patch sets skb->local_df in xfrm6_prepare_output() to achieve fragmentation in this case. Signed-off-by: Steffen Klassert <steffen.klassert@secunet.com> Signed-off-by: David S. Miller <davem@davemloft.net>
95 lines
2.1 KiB
C
95 lines
2.1 KiB
C
/*
|
|
* xfrm6_output.c - Common IPsec encapsulation code for IPv6.
|
|
* Copyright (C) 2002 USAGI/WIDE Project
|
|
* Copyright (c) 2004 Herbert Xu <herbert@gondor.apana.org.au>
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version
|
|
* 2 of the License, or (at your option) any later version.
|
|
*/
|
|
|
|
#include <linux/if_ether.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/module.h>
|
|
#include <linux/skbuff.h>
|
|
#include <linux/icmpv6.h>
|
|
#include <linux/netfilter_ipv6.h>
|
|
#include <net/dst.h>
|
|
#include <net/ipv6.h>
|
|
#include <net/xfrm.h>
|
|
|
|
int xfrm6_find_1stfragopt(struct xfrm_state *x, struct sk_buff *skb,
|
|
u8 **prevhdr)
|
|
{
|
|
return ip6_find_1stfragopt(skb, prevhdr);
|
|
}
|
|
|
|
EXPORT_SYMBOL(xfrm6_find_1stfragopt);
|
|
|
|
static int xfrm6_tunnel_check_size(struct sk_buff *skb)
|
|
{
|
|
int mtu, ret = 0;
|
|
struct dst_entry *dst = skb->dst;
|
|
|
|
mtu = dst_mtu(dst);
|
|
if (mtu < IPV6_MIN_MTU)
|
|
mtu = IPV6_MIN_MTU;
|
|
|
|
if (!skb->local_df && skb->len > mtu) {
|
|
skb->dev = dst->dev;
|
|
icmpv6_send(skb, ICMPV6_PKT_TOOBIG, 0, mtu, skb->dev);
|
|
ret = -EMSGSIZE;
|
|
}
|
|
|
|
return ret;
|
|
}
|
|
|
|
int xfrm6_extract_output(struct xfrm_state *x, struct sk_buff *skb)
|
|
{
|
|
int err;
|
|
|
|
err = xfrm6_tunnel_check_size(skb);
|
|
if (err)
|
|
return err;
|
|
|
|
XFRM_MODE_SKB_CB(skb)->protocol = ipv6_hdr(skb)->nexthdr;
|
|
|
|
return xfrm6_extract_header(skb);
|
|
}
|
|
|
|
int xfrm6_prepare_output(struct xfrm_state *x, struct sk_buff *skb)
|
|
{
|
|
int err;
|
|
|
|
err = xfrm_inner_extract_output(x, skb);
|
|
if (err)
|
|
return err;
|
|
|
|
memset(IP6CB(skb), 0, sizeof(*IP6CB(skb)));
|
|
#ifdef CONFIG_NETFILTER
|
|
IP6CB(skb)->flags |= IP6SKB_XFRM_TRANSFORMED;
|
|
#endif
|
|
|
|
skb->protocol = htons(ETH_P_IPV6);
|
|
skb->local_df = 1;
|
|
|
|
return x->outer_mode->output2(x, skb);
|
|
}
|
|
EXPORT_SYMBOL(xfrm6_prepare_output);
|
|
|
|
static int xfrm6_output_finish(struct sk_buff *skb)
|
|
{
|
|
#ifdef CONFIG_NETFILTER
|
|
IP6CB(skb)->flags |= IP6SKB_XFRM_TRANSFORMED;
|
|
#endif
|
|
|
|
skb->protocol = htons(ETH_P_IPV6);
|
|
return xfrm_output(skb);
|
|
}
|
|
|
|
int xfrm6_output(struct sk_buff *skb)
|
|
{
|
|
return NF_HOOK(PF_INET6, NF_INET_POST_ROUTING, skb, NULL, skb->dst->dev,
|
|
xfrm6_output_finish);
|
|
}
|