mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 08:58:07 +00:00
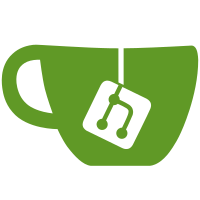
-----BEGIN PGP SIGNATURE----- iHUEABYIAB0WIQQUwxxKyE5l/npt8ARiEGxRG/Sl2wUCXRozKAAKCRBiEGxRG/Sl 25okAP9I0Rmscpqjb/+GEeXH4EmL3moGzc9o/BzHRqfeO4wqYAEA+8f7L20xHe8g tvEGfP7mN/oBmcAfqgH5K9F4eJsBRAw= =NkCn -----END PGP SIGNATURE----- Merge tag 'leds-for-5.3-rc1' of git://git.kernel.org/pub/scm/linux/kernel/git/j.anaszewski/linux-leds Pull LED updates from Jacek Anaszewski: - Add a new LED common module for ti-lmu driver family - Modify MFD ti-lmu bindings - add ti,brightness-resolution - add the ramp up/down property - Add regulator support for LM36274 driver to lm363x-regulator.c - New LED class drivers with DT bindings: - leds-spi-byte - leds-lm36274 - leds-lm3697 (move the support from MFD to LED subsystem) - Simplify getting the I2C adapter of a client: - leds-tca6507 - leds-pca955x - Convert LED documentation to ReST * tag 'leds-for-5.3-rc1' of git://git.kernel.org/pub/scm/linux/kernel/git/j.anaszewski/linux-leds: dt: leds-lm36274.txt: fix a broken reference to ti-lmu.txt docs: leds: convert to ReST leds: leds-tca6507: simplify getting the adapter of a client leds: leds-pca955x: simplify getting the adapter of a client leds: lm36274: Introduce the TI LM36274 LED driver dt-bindings: leds: Add LED bindings for the LM36274 regulator: lm363x: Add support for LM36274 mfd: ti-lmu: Add LM36274 support to the ti-lmu dt-bindings: mfd: Add lm36274 bindings to ti-lmu leds: max77650: Remove set but not used variable 'parent' leds: avoid flush_work in atomic context leds: lm3697: Introduce the lm3697 driver mfd: ti-lmu: Remove support for LM3697 dt-bindings: ti-lmu: Modify dt bindings for the LM3697 leds: TI LMU: Add common code for TI LMU devices leds: spi-byte: add single byte SPI LED driver dt-bindings: leds: Add binding for spi-byte LED. dt-bindings: mfd: LMU: Add ti,brightness-resolution dt-bindings: mfd: LMU: Add the ramp up/down property
87 lines
1.8 KiB
C
87 lines
1.8 KiB
C
/* SPDX-License-Identifier: GPL-2.0-only */
|
|
/*
|
|
* TI LMU (Lighting Management Unit) Devices
|
|
*
|
|
* Copyright 2017 Texas Instruments
|
|
*
|
|
* Author: Milo Kim <milo.kim@ti.com>
|
|
*/
|
|
|
|
#ifndef __MFD_TI_LMU_H__
|
|
#define __MFD_TI_LMU_H__
|
|
|
|
#include <linux/gpio.h>
|
|
#include <linux/notifier.h>
|
|
#include <linux/regmap.h>
|
|
#include <linux/gpio/consumer.h>
|
|
|
|
/* Notifier event */
|
|
#define LMU_EVENT_MONITOR_DONE 0x01
|
|
|
|
enum ti_lmu_id {
|
|
LM3631,
|
|
LM3632,
|
|
LM3633,
|
|
LM3695,
|
|
LM36274,
|
|
LMU_MAX_ID,
|
|
};
|
|
|
|
enum ti_lmu_max_current {
|
|
LMU_IMAX_5mA,
|
|
LMU_IMAX_6mA,
|
|
LMU_IMAX_7mA = 0x03,
|
|
LMU_IMAX_8mA,
|
|
LMU_IMAX_9mA,
|
|
LMU_IMAX_10mA = 0x07,
|
|
LMU_IMAX_11mA,
|
|
LMU_IMAX_12mA,
|
|
LMU_IMAX_13mA,
|
|
LMU_IMAX_14mA,
|
|
LMU_IMAX_15mA = 0x0D,
|
|
LMU_IMAX_16mA,
|
|
LMU_IMAX_17mA,
|
|
LMU_IMAX_18mA,
|
|
LMU_IMAX_19mA,
|
|
LMU_IMAX_20mA = 0x13,
|
|
LMU_IMAX_21mA,
|
|
LMU_IMAX_22mA,
|
|
LMU_IMAX_23mA = 0x17,
|
|
LMU_IMAX_24mA,
|
|
LMU_IMAX_25mA,
|
|
LMU_IMAX_26mA,
|
|
LMU_IMAX_27mA = 0x1C,
|
|
LMU_IMAX_28mA,
|
|
LMU_IMAX_29mA,
|
|
LMU_IMAX_30mA,
|
|
};
|
|
|
|
enum lm363x_regulator_id {
|
|
LM3631_BOOST, /* Boost output */
|
|
LM3631_LDO_CONT, /* Display panel controller */
|
|
LM3631_LDO_OREF, /* Gamma reference */
|
|
LM3631_LDO_POS, /* Positive display bias output */
|
|
LM3631_LDO_NEG, /* Negative display bias output */
|
|
LM3632_BOOST, /* Boost output */
|
|
LM3632_LDO_POS, /* Positive display bias output */
|
|
LM3632_LDO_NEG, /* Negative display bias output */
|
|
LM36274_BOOST, /* Boost output */
|
|
LM36274_LDO_POS, /* Positive display bias output */
|
|
LM36274_LDO_NEG, /* Negative display bias output */
|
|
};
|
|
|
|
/**
|
|
* struct ti_lmu
|
|
*
|
|
* @dev: Parent device pointer
|
|
* @regmap: Used for i2c communcation on accessing registers
|
|
* @en_gpio: GPIO for HWEN pin [Optional]
|
|
* @notifier: Notifier for reporting hwmon event
|
|
*/
|
|
struct ti_lmu {
|
|
struct device *dev;
|
|
struct regmap *regmap;
|
|
struct gpio_desc *en_gpio;
|
|
struct blocking_notifier_head notifier;
|
|
};
|
|
#endif
|