mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-23 09:19:51 +00:00
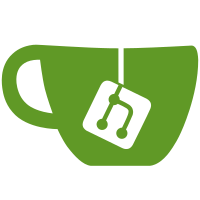
If userspace does not include the trailing end of batch message, then
nfnetlink aborts the transaction. This allows to check that ruleset
updates trigger no errors.
After this patch, invoking this command from the prerouting chain:
# nft -c add rule x y fib saddr . oif type local
fails since oif is not supported there.
This patch fixes the lack of rule validation from the abort/check path
to catch configuration errors such as the one above.
Fixes: a654de8fdc
("netfilter: nf_tables: fix chain dependency validation")
Signed-off-by: Pablo Neira Ayuso <pablo@netfilter.org>
74 lines
2.3 KiB
C
74 lines
2.3 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef _NFNETLINK_H
|
|
#define _NFNETLINK_H
|
|
|
|
#include <linux/netlink.h>
|
|
#include <linux/capability.h>
|
|
#include <net/netlink.h>
|
|
#include <uapi/linux/netfilter/nfnetlink.h>
|
|
|
|
struct nfnl_callback {
|
|
int (*call)(struct net *net, struct sock *nl, struct sk_buff *skb,
|
|
const struct nlmsghdr *nlh,
|
|
const struct nlattr * const cda[],
|
|
struct netlink_ext_ack *extack);
|
|
int (*call_rcu)(struct net *net, struct sock *nl, struct sk_buff *skb,
|
|
const struct nlmsghdr *nlh,
|
|
const struct nlattr * const cda[],
|
|
struct netlink_ext_ack *extack);
|
|
int (*call_batch)(struct net *net, struct sock *nl, struct sk_buff *skb,
|
|
const struct nlmsghdr *nlh,
|
|
const struct nlattr * const cda[],
|
|
struct netlink_ext_ack *extack);
|
|
const struct nla_policy *policy; /* netlink attribute policy */
|
|
const u_int16_t attr_count; /* number of nlattr's */
|
|
};
|
|
|
|
enum nfnl_abort_action {
|
|
NFNL_ABORT_NONE = 0,
|
|
NFNL_ABORT_AUTOLOAD,
|
|
NFNL_ABORT_VALIDATE,
|
|
};
|
|
|
|
struct nfnetlink_subsystem {
|
|
const char *name;
|
|
__u8 subsys_id; /* nfnetlink subsystem ID */
|
|
__u8 cb_count; /* number of callbacks */
|
|
const struct nfnl_callback *cb; /* callback for individual types */
|
|
struct module *owner;
|
|
int (*commit)(struct net *net, struct sk_buff *skb);
|
|
int (*abort)(struct net *net, struct sk_buff *skb,
|
|
enum nfnl_abort_action action);
|
|
void (*cleanup)(struct net *net);
|
|
bool (*valid_genid)(struct net *net, u32 genid);
|
|
};
|
|
|
|
int nfnetlink_subsys_register(const struct nfnetlink_subsystem *n);
|
|
int nfnetlink_subsys_unregister(const struct nfnetlink_subsystem *n);
|
|
|
|
int nfnetlink_has_listeners(struct net *net, unsigned int group);
|
|
int nfnetlink_send(struct sk_buff *skb, struct net *net, u32 portid,
|
|
unsigned int group, int echo, gfp_t flags);
|
|
int nfnetlink_set_err(struct net *net, u32 portid, u32 group, int error);
|
|
int nfnetlink_unicast(struct sk_buff *skb, struct net *net, u32 portid);
|
|
|
|
static inline u16 nfnl_msg_type(u8 subsys, u8 msg_type)
|
|
{
|
|
return subsys << 8 | msg_type;
|
|
}
|
|
|
|
void nfnl_lock(__u8 subsys_id);
|
|
void nfnl_unlock(__u8 subsys_id);
|
|
#ifdef CONFIG_PROVE_LOCKING
|
|
bool lockdep_nfnl_is_held(__u8 subsys_id);
|
|
#else
|
|
static inline bool lockdep_nfnl_is_held(__u8 subsys_id)
|
|
{
|
|
return true;
|
|
}
|
|
#endif /* CONFIG_PROVE_LOCKING */
|
|
|
|
#define MODULE_ALIAS_NFNL_SUBSYS(subsys) \
|
|
MODULE_ALIAS("nfnetlink-subsys-" __stringify(subsys))
|
|
|
|
#endif /* _NFNETLINK_H */
|