mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 08:58:07 +00:00
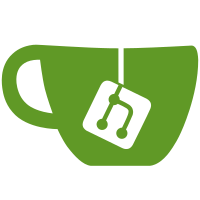
There is a regular need in the kernel to provide a way to declare having a dynamically sized set of trailing elements in a structure. Kernel code should always use “flexible array members”[1] for these cases. The older style of one-element or zero-length arrays should no longer be used[2]. This code was transformed with the help of Coccinelle: (next-20220214$ spatch --jobs $(getconf _NPROCESSORS_ONLN) --sp-file script.cocci --include-headers --dir . > output.patch) @@ identifier S, member, array; type T1, T2; @@ struct S { ... T1 member; T2 array[ - 0 ]; }; UAPI and wireless changes were intentionally excluded from this patch and will be sent out separately. [1] https://en.wikipedia.org/wiki/Flexible_array_member [2] https://www.kernel.org/doc/html/v5.16/process/deprecated.html#zero-length-and-one-element-arrays Link: https://github.com/KSPP/linux/issues/78 Reviewed-by: Kees Cook <keescook@chromium.org> Signed-off-by: Gustavo A. R. Silva <gustavoars@kernel.org>
68 lines
1.2 KiB
C
68 lines
1.2 KiB
C
/* SPDX-License-Identifier: GPL-2.0+ */
|
|
/*
|
|
* IPv6 IOAM implementation
|
|
*
|
|
* Author:
|
|
* Justin Iurman <justin.iurman@uliege.be>
|
|
*/
|
|
|
|
#ifndef _NET_IOAM6_H
|
|
#define _NET_IOAM6_H
|
|
|
|
#include <linux/net.h>
|
|
#include <linux/ipv6.h>
|
|
#include <linux/ioam6.h>
|
|
#include <linux/rhashtable-types.h>
|
|
|
|
struct ioam6_namespace {
|
|
struct rhash_head head;
|
|
struct rcu_head rcu;
|
|
|
|
struct ioam6_schema __rcu *schema;
|
|
|
|
__be16 id;
|
|
__be32 data;
|
|
__be64 data_wide;
|
|
};
|
|
|
|
struct ioam6_schema {
|
|
struct rhash_head head;
|
|
struct rcu_head rcu;
|
|
|
|
struct ioam6_namespace __rcu *ns;
|
|
|
|
u32 id;
|
|
int len;
|
|
__be32 hdr;
|
|
|
|
u8 data[];
|
|
};
|
|
|
|
struct ioam6_pernet_data {
|
|
struct mutex lock;
|
|
struct rhashtable namespaces;
|
|
struct rhashtable schemas;
|
|
};
|
|
|
|
static inline struct ioam6_pernet_data *ioam6_pernet(struct net *net)
|
|
{
|
|
#if IS_ENABLED(CONFIG_IPV6)
|
|
return net->ipv6.ioam6_data;
|
|
#else
|
|
return NULL;
|
|
#endif
|
|
}
|
|
|
|
struct ioam6_namespace *ioam6_namespace(struct net *net, __be16 id);
|
|
void ioam6_fill_trace_data(struct sk_buff *skb,
|
|
struct ioam6_namespace *ns,
|
|
struct ioam6_trace_hdr *trace,
|
|
bool is_input);
|
|
|
|
int ioam6_init(void);
|
|
void ioam6_exit(void);
|
|
|
|
int ioam6_iptunnel_init(void);
|
|
void ioam6_iptunnel_exit(void);
|
|
|
|
#endif /* _NET_IOAM6_H */
|