mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-26 19:00:25 +00:00
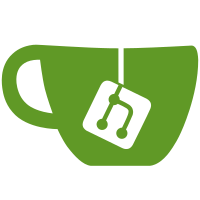
After installing a route to the kernel, user space receives an acknowledgment, which means the route was installed in the kernel, but not necessarily in hardware. The asynchronous nature of route installation in hardware can lead to a routing daemon advertising a route before it was actually installed in hardware. This can result in packet loss or mis-routed packets until the route is installed in hardware. To avoid such cases, previous patch set added the ability to emit RTM_NEWROUTE notifications whenever RTM_F_OFFLOAD/RTM_F_TRAP flags are changed, this behavior is controlled by sysctl. With the above mentioned behavior, it is possible to know from user-space if the route was offloaded, but if the offload fails there is no indication to user-space. Following a failure, a routing daemon will wait indefinitely for a notification that will never come. This patch adds an "offload_failed" indication to IPv4 routes, so that users will have better visibility into the offload process. 'struct fib_alias', and 'struct fib_rt_info' are extended with new field that indicates if route offload failed. Note that the new field is added using unused bit and therefore there is no need to increase structs size. Signed-off-by: Amit Cohen <amcohen@nvidia.com> Signed-off-by: Ido Schimmel <idosch@nvidia.com> Signed-off-by: David S. Miller <davem@davemloft.net>
63 lines
1.6 KiB
C
63 lines
1.6 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef _FIB_LOOKUP_H
|
|
#define _FIB_LOOKUP_H
|
|
|
|
#include <linux/types.h>
|
|
#include <linux/list.h>
|
|
#include <net/ip_fib.h>
|
|
#include <net/nexthop.h>
|
|
|
|
struct fib_alias {
|
|
struct hlist_node fa_list;
|
|
struct fib_info *fa_info;
|
|
u8 fa_tos;
|
|
u8 fa_type;
|
|
u8 fa_state;
|
|
u8 fa_slen;
|
|
u32 tb_id;
|
|
s16 fa_default;
|
|
u8 offload:1,
|
|
trap:1,
|
|
offload_failed:1,
|
|
unused:5;
|
|
struct rcu_head rcu;
|
|
};
|
|
|
|
#define FA_S_ACCESSED 0x01
|
|
|
|
/* Dont write on fa_state unless needed, to keep it shared on all cpus */
|
|
static inline void fib_alias_accessed(struct fib_alias *fa)
|
|
{
|
|
if (!(fa->fa_state & FA_S_ACCESSED))
|
|
fa->fa_state |= FA_S_ACCESSED;
|
|
}
|
|
|
|
/* Exported by fib_semantics.c */
|
|
void fib_release_info(struct fib_info *);
|
|
struct fib_info *fib_create_info(struct fib_config *cfg,
|
|
struct netlink_ext_ack *extack);
|
|
int fib_nh_match(struct net *net, struct fib_config *cfg, struct fib_info *fi,
|
|
struct netlink_ext_ack *extack);
|
|
bool fib_metrics_match(struct fib_config *cfg, struct fib_info *fi);
|
|
int fib_dump_info(struct sk_buff *skb, u32 pid, u32 seq, int event,
|
|
const struct fib_rt_info *fri, unsigned int flags);
|
|
void rtmsg_fib(int event, __be32 key, struct fib_alias *fa, int dst_len,
|
|
u32 tb_id, const struct nl_info *info, unsigned int nlm_flags);
|
|
size_t fib_nlmsg_size(struct fib_info *fi);
|
|
|
|
static inline void fib_result_assign(struct fib_result *res,
|
|
struct fib_info *fi)
|
|
{
|
|
/* we used to play games with refcounts, but we now use RCU */
|
|
res->fi = fi;
|
|
res->nhc = fib_info_nhc(fi, 0);
|
|
}
|
|
|
|
struct fib_prop {
|
|
int error;
|
|
u8 scope;
|
|
};
|
|
|
|
extern const struct fib_prop fib_props[RTN_MAX + 1];
|
|
|
|
#endif /* _FIB_LOOKUP_H */
|