mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-26 02:39:48 +00:00
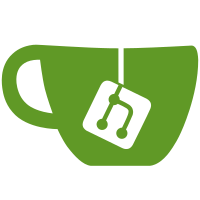
Add peci-cputemp driver for Digital Thermal Sensor (DTS) thermal readings of the processor package and processor cores that are accessible via the PECI interface. The main use case for the driver (and PECI interface) is out-of-band management, where we're able to obtain the DTS readings from an external entity connected with PECI, e.g. BMC on server platforms. Co-developed-by: Jae Hyun Yoo <jae.hyun.yoo@linux.intel.com> Reviewed-by: Pierre-Louis Bossart <pierre-louis.bossart@linux.intel.com> Acked-by: Guenter Roeck <linux@roeck-us.net> Acked-by: Joel Stanley <joel@jms.id.au> Signed-off-by: Jae Hyun Yoo <jae.hyun.yoo@linux.intel.com> Signed-off-by: Iwona Winiarska <iwona.winiarska@intel.com> Link: https://lore.kernel.org/r/20220208153639.255278-11-iwona.winiarska@intel.com Signed-off-by: Greg Kroah-Hartman <gregkh@linuxfoundation.org>
58 lines
1.4 KiB
C
58 lines
1.4 KiB
C
/* SPDX-License-Identifier: GPL-2.0-only */
|
|
/* Copyright (c) 2021 Intel Corporation */
|
|
|
|
#include <linux/mutex.h>
|
|
#include <linux/types.h>
|
|
|
|
#ifndef __PECI_HWMON_COMMON_H
|
|
#define __PECI_HWMON_COMMON_H
|
|
|
|
#define PECI_HWMON_UPDATE_INTERVAL HZ
|
|
|
|
/**
|
|
* struct peci_sensor_state - PECI state information
|
|
* @valid: flag to indicate the sensor value is valid
|
|
* @last_updated: time of the last update in jiffies
|
|
* @lock: mutex to protect sensor access
|
|
*/
|
|
struct peci_sensor_state {
|
|
bool valid;
|
|
unsigned long last_updated;
|
|
struct mutex lock; /* protect sensor access */
|
|
};
|
|
|
|
/**
|
|
* struct peci_sensor_data - PECI sensor information
|
|
* @value: sensor value in milli units
|
|
* @state: sensor update state
|
|
*/
|
|
|
|
struct peci_sensor_data {
|
|
s32 value;
|
|
struct peci_sensor_state state;
|
|
};
|
|
|
|
/**
|
|
* peci_sensor_need_update() - check whether sensor update is needed or not
|
|
* @sensor: pointer to sensor data struct
|
|
*
|
|
* Return: true if update is needed, false if not.
|
|
*/
|
|
|
|
static inline bool peci_sensor_need_update(struct peci_sensor_state *state)
|
|
{
|
|
return !state->valid ||
|
|
time_after(jiffies, state->last_updated + PECI_HWMON_UPDATE_INTERVAL);
|
|
}
|
|
|
|
/**
|
|
* peci_sensor_mark_updated() - mark the sensor is updated
|
|
* @sensor: pointer to sensor data struct
|
|
*/
|
|
static inline void peci_sensor_mark_updated(struct peci_sensor_state *state)
|
|
{
|
|
state->valid = true;
|
|
state->last_updated = jiffies;
|
|
}
|
|
|
|
#endif /* __PECI_HWMON_COMMON_H */
|