mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-23 09:19:51 +00:00
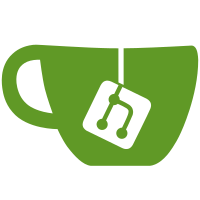
The UX360CA has a WMI device id 0x00060062, which reports whether the lid is flipped in tablet mode (1) or in normal laptop mode (0). Add a quirk (quirk_asus_use_lid_flip_devid) for devices on which this WMI device should be used to figure out the SW_TABLET_MODE state, as opposed to the quirk_asus_use_kbd_dock_devid. Additionally, the device needs to be queried on resume and restore because the firmware does not generate an event if the laptop is put to sleep while in tablet mode, flipped to normal mode, and later awoken. It is assumed other UX360* models have the same WMI device. As such, the quirk is applied to devices with DMI_MATCH(DMI_PRODUCT_NAME, "UX360"). More devices with this feature need to be tested and added accordingly. The reason for using an allowlist via the quirk mechanism is that the new WMI device (0x00060062) is also present on some models which do not have a 360 degree hinge (at least FX503VD and GL503VD from Hans' DSTS collection) and therefore its presence cannot be relied on. Signed-off-by: Samuel Čavoj <samuel@cavoj.net> Cc: Hans de Goede <hdegoede@redhat.com> Link: https://lore.kernel.org/r/20201020220944.1075530-1-samuel@cavoj.net Reviewed-by: Hans de Goede <hdegoede@redhat.com> Signed-off-by: Hans de Goede <hdegoede@redhat.com>
81 lines
2.2 KiB
C
81 lines
2.2 KiB
C
/* SPDX-License-Identifier: GPL-2.0-or-later */
|
|
/*
|
|
* Asus PC WMI hotkey driver
|
|
*
|
|
* Copyright(C) 2010 Intel Corporation.
|
|
* Copyright(C) 2010-2011 Corentin Chary <corentin.chary@gmail.com>
|
|
*
|
|
* Portions based on wistron_btns.c:
|
|
* Copyright (C) 2005 Miloslav Trmac <mitr@volny.cz>
|
|
* Copyright (C) 2005 Bernhard Rosenkraenzer <bero@arklinux.org>
|
|
* Copyright (C) 2005 Dmitry Torokhov <dtor@mail.ru>
|
|
*/
|
|
|
|
#ifndef _ASUS_WMI_H_
|
|
#define _ASUS_WMI_H_
|
|
|
|
#include <linux/platform_device.h>
|
|
#include <linux/i8042.h>
|
|
|
|
#define ASUS_WMI_KEY_IGNORE (-1)
|
|
#define ASUS_WMI_BRN_DOWN 0x20
|
|
#define ASUS_WMI_BRN_UP 0x2f
|
|
|
|
struct module;
|
|
struct key_entry;
|
|
struct asus_wmi;
|
|
|
|
struct quirk_entry {
|
|
bool hotplug_wireless;
|
|
bool scalar_panel_brightness;
|
|
bool store_backlight_power;
|
|
bool wmi_backlight_power;
|
|
bool wmi_backlight_native;
|
|
bool wmi_backlight_set_devstate;
|
|
bool wmi_force_als_set;
|
|
bool use_kbd_dock_devid;
|
|
bool use_lid_flip_devid;
|
|
int wapf;
|
|
/*
|
|
* For machines with AMD graphic chips, it will send out WMI event
|
|
* and ACPI interrupt at the same time while hitting the hotkey.
|
|
* To simplify the problem, we just have to ignore the WMI event,
|
|
* and let the ACPI interrupt to send out the key event.
|
|
*/
|
|
int no_display_toggle;
|
|
u32 xusb2pr;
|
|
|
|
bool (*i8042_filter)(unsigned char data, unsigned char str,
|
|
struct serio *serio);
|
|
};
|
|
|
|
struct asus_wmi_driver {
|
|
int brightness;
|
|
int panel_power;
|
|
int wlan_ctrl_by_user;
|
|
|
|
const char *name;
|
|
struct module *owner;
|
|
|
|
const char *event_guid;
|
|
|
|
const struct key_entry *keymap;
|
|
const char *input_name;
|
|
const char *input_phys;
|
|
struct quirk_entry *quirks;
|
|
/* Returns new code, value, and autorelease values in arguments.
|
|
* Return ASUS_WMI_KEY_IGNORE in code if event should be ignored. */
|
|
void (*key_filter) (struct asus_wmi_driver *driver, int *code,
|
|
unsigned int *value, bool *autorelease);
|
|
|
|
int (*probe) (struct platform_device *device);
|
|
void (*detect_quirks) (struct asus_wmi_driver *driver);
|
|
|
|
struct platform_driver platform_driver;
|
|
struct platform_device *platform_device;
|
|
};
|
|
|
|
int asus_wmi_register_driver(struct asus_wmi_driver *driver);
|
|
void asus_wmi_unregister_driver(struct asus_wmi_driver *driver);
|
|
|
|
#endif /* !_ASUS_WMI_H_ */
|