mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-24 17:59:32 +00:00
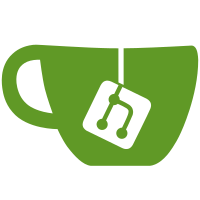
Pull drm updates from Dave Airlie:
"I Was Almost Tempted To Capitalise Every Word, but then I decided I
couldn't read it myself!
I've also got one pull request for the sti driver outstanding. It
relied on a commit in Greg's tree and I didn't find out in time, that
commit is in your tree now so I might send that along once this is
merged.
I also had the accidental misfortune to have access to a Skylake on my
desk for a few days, and I've had to encourage Intel to try harder,
which seems to be happening now.
Here is the main drm-next pull request for 4.4.
Highlights:
New driver:
vc4 driver for the Rasberry Pi VPU.
(From Eric Anholt at Broadcom.)
Core:
Atomic fbdev support
Atomic helpers for runtime pm
dp/aux i2c STATUS_UPDATE handling
struct_mutex usage cleanups.
Generic of probing support.
Documentation:
Kerneldoc for VGA switcheroo code.
Rename to gpu instead of drm to reflect scope.
i915:
Skylake GuC firmware fixes
HPD A support
VBT backlight fallbacks
Fastboot by default for some systems
FBC work
BXT/SKL workarounds
Skylake deeper sleep state fixes
amdgpu:
Enable GPU scheduler by default
New atombios opcodes
GPUVM debugging options
Stoney support.
Fencing cleanups.
radeon:
More efficient CS checking
nouveau:
gk20a instance memory handling improvements.
Improved PGOB detection and GK107 support
Kepler GDDR5 PLL statbility improvement
G8x/GT2xx reclock improvements
new userspace API compatiblity fixes.
virtio-gpu:
Add 3D support - qemu 2.5 has it merged for it's gtk backend.
msm:
Initial msm88896 (snapdragon 8200)
exynos:
HDMI cleanups
Enable mixer driver byt default
Add DECON-TV support
vmwgfx:
Move to using memremap + fixes.
rcar-du:
Add support for R8A7793/4 DU
armada:
Remove support for non-component mode
Improved plane handling
Power savings while in DPMS off.
tda998x:
Remove unused slave encoder support
Use more HDMI helpers
Fix EDID read handling
dwhdmi:
Interlace video mode support for ipu-v3/dw_hdmi
Hotplug state fixes
Audio driver integration
imx:
More color formats support.
tegra:
Minor fixes/improvements"
[ Merge fixup: remove unused variable 'dev' that had all uses removed in
commit 4e270f0880
: "drm/gem: Drop struct_mutex requirement from
drm_gem_mmap_obj" ]
* 'drm-next' of git://people.freedesktop.org/~airlied/linux: (764 commits)
drm/vmwgfx: Relax irq locking somewhat
drm/vmwgfx: Properly flush cursor updates and page-flips
drm/i915/skl: disable display side power well support for now
drm/i915: Extend DSL readout fix to BDW and SKL.
drm/i915: Do graphics device reset under forcewake
drm/i915: Skip fence installation for objects with rotated views (v4)
vga_switcheroo: Drop client power state VGA_SWITCHEROO_INIT
drm/amdgpu: group together common fence implementation
drm/amdgpu: remove AMDGPU_FENCE_OWNER_MOVE
drm/amdgpu: remove now unused fence functions
drm/amdgpu: fix fence fallback check
drm/amdgpu: fix stoping the scheduler timeout
drm/amdgpu: cleanup on error in amdgpu_cs_ioctl()
drm/i915: Fix locking around GuC firmware load
drm/amdgpu: update Fiji's Golden setting
drm/amdgpu: update Fiji's rev id
drm/amdgpu: extract common code in vi_common_early_init
drm/amd/scheduler: don't oops on failure to load
drm/amdgpu: don't oops on failure to load (v2)
drm/amdgpu: don't VT switch on suspend
...
119 lines
3.7 KiB
C
119 lines
3.7 KiB
C
/*
|
|
* Copyright (C) 2015 Red Hat, Inc.
|
|
* All Rights Reserved.
|
|
*
|
|
* Permission is hereby granted, free of charge, to any person obtaining
|
|
* a copy of this software and associated documentation files (the
|
|
* "Software"), to deal in the Software without restriction, including
|
|
* without limitation the rights to use, copy, modify, merge, publish,
|
|
* distribute, sublicense, and/or sell copies of the Software, and to
|
|
* permit persons to whom the Software is furnished to do so, subject to
|
|
* the following conditions:
|
|
*
|
|
* The above copyright notice and this permission notice (including the
|
|
* next paragraph) shall be included in all copies or substantial
|
|
* portions of the Software.
|
|
*
|
|
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
|
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
|
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
|
|
* IN NO EVENT SHALL THE COPYRIGHT OWNER(S) AND/OR ITS SUPPLIERS BE
|
|
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
|
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
|
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
|
*/
|
|
|
|
#include <drm/drmP.h>
|
|
#include "virtgpu_drv.h"
|
|
|
|
static const char *virtio_get_driver_name(struct fence *f)
|
|
{
|
|
return "virtio_gpu";
|
|
}
|
|
|
|
static const char *virtio_get_timeline_name(struct fence *f)
|
|
{
|
|
return "controlq";
|
|
}
|
|
|
|
static bool virtio_enable_signaling(struct fence *f)
|
|
{
|
|
return true;
|
|
}
|
|
|
|
static bool virtio_signaled(struct fence *f)
|
|
{
|
|
struct virtio_gpu_fence *fence = to_virtio_fence(f);
|
|
|
|
if (atomic64_read(&fence->drv->last_seq) >= fence->seq)
|
|
return true;
|
|
return false;
|
|
}
|
|
|
|
static void virtio_fence_value_str(struct fence *f, char *str, int size)
|
|
{
|
|
struct virtio_gpu_fence *fence = to_virtio_fence(f);
|
|
|
|
snprintf(str, size, "%llu", fence->seq);
|
|
}
|
|
|
|
static void virtio_timeline_value_str(struct fence *f, char *str, int size)
|
|
{
|
|
struct virtio_gpu_fence *fence = to_virtio_fence(f);
|
|
|
|
snprintf(str, size, "%llu", (u64)atomic64_read(&fence->drv->last_seq));
|
|
}
|
|
|
|
static const struct fence_ops virtio_fence_ops = {
|
|
.get_driver_name = virtio_get_driver_name,
|
|
.get_timeline_name = virtio_get_timeline_name,
|
|
.enable_signaling = virtio_enable_signaling,
|
|
.signaled = virtio_signaled,
|
|
.wait = fence_default_wait,
|
|
.fence_value_str = virtio_fence_value_str,
|
|
.timeline_value_str = virtio_timeline_value_str,
|
|
};
|
|
|
|
int virtio_gpu_fence_emit(struct virtio_gpu_device *vgdev,
|
|
struct virtio_gpu_ctrl_hdr *cmd_hdr,
|
|
struct virtio_gpu_fence **fence)
|
|
{
|
|
struct virtio_gpu_fence_driver *drv = &vgdev->fence_drv;
|
|
unsigned long irq_flags;
|
|
|
|
*fence = kmalloc(sizeof(struct virtio_gpu_fence), GFP_ATOMIC);
|
|
if ((*fence) == NULL)
|
|
return -ENOMEM;
|
|
|
|
spin_lock_irqsave(&drv->lock, irq_flags);
|
|
(*fence)->drv = drv;
|
|
(*fence)->seq = ++drv->sync_seq;
|
|
fence_init(&(*fence)->f, &virtio_fence_ops, &drv->lock,
|
|
0, (*fence)->seq);
|
|
fence_get(&(*fence)->f);
|
|
list_add_tail(&(*fence)->node, &drv->fences);
|
|
spin_unlock_irqrestore(&drv->lock, irq_flags);
|
|
|
|
cmd_hdr->flags |= cpu_to_le32(VIRTIO_GPU_FLAG_FENCE);
|
|
cmd_hdr->fence_id = cpu_to_le64((*fence)->seq);
|
|
return 0;
|
|
}
|
|
|
|
void virtio_gpu_fence_event_process(struct virtio_gpu_device *vgdev,
|
|
u64 last_seq)
|
|
{
|
|
struct virtio_gpu_fence_driver *drv = &vgdev->fence_drv;
|
|
struct virtio_gpu_fence *fence, *tmp;
|
|
unsigned long irq_flags;
|
|
|
|
spin_lock_irqsave(&drv->lock, irq_flags);
|
|
atomic64_set(&vgdev->fence_drv.last_seq, last_seq);
|
|
list_for_each_entry_safe(fence, tmp, &drv->fences, node) {
|
|
if (last_seq < fence->seq)
|
|
continue;
|
|
fence_signal_locked(&fence->f);
|
|
list_del(&fence->node);
|
|
fence_put(&fence->f);
|
|
}
|
|
spin_unlock_irqrestore(&drv->lock, irq_flags);
|
|
}
|