mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-14 06:35:12 +00:00
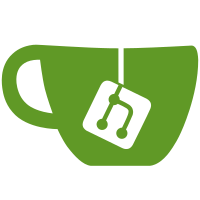
The taskstats interface uses microsecond granularity for the user and system time values. The conversion from cputime to the taskstats values uses the cputime_to_msecs primitive which effectively limits the granularity to milliseconds. Add the cputime_to_usecs primitive for architectures that have better, more precise CPU time values. Remove cputime_to_msecs primitive because there are no more users left. Signed-off-by: Michael Holzheu <holzheu@linux.vnet.ibm.com> Acked-by: Balbir Singh <balbir@linux.vnet.ibm.com> Cc: Luck Tony <tony.luck@intel.com> Cc: Shailabh Nagar <nagar1234@in.ibm.com> Cc: Martin Schwidefsky <schwidefsky@de.ibm.com> Cc: Oleg Nesterov <oleg@redhat.com> Cc: Benjamin Herrenschmidt <benh@kernel.crashing.org> Cc: Heiko Carstens <heiko.carstens@de.ibm.com> Cc: Thomas Gleixner <tglx@linutronix.de> Cc: Shailabh Nagar <nagar@us.ibm.com> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
70 lines
2 KiB
C
70 lines
2 KiB
C
#ifndef _ASM_GENERIC_CPUTIME_H
|
|
#define _ASM_GENERIC_CPUTIME_H
|
|
|
|
#include <linux/time.h>
|
|
#include <linux/jiffies.h>
|
|
|
|
typedef unsigned long cputime_t;
|
|
|
|
#define cputime_zero (0UL)
|
|
#define cputime_one_jiffy jiffies_to_cputime(1)
|
|
#define cputime_max ((~0UL >> 1) - 1)
|
|
#define cputime_add(__a, __b) ((__a) + (__b))
|
|
#define cputime_sub(__a, __b) ((__a) - (__b))
|
|
#define cputime_div(__a, __n) ((__a) / (__n))
|
|
#define cputime_halve(__a) ((__a) >> 1)
|
|
#define cputime_eq(__a, __b) ((__a) == (__b))
|
|
#define cputime_gt(__a, __b) ((__a) > (__b))
|
|
#define cputime_ge(__a, __b) ((__a) >= (__b))
|
|
#define cputime_lt(__a, __b) ((__a) < (__b))
|
|
#define cputime_le(__a, __b) ((__a) <= (__b))
|
|
#define cputime_to_jiffies(__ct) (__ct)
|
|
#define cputime_to_scaled(__ct) (__ct)
|
|
#define jiffies_to_cputime(__hz) (__hz)
|
|
|
|
typedef u64 cputime64_t;
|
|
|
|
#define cputime64_zero (0ULL)
|
|
#define cputime64_add(__a, __b) ((__a) + (__b))
|
|
#define cputime64_sub(__a, __b) ((__a) - (__b))
|
|
#define cputime64_to_jiffies64(__ct) (__ct)
|
|
#define jiffies64_to_cputime64(__jif) (__jif)
|
|
#define cputime_to_cputime64(__ct) ((u64) __ct)
|
|
|
|
|
|
/*
|
|
* Convert cputime to microseconds and back.
|
|
*/
|
|
#define cputime_to_usecs(__ct) jiffies_to_usecs(__ct);
|
|
#define usecs_to_cputime(__msecs) usecs_to_jiffies(__msecs);
|
|
|
|
/*
|
|
* Convert cputime to seconds and back.
|
|
*/
|
|
#define cputime_to_secs(jif) ((jif) / HZ)
|
|
#define secs_to_cputime(sec) ((sec) * HZ)
|
|
|
|
/*
|
|
* Convert cputime to timespec and back.
|
|
*/
|
|
#define timespec_to_cputime(__val) timespec_to_jiffies(__val)
|
|
#define cputime_to_timespec(__ct,__val) jiffies_to_timespec(__ct,__val)
|
|
|
|
/*
|
|
* Convert cputime to timeval and back.
|
|
*/
|
|
#define timeval_to_cputime(__val) timeval_to_jiffies(__val)
|
|
#define cputime_to_timeval(__ct,__val) jiffies_to_timeval(__ct,__val)
|
|
|
|
/*
|
|
* Convert cputime to clock and back.
|
|
*/
|
|
#define cputime_to_clock_t(__ct) jiffies_to_clock_t(__ct)
|
|
#define clock_t_to_cputime(__x) clock_t_to_jiffies(__x)
|
|
|
|
/*
|
|
* Convert cputime64 to clock.
|
|
*/
|
|
#define cputime64_to_clock_t(__ct) jiffies_64_to_clock_t(__ct)
|
|
|
|
#endif
|