mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-12 13:55:32 +00:00
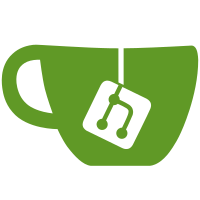
In order to increase the self-encapsulation of the dtpm generic code, the following changes are adding a power update ops to the dtpm ops. That allows the generic code to call directly the dtpm backend function to update the power values. The power update function does compute the power characteristics when the function is invoked. In the case of the CPUs, the power consumption depends on the number of online CPUs. The online CPUs mask is not up to date at CPUHP_AP_ONLINE_DYN state in the tear down callback. That is the reason why the online / offline are at separate state. As there is already an existing state for DTPM, this one is only moved to the DEAD state, so there is no addition of new state with these changes. The dtpm node is not removed when the cpu is unplugged. That simplifies the code for the next changes and results in a more self-encapsulated code. Signed-off-by: Daniel Lezcano <daniel.lezcano@linaro.org> Reviewed-by: Lukasz Luba <lukasz.luba@arm.com> Link: https://lore.kernel.org/r/20210312130411.29833-1-daniel.lezcano@linaro.org
78 lines
1.7 KiB
C
78 lines
1.7 KiB
C
/* SPDX-License-Identifier: GPL-2.0-only */
|
|
/*
|
|
* Copyright (C) 2020 Linaro Ltd
|
|
*
|
|
* Author: Daniel Lezcano <daniel.lezcano@linaro.org>
|
|
*/
|
|
#ifndef ___DTPM_H__
|
|
#define ___DTPM_H__
|
|
|
|
#include <linux/powercap.h>
|
|
|
|
#define MAX_DTPM_DESCR 8
|
|
#define MAX_DTPM_CONSTRAINTS 1
|
|
|
|
struct dtpm {
|
|
struct powercap_zone zone;
|
|
struct dtpm *parent;
|
|
struct list_head sibling;
|
|
struct list_head children;
|
|
struct dtpm_ops *ops;
|
|
unsigned long flags;
|
|
u64 power_limit;
|
|
u64 power_max;
|
|
u64 power_min;
|
|
int weight;
|
|
void *private;
|
|
};
|
|
|
|
struct dtpm_ops {
|
|
u64 (*set_power_uw)(struct dtpm *, u64);
|
|
u64 (*get_power_uw)(struct dtpm *);
|
|
int (*update_power_uw)(struct dtpm *);
|
|
void (*release)(struct dtpm *);
|
|
};
|
|
|
|
struct dtpm_descr;
|
|
|
|
typedef int (*dtpm_init_t)(struct dtpm_descr *);
|
|
|
|
struct dtpm_descr {
|
|
struct dtpm *parent;
|
|
const char *name;
|
|
dtpm_init_t init;
|
|
};
|
|
|
|
/* Init section thermal table */
|
|
extern struct dtpm_descr *__dtpm_table[];
|
|
extern struct dtpm_descr *__dtpm_table_end[];
|
|
|
|
#define DTPM_TABLE_ENTRY(name) \
|
|
static typeof(name) *__dtpm_table_entry_##name \
|
|
__used __section("__dtpm_table") = &name
|
|
|
|
#define DTPM_DECLARE(name) DTPM_TABLE_ENTRY(name)
|
|
|
|
#define for_each_dtpm_table(__dtpm) \
|
|
for (__dtpm = __dtpm_table; \
|
|
__dtpm < __dtpm_table_end; \
|
|
__dtpm++)
|
|
|
|
static inline struct dtpm *to_dtpm(struct powercap_zone *zone)
|
|
{
|
|
return container_of(zone, struct dtpm, zone);
|
|
}
|
|
|
|
int dtpm_update_power(struct dtpm *dtpm);
|
|
|
|
int dtpm_release_zone(struct powercap_zone *pcz);
|
|
|
|
struct dtpm *dtpm_alloc(struct dtpm_ops *ops);
|
|
|
|
void dtpm_unregister(struct dtpm *dtpm);
|
|
|
|
int dtpm_register(const char *name, struct dtpm *dtpm, struct dtpm *parent);
|
|
|
|
int dtpm_register_cpu(struct dtpm *parent);
|
|
|
|
#endif
|