mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 08:58:07 +00:00
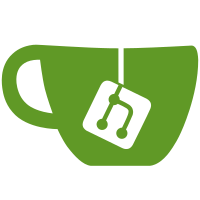
If the kernel oopses while on the trampoline stack, it will print "<SYSENTER>" even if SYSENTER is not involved. That is rather confusing. The "SYSENTER" stack is used for a lot more than SYSENTER now. Give it a better string to display in stack dumps, and rename the kernel code to match. Also move the 32-bit code over to the new naming even though it still uses the entry stack only for SYSENTER. Signed-off-by: Dave Hansen <dave.hansen@linux.intel.com> Signed-off-by: Thomas Gleixner <tglx@linutronix.de> Cc: Andy Lutomirski <luto@kernel.org> Cc: Borislav Petkov <bp@alien8.de> Cc: Borislav Petkov <bp@suse.de> Cc: Brian Gerst <brgerst@gmail.com> Cc: Denys Vlasenko <dvlasenk@redhat.com> Cc: H. Peter Anvin <hpa@zytor.com> Cc: Josh Poimboeuf <jpoimboe@redhat.com> Cc: Juergen Gross <jgross@suse.com> Cc: Linus Torvalds <torvalds@linux-foundation.org> Cc: Peter Zijlstra <peterz@infradead.org> Signed-off-by: Ingo Molnar <mingo@kernel.org>
116 lines
2.6 KiB
C
116 lines
2.6 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
/*
|
|
* Copyright (C) 1991, 1992 Linus Torvalds
|
|
* Copyright (C) 2000, 2001, 2002 Andi Kleen, SuSE Labs
|
|
*/
|
|
|
|
#ifndef _ASM_X86_STACKTRACE_H
|
|
#define _ASM_X86_STACKTRACE_H
|
|
|
|
#include <linux/uaccess.h>
|
|
#include <linux/ptrace.h>
|
|
#include <asm/switch_to.h>
|
|
|
|
enum stack_type {
|
|
STACK_TYPE_UNKNOWN,
|
|
STACK_TYPE_TASK,
|
|
STACK_TYPE_IRQ,
|
|
STACK_TYPE_SOFTIRQ,
|
|
STACK_TYPE_ENTRY,
|
|
STACK_TYPE_EXCEPTION,
|
|
STACK_TYPE_EXCEPTION_LAST = STACK_TYPE_EXCEPTION + N_EXCEPTION_STACKS-1,
|
|
};
|
|
|
|
struct stack_info {
|
|
enum stack_type type;
|
|
unsigned long *begin, *end, *next_sp;
|
|
};
|
|
|
|
bool in_task_stack(unsigned long *stack, struct task_struct *task,
|
|
struct stack_info *info);
|
|
|
|
bool in_entry_stack(unsigned long *stack, struct stack_info *info);
|
|
|
|
int get_stack_info(unsigned long *stack, struct task_struct *task,
|
|
struct stack_info *info, unsigned long *visit_mask);
|
|
|
|
const char *stack_type_name(enum stack_type type);
|
|
|
|
static inline bool on_stack(struct stack_info *info, void *addr, size_t len)
|
|
{
|
|
void *begin = info->begin;
|
|
void *end = info->end;
|
|
|
|
return (info->type != STACK_TYPE_UNKNOWN &&
|
|
addr >= begin && addr < end &&
|
|
addr + len > begin && addr + len <= end);
|
|
}
|
|
|
|
#ifdef CONFIG_X86_32
|
|
#define STACKSLOTS_PER_LINE 8
|
|
#else
|
|
#define STACKSLOTS_PER_LINE 4
|
|
#endif
|
|
|
|
#ifdef CONFIG_FRAME_POINTER
|
|
static inline unsigned long *
|
|
get_frame_pointer(struct task_struct *task, struct pt_regs *regs)
|
|
{
|
|
if (regs)
|
|
return (unsigned long *)regs->bp;
|
|
|
|
if (task == current)
|
|
return __builtin_frame_address(0);
|
|
|
|
return &((struct inactive_task_frame *)task->thread.sp)->bp;
|
|
}
|
|
#else
|
|
static inline unsigned long *
|
|
get_frame_pointer(struct task_struct *task, struct pt_regs *regs)
|
|
{
|
|
return NULL;
|
|
}
|
|
#endif /* CONFIG_FRAME_POINTER */
|
|
|
|
static inline unsigned long *
|
|
get_stack_pointer(struct task_struct *task, struct pt_regs *regs)
|
|
{
|
|
if (regs)
|
|
return (unsigned long *)kernel_stack_pointer(regs);
|
|
|
|
if (task == current)
|
|
return __builtin_frame_address(0);
|
|
|
|
return (unsigned long *)task->thread.sp;
|
|
}
|
|
|
|
void show_trace_log_lvl(struct task_struct *task, struct pt_regs *regs,
|
|
unsigned long *stack, char *log_lvl);
|
|
|
|
extern unsigned int code_bytes;
|
|
|
|
/* The form of the top of the frame on the stack */
|
|
struct stack_frame {
|
|
struct stack_frame *next_frame;
|
|
unsigned long return_address;
|
|
};
|
|
|
|
struct stack_frame_ia32 {
|
|
u32 next_frame;
|
|
u32 return_address;
|
|
};
|
|
|
|
static inline unsigned long caller_frame_pointer(void)
|
|
{
|
|
struct stack_frame *frame;
|
|
|
|
frame = __builtin_frame_address(0);
|
|
|
|
#ifdef CONFIG_FRAME_POINTER
|
|
frame = frame->next_frame;
|
|
#endif
|
|
|
|
return (unsigned long)frame;
|
|
}
|
|
|
|
#endif /* _ASM_X86_STACKTRACE_H */
|